The Pitfalls of Not Using Connection Pooling
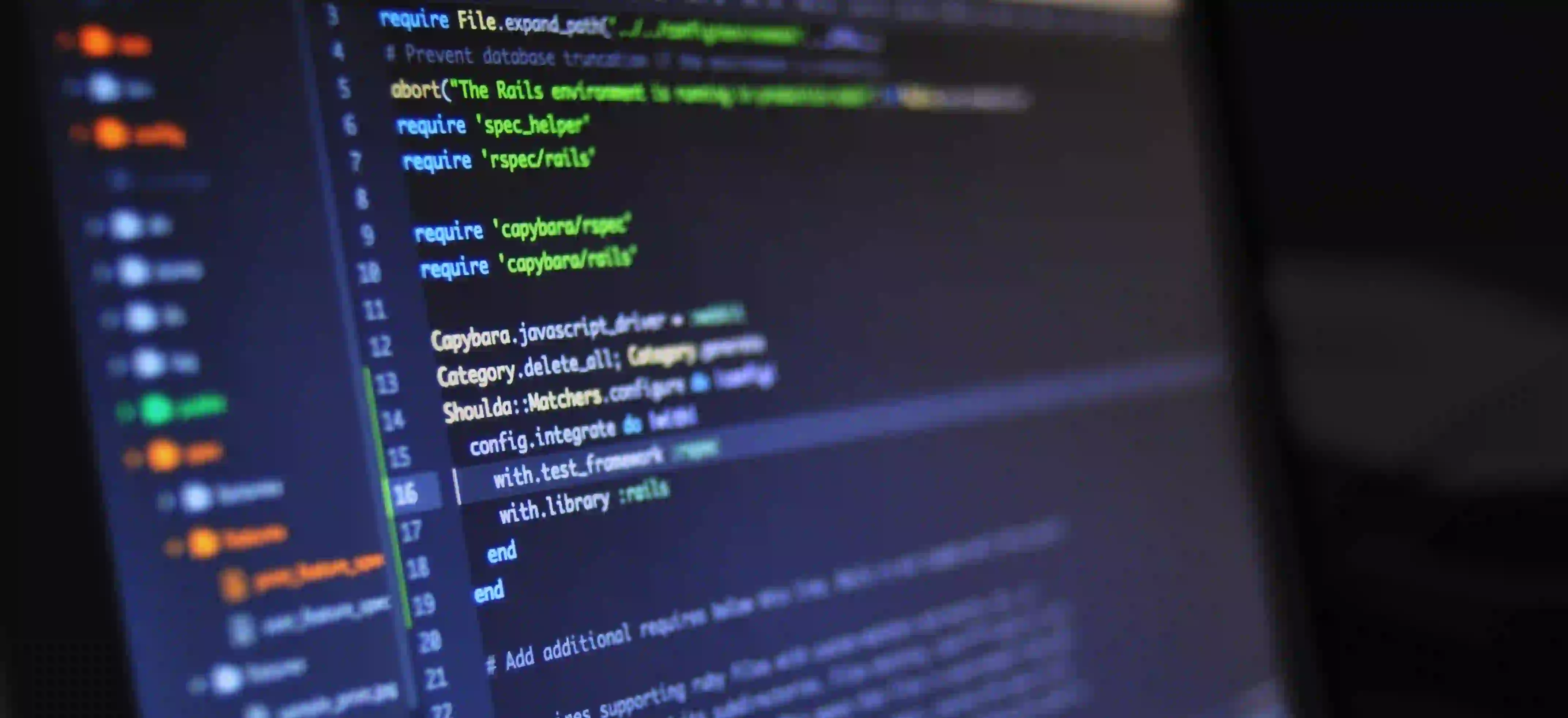
The Pitfalls of Not Using Connection Pooling in Java
In the realm of Java development, effective management of database connections is crucial for the performance and scalability of applications. One fundamental practice for achieving efficient connection management is through the use of connection pooling. In this article, we will delve into the potential pitfalls of not employing connection pooling in Java applications, and how embracing this approach can lead to substantial benefits.
