Optimizing Memory Usage in Java Applications
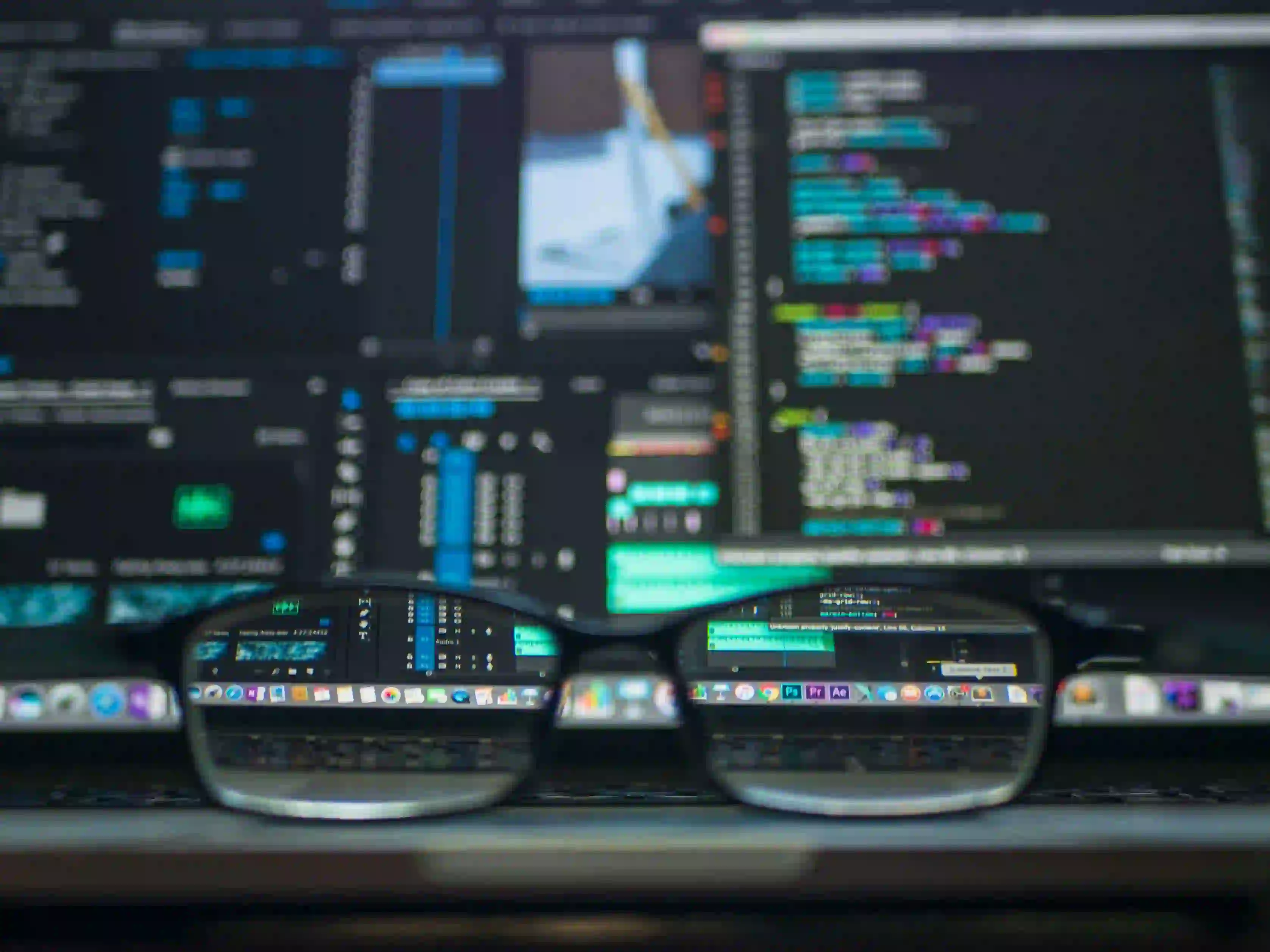
Optimizing Memory Usage in Java Applications
Memory management is a critical aspect of Java application development. Inefficient memory allocation and management can lead to increased resource consumption, degraded performance, and even application crashes. Therefore, optimizing memory usage in Java applications is essential for achieving better performance and scalability.
In this article, we will explore various techniques and best practices for optimizing memory usage in Java applications.
1. Use Primitive Data Types
When dealing with simple data types like integers, booleans, or characters, it's better to use primitive data types (int
, boolean
, char
, etc.) instead of their object counterparts (Integer
, Boolean
, Character
, etc.). Primitive types consume less memory as they directly hold the value, whereas object types carry additional metadata.
Example:
// Object type
Integer value = new Integer(10);
// Primitive type
int primitiveValue = 10;
In this example, the Integer
object consumes more memory compared to the int
primitive type.
2. Efficient Collections Usage
When working with collections such as lists, sets, and maps, it's important to choose the right collection type based on the specific requirements. For example, if the size of the collection is known and fixed, it's better to use arrays instead of ArrayLists to save memory.
Example:
// ArrayList
List<String> list = new ArrayList<>();
// Array
String[] array = new String[10];
In this case, using an array saves memory compared to an ArrayList because it doesn't need to store extra information about capacity and resizing.
3. Avoid Memory Leaks
Memory leaks can occur if objects are not properly managed and released when they are no longer needed. It's important to be mindful of object references and ensure that they are properly nullified when no longer in use. Failing to do so can lead to memory leaks and unnecessary memory consumption.
4. Use String Pool for String Literals
In Java, string literals (e.g., "hello"
) are stored in a string pool, which allows multiple references to the same string value to share a single copy. This can significantly reduce memory usage when working with a large number of string literals.
Example:
String s1 = "hello";
String s2 = "hello";
// s1 and s2 refer to the same "hello" instance in the string pool
5. Limit Object Instantiation
Frequent object instantiation can lead to increased memory usage and overhead. Reusing objects instead of creating new ones can help in optimizing memory consumption.
Example:
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("some text");
}
String result = sb.toString();
sb.setLength(0); // Reusing the same StringBuilder instance
6. Use Memory Profiling Tools
Utilize memory profiling tools such as VisualVM, YourKit, or Java Mission Control to analyze memory usage, identify potential memory leaks, and optimize memory allocation in Java applications.
7. Implement Object Pooling
Object pooling can be implemented for frequently used objects to avoid the overhead of creating and garbage collecting objects. This can be particularly beneficial for heavy-weight objects or resources.
8. Optimize Garbage Collection
Tune garbage collection settings based on the specific memory requirements and usage patterns of the application. This can involve adjusting the heap size, garbage collection algorithms, and other related parameters.
Final Thoughts
Optimizing memory usage in Java applications is crucial for achieving better performance and scalability. By following the best practices outlined in this article, developers can effectively manage memory consumption, reduce overhead, and mitigate potential issues such as memory leaks and inefficient garbage collection.
Remember to strike a balance between memory optimization and code readability/maintainability. As with any optimization effort, it's important to profile and measure the impact of changes to ensure that they result in tangible improvements without sacrificing code clarity.
In summary, efficient memory management is not just about saving memory, but also about improving the overall performance and user experience of Java applications.
By incorporating these techniques and best practices, developers can enhance the efficiency and reliability of their Java applications while ensuring optimal memory usage.
Remember, always profile your application to measure the impact of the memory optimization techniques and adjust accordingly.
Happy coding!