Handling Command-Line Arguments with JOpt Simple
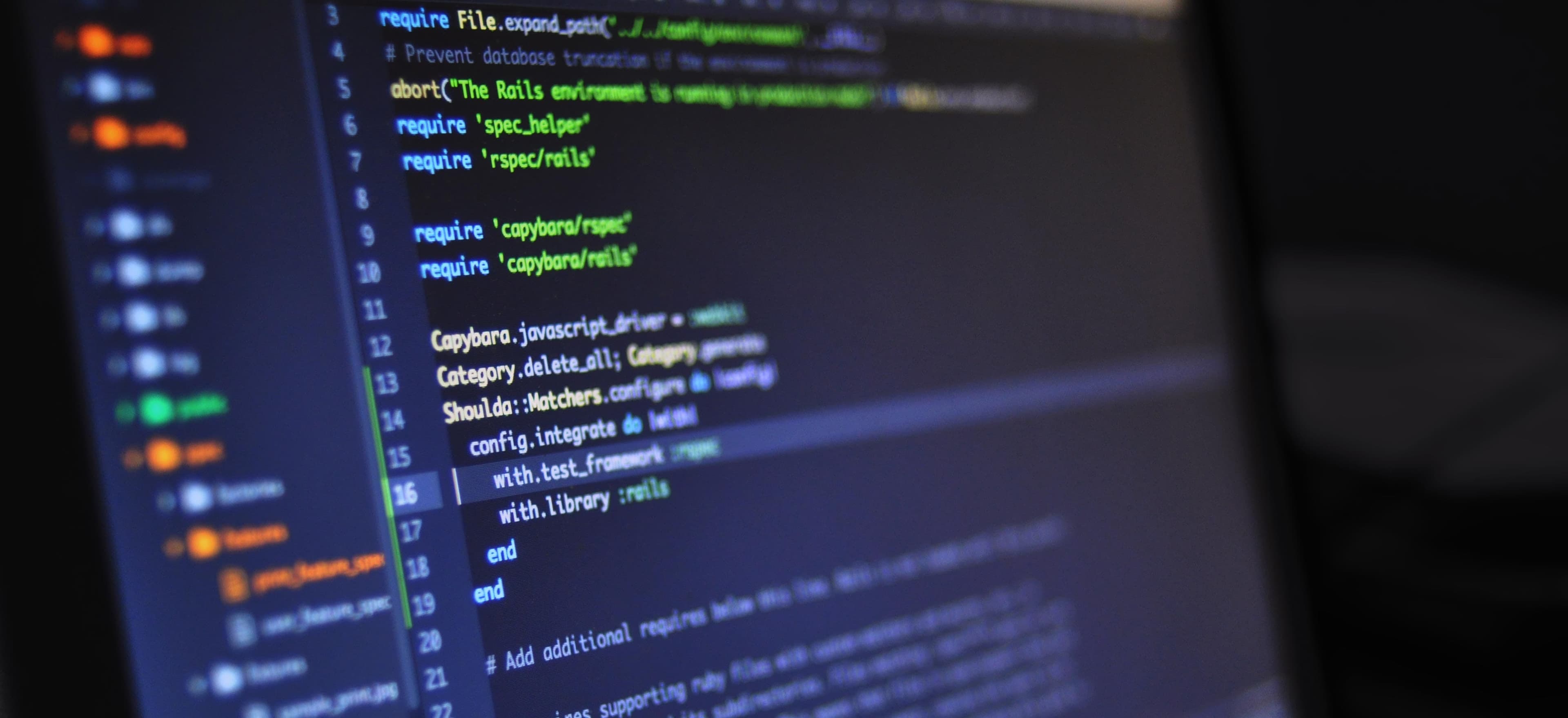
- Published on
Handling Command-Line Arguments with JOpt Simple
When it comes to writing command-line applications in Java, it's essential to have a robust library for parsing and handling command-line arguments. This is where JOpt Simple comes in. JOpt Simple is a simple, yet powerful library for parsing command-line options and arguments in Java.
In this blog post, we'll explore how to use JOpt Simple to handle command-line arguments in your Java applications. We'll cover the basics of parsing and handling options and arguments, and we'll provide some practical examples to demonstrate its usage.
What is JOpt Simple?