Understanding Connection Pooling
Before we delve into the perils of avoiding connection pooling, it is imperative to grasp the concept itself. Connection pooling involves creating a pool of database connections that can be reused, rather than creating a new connection for each request to the database. This mechanism significantly reduces the overhead of creating and destroying connections, leading to improved performance and resource utilization.
The Perils of Neglecting Connection Pooling
Performance Bottlenecks
When connection pooling is omitted, the process of establishing and tearing down a connection for each database interaction can be a major performance bottleneck. The overhead incurred in creating new connections, authentication, and network setup can significantly degrade the responsiveness of the application.
Code Example: Creating a New Connection without Connection Pooling
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
// Perform database operations
connection.close(); // Connection closed for each operation
The above code snippet demonstrates the precarious practice of creating a new connection for each database operation, potentially leading to performance issues.
Resource Exhaustion
In scenarios where connection pooling is absent, the application may exhaust the available database connections under heavy load. Without a limit on the number of connections being created, the database server can become overwhelmed, leading to resource contention and potentially crashing the application.
Scalability Impediments
In the absence of connection pooling, scaling the application to accommodate increased load becomes arduous. The lack of efficient connection management can hinder the ability to handle a growing number of concurrent users, limiting the application's scalability.
Memory Leaks
Failing to use connection pooling can also result in memory leaks. Improper handling of connections, such as not closing them after use, can lead to resource leakage and eventual depletion of system resources.
Embracing Connection Pooling in Java
Benefits of Connection Pooling
By incorporating connection pooling into Java applications, the aforementioned perils can be averted, paving the way for enhanced performance, scalability, and resource efficiency. Connection pooling offers the following benefits:
-
Improved Performance: Reusing established connections reduces the overhead of creating new connections, resulting in improved response times and overall application performance.
-
Resource Optimization: Connection pooling ensures efficient utilization of database resources by reusing connections, thus preventing resource exhaustion and contention.
-
Scalability: With connection pooling, applications are better equipped to handle increased concurrent requests, facilitating scalability without compromising performance.
Using JDBC Connection Pooling
In Java, the JDBC API provides support for connection pooling through DataSource
implementations such as Apache DBCP and HikariCP. These libraries offer configurable connection pooling mechanisms that can be seamlessly integrated into Java applications.
Code Example: Configuring Connection Pool using HikariCP
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("username");
config.setPassword("password");
config.setMaximumPoolSize(10); // Set maximum connections
DataSource dataSource = new HikariDataSource(config);
Connection connection = dataSource.getConnection(); // Obtain connection from the pool
// Perform database operations
connection.close(); // Connection returned to the pool
The above code snippet illustrates the usage of HikariCP to configure and utilize connection pooling, thereby enhancing the efficiency of database connectivity.
Closing the Chapter
In the realm of Java development, the significance of employing connection pooling cannot be overstated. By sidestepping the pitfalls associated with the absence of connection pooling, Java applications can achieve optimal performance, scalability, and resource utilization. Embracing connection pooling is not merely a best practice, but an indispensable facet of building robust and high-performing Java applications.
In conclusion, the incorporation of connection pooling stands as a pivotal stride towards fortifying the foundation of Java applications, ensuring their resilience in the face of burgeoning demands. It is imperative for Java developers to acknowledge the transformative impact of connection pooling and integrate it comprehensively into their development endeavors.
By recognizing the pitfalls of neglecting connection pooling and harnessing its benefits, Java applications can ascend to higher echelons of efficiency and reliability, empowering developers to construct systems that are robust, responsive, and primed for seamless scalability.
In essence, connection pooling serves as an indispensable mechanism for orchestrating harmonious interactions between Java applications and their underlying databases, culminating in a symbiotic relationship characterized by efficiency, resilience, and unwavering performance.
Utilizing connection pooling is not merely an option; it represents a foundational imperative for any Java application seeking to carve a path towards optimal performance, scalability, and resource utilization. As the essence of Java development continues to evolve, the relevance of connection pooling remains steadfast, serving as a linchpin for engineering databases interactions that epitomize efficiency, agility, and steadfast reliability.