Custom Configuration Injection in Activiti: Best Practices
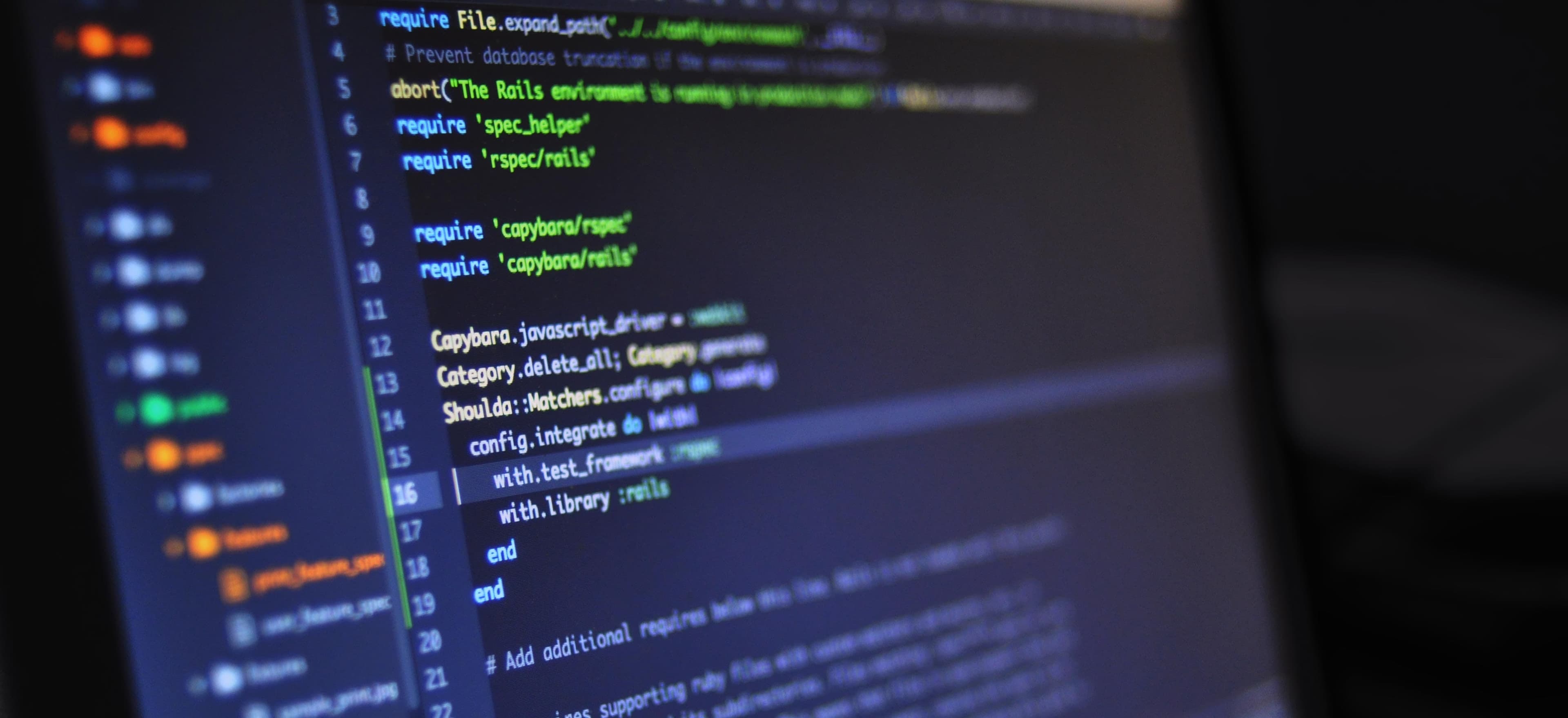
- Published on
Custom Configuration Injection in Activiti: Best Practices
Configuring and customizing the behavior of a BPM (Business Process Management) tool like Activiti is a crucial aspect of building robust and flexible applications. In this article, we will explore the best practices for injecting custom configurations in Activiti, leveraging the power of Java and the Activiti BPMN engine.
Why Custom Configuration Injection is Important
Custom configuration injection allows developers to tailor the behavior of Activiti to suit specific business requirements. Whether it's defining custom task behaviors, configuring process variables, or fine-tuning the process engine's settings, custom configuration plays a vital role in molding Activiti to fit the needs of the organization. It provides the flexibility to adapt to dynamic business processes and ensures that the BPM solution aligns perfectly with the business's unique workflow requirements.
Leveraging Java for Custom Configuration Injection
Java, being the primary language for Activiti development, provides a powerful and flexible foundation for injecting custom configurations into Activiti. Leveraging Java's rich ecosystem and dependency injection frameworks like Spring, custom configurations can be seamlessly integrated into Activiti processes.
Let's delve into some best practices for custom configuration injection in Activiti using Java:
1. Utilizing Spring Framework for Configuration Injection
Spring Framework offers robust support for dependency injection and configuration management. Leveraging Spring's @Autowired
and @Value
annotations, as well as Spring Beans, allows for seamless injection of custom configurations into Activiti components.
@Component
public class CustomTaskBehavior implements JavaDelegate {
@Value("${custom.task.timeout}")
private int taskTimeout;
@Override
public void execute(DelegateExecution execution) {
// Custom task behavior implementation using injected taskTimeout
}
}
In this example, the taskTimeout
value is injected from the Spring environment, allowing for dynamic customization of task behavior without the need for hard-coded values within the BPMN process definition.
2. Externalizing Configuration using Property Files
Externalizing configuration properties into separate files allows for easy management and modification of custom configurations without altering the application code. Java's Properties
class or Spring's PropertySourcesPlaceholderConfigurer
can be used to load external configuration properties into Activiti processes.
@Configuration
@PropertySource("classpath:custom-config.properties")
public class CustomConfiguration {
@Value("${process.timeout}")
private int processTimeout;
// Other custom configurations
}
By externalizing configuration properties, the flexibility to adjust settings without recompiling the code is achieved, promoting maintainability and adherence to the principle of separation of concerns.
3. Leveraging Activiti Configuration Beans
Activiti provides configuration beans that allow developers to customize various aspects of the BPM engine's behavior. By extending and configuring these beans, custom settings can be seamlessly integrated into the Activiti engine.
@Configuration
public class ActivitiEngineConfiguration {
@Autowired
private DataSource dataSource;
@Bean
public StandaloneProcessEngineConfiguration processEngineConfiguration() {
StandaloneProcessEngineConfiguration config = new StandaloneProcessEngineConfiguration();
config.setDataSource(dataSource);
// Other custom engine configurations
return config;
}
}
By creating and configuring the StandaloneProcessEngineConfiguration
bean, custom data sources, database settings, and other engine configurations can be injected into the Activiti process engine.
The Closing Argument
Custom configuration injection in Activiti is a powerful mechanism for tailoring the BPM engine to specific business requirements. By leveraging Java's capabilities, particularly with the use of Spring Framework and externalized property files, developers can seamlessly inject custom configurations into Activiti, promoting flexibility and maintainability.
Incorporating these best practices not only ensures a more adaptable BPM solution but also simplifies the process of adjusting configurations as business requirements evolve.
With a solid understanding of these best practices, developers can confidently build robust and highly customizable BPM solutions using Activiti, empowering organizations to efficiently model and execute their business processes.
For further exploration of Activiti and BPMN, consider referring to Activiti's official documentation and Camunda's BPMN overview.
Remember, the key to successful BPM solutions lies in the thoughtful and strategic application of custom configurations, empowering businesses to thrive in an ever-changing landscape.