Reversing Unordered Linked List Efficiently
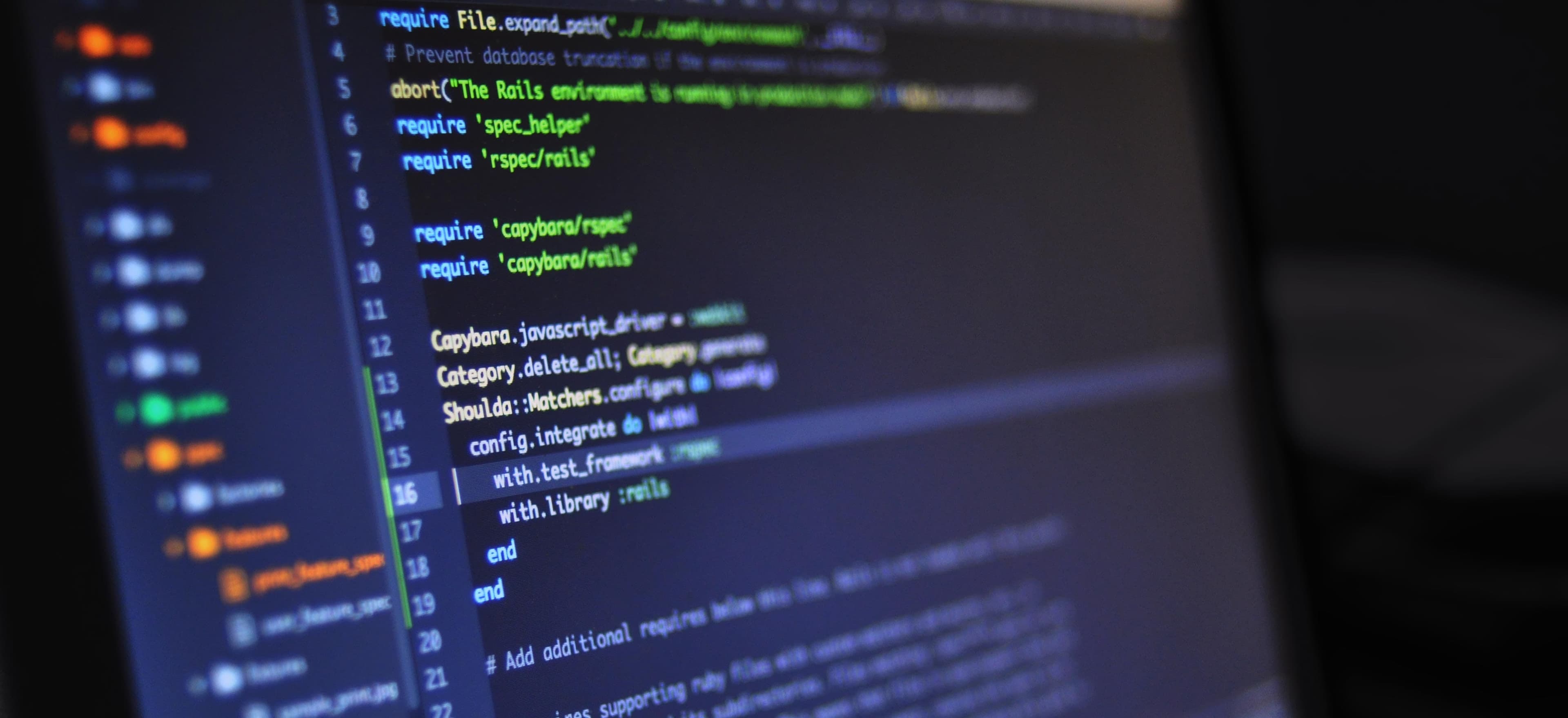
- Published on
Reversing Unordered Linked List Efficiently
When it comes to efficiently reversing an unordered linked list in Java, there are several approaches to consider. In this article, we'll explore different techniques and their implementation in Java to achieve this task.
Understanding Linked List Reversal
Before delving into the coding part, it's important to understand how linked list reversal works. In a linked list, each element (node) points to the next element, forming a sequence. When reversing the linked list, the pointers need to be adjusted so that the last element becomes the first, and so on. This process requires careful manipulation of the pointers to ensure that the elements are rearranged in the reverse order.
Approach using Iterative Method
One of the most commonly used methods to reverse a linked list is the iterative approach. This method involves traversing the list while rearranging the pointers to reverse the direction of the list.
Let's take a look at the Java code for reversing an unordered linked list using the iterative method:
class ListNode {
int val;
ListNode next;
ListNode(int val) {
this.val = val;
}
}
public class LinkedListReversal {
public ListNode reverseList(ListNode head) {
ListNode prev = null;
ListNode curr = head;
while (curr != null) {
ListNode nextTemp = curr.next;
curr.next = prev;
prev = curr;
curr = nextTemp;
}
return prev;
}
}
In the above code, we create a ListNode
class to represent the elements of the linked list. The reverseList
method takes the head of the linked list as input and iteratively reverses the list by manipulating the pointers.
The prev
pointer is used to keep track of the previously visited node, while curr
is used to traverse the list. Inside the loop, the nextTemp
variable is used to store the next node in the original list before updating the curr.next
pointer to point to the previous node.
This process continues until we have traversed the entire list, and finally, the prev
pointer becomes the new head of the reversed list.
Approach using Recursive Method
Another approach to reversing an unordered linked list is the recursive method. This method involves recursively traversing the list and adjusting the pointers to reverse the list.
Let's see how this can be implemented in Java:
public class LinkedListReversal {
public ListNode reverseList(ListNode head) {
if (head == null || head.next == null) {
return head;
}
ListNode reversedListHead = reverseList(head.next);
head.next.next = head;
head.next = null;
return reversedListHead;
}
}
In the recursive approach, we check for the base case where the head
is either null or the last element of the list. In this case, we simply return the head
as the reversed list.
Otherwise, we recursively call the reverseList
method with the head.next
, obtaining the reversed list. Then, we adjust the pointers to reverse the list and return the new head of the reversed list.
Choosing the Right Approach
When it comes to choosing between the iterative and recursive approaches, there are trade-offs to consider. The iterative approach is often preferred for its efficiency and minimal space complexity. It involves a simple loop and a few pointer manipulations, making it suitable for large linked lists without causing a stack overflow.
On the other hand, the recursive approach provides a more elegant and concise solution. However, it may not be as efficient as the iterative approach due to the overhead of recursive function calls, especially for very large lists.
The Closing Argument
Reversing an unordered linked list efficiently in Java is a fundamental operation with various implementation techniques. Whether you opt for the iterative approach for its efficiency or the recursive approach for its elegance, it's important to understand the trade-offs and choose the method that best suits your requirements.
By mastering the art of linked list reversal, you'll not only strengthen your understanding of data structures and algorithms but also enhance your problem-solving skills in Java programming.
Now that you have a deeper understanding of linked list reversal in Java, you might want to explore further by implementing other advanced operations or delving into more complex data structures.
Feel free to check out GeeksforGeeks for additional insights into linked list reversal and its applications in Java programming.