JOpt Simple is a command line parsing library for Java that makes it easy to parse command line options and arguments. It provides a simple and intuitive API for defining and parsing command line options, making it a popular choice for developers working on command-line applications in Java.
With JOpt Simple, you can define command line options and their associated arguments, parse the command line input, and handle the parsed options and arguments in your application. It simplifies the process of handling command-line input, allowing you to focus on the core logic of your application.
Getting Started with JOpt Simple
To get started with JOpt Simple, you'll need to include the JOpt Simple library in your Java project. You can add JOpt Simple as a dependency using Maven, Gradle, or by manually including the JAR file in your project.
Here's an example of how to include JOpt Simple as a Maven dependency:
<dependencies>
<dependency>
<groupId>net.sourceforge.jopt-simple</groupId>
<artifactId>jopt-simple</artifactId>
<version>5.0.3</version>
</dependency>
</dependencies>
Once you have JOpt Simple included in your project, you can start using it to parse and handle command-line arguments.
Defining Options and Parsing Arguments
The first step in using JOpt Simple is to define the command line options and arguments that your application supports. You can define options using the OptionParser
class, which allows you to specify the short and long forms of the option, whether it requires an argument, and other properties.
Here's an example of defining command line options using JOpt Simple:
import joptsimple.OptionParser;
import joptsimple.OptionSet;
import joptsimple.OptionSpec;
public class CommandLineApp {
public static void main(String[] args) {
OptionParser parser = new OptionParser();
OptionSpec<String> inputOption = parser.accepts("input").withRequiredArg().ofType(String.class);
OptionSpec<Void> helpOption = parser.accepts("help");
OptionSet options = parser.parse(args);
if (options.has(helpOption)) {
System.out.println("Usage: myapp --input <file>");
} else if (options.has(inputOption)) {
String inputFile = options.valueOf(inputOption);
System.out.println("Input file: " + inputFile);
} else {
System.out.println("No input file specified. Use --help for usage.");
}
}
}
In this example, we define two options using OptionSpec
– inputOption
and helpOption
. The inputOption
specifies that it requires an argument of type String
, while the helpOption
does not require an argument.
We then parse the command line arguments using parser.parse(args)
and use the OptionSet
to check if the helpOption
is present or if the inputOption
is present with its specified argument.
Handling Parsed Options and Arguments
Once the command line arguments have been parsed, you can access the parsed options and arguments using the OptionSet
object. The OptionSet
provides methods to check if a specific option is present, retrieve the values of options that accept arguments, and perform other operations based on the parsed input.
Here's an example of handling parsed options and arguments using JOpt Simple:
OptionSpec<Integer> verbosityOption = parser
.acceptsAll(Arrays.asList("v", "verbose"), "Enable verbose mode")
.withOptionalArg()
.ofType(Integer.class)
.defaultsTo(1);
OptionSpec<Boolean> debugOption = parser
.accepts("debug", "Enable debug mode")
.withOptionalArg()
.ofType(Boolean.class)
.defaultsTo(false);
OptionSet options = parser.parse(args);
int verbosityLevel = options.valueOf(verbosityOption);
boolean debugMode = options.valueOf(debugOption);
if (verbosityLevel > 0) {
System.out.println("Verbose mode enabled with level " + verbosityLevel);
}
if (debugMode) {
System.out.println("Debug mode enabled");
}
In this example, we define options for enabling verbose and debug modes. The verbosityOption
specifies an optional integer argument with a default value of 1, while the debugOption
specifies a boolean argument with a default value of false
.
We then retrieve the values of these options using options.valueOf(verbosityOption)
and options.valueOf(debugOption)
and perform logic based on the parsed values.
Closing Remarks
In this blog post, we explored how to use JOpt Simple to handle command-line arguments in Java applications. We covered the basics of defining options, parsing arguments, and handling the parsed options and arguments in your application.
JOpt Simple provides a simple and intuitive API for parsing command-line options, making it easy to handle command-line input in your Java applications. With its straightforward approach, JOpt Simple is a valuable tool for developers working on command-line applications in Java.
If you're interested in learning more about JOpt Simple, you can check out the official documentation or explore the source code on GitHub.
We hope this blog post has provided you with a solid understanding of how to use JOpt Simple in your Java applications. Whether you're building a simple command-line tool or a complex console-based application, JOpt Simple can help you parse and handle command-line arguments with ease.