Challenges of Load Balancing with Round Robin Scheduling
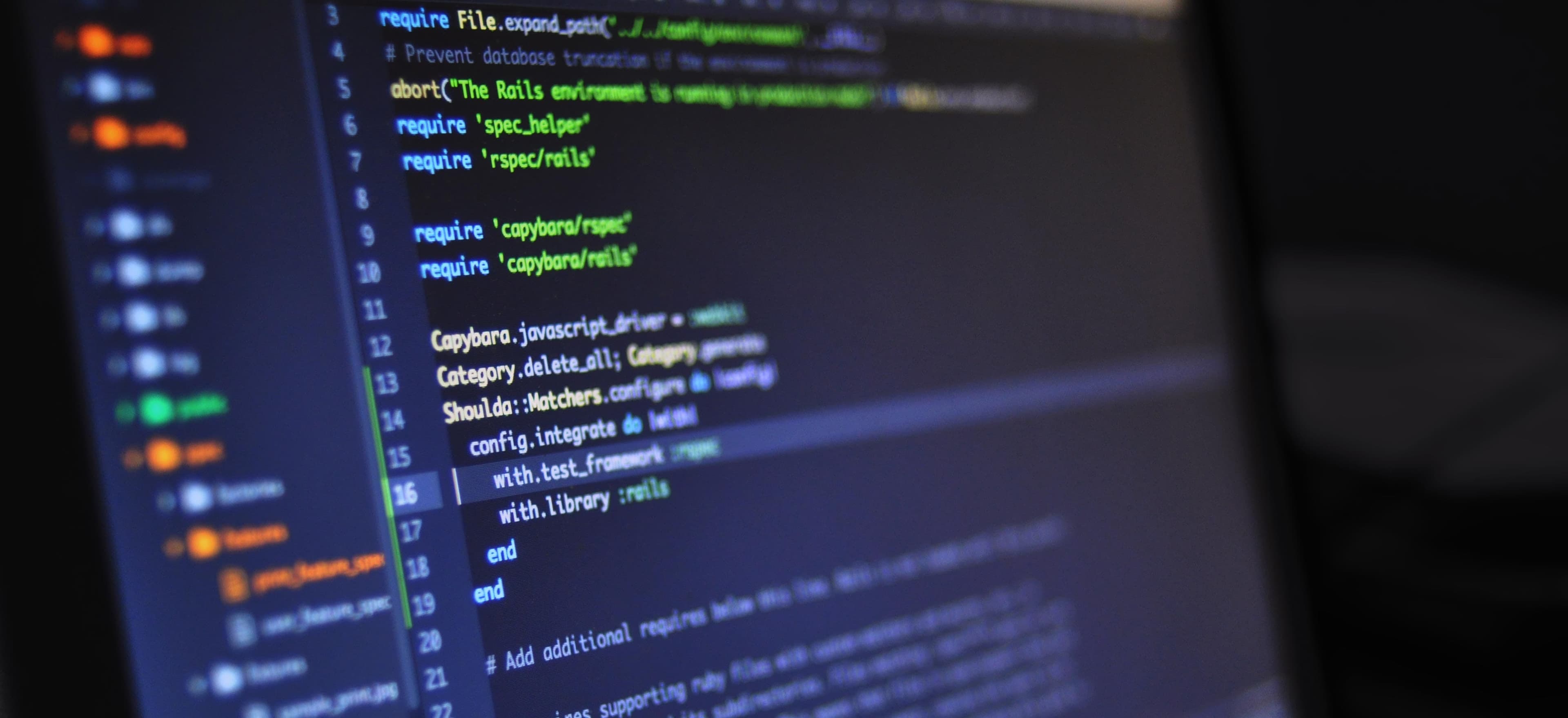
- Published on
The Challenges of Load Balancing with Round Robin Scheduling
Load balancing is a critical aspect of building scalable and reliable systems. It ensures that incoming requests are distributed across multiple servers to avoid overloading any single server. Round Robin Scheduling, a simple and widely-used load balancing algorithm, distributes incoming requests sequentially to a group of servers. While round robin scheduling provides a basic mechanism for load distribution, it also presents several challenges that can impact the performance and scalability of a system.
In this article, we will delve into the challenges of load balancing with round robin scheduling in the context of Java-based applications. We will explore the limitations of round robin scheduling and discuss potential solutions to mitigate its shortcomings.
Understanding Round Robin Scheduling
Round robin scheduling evenly distributes incoming requests across a pool of servers. The algorithm works by sequentially allocating each new request to the next server in the pool. Once the last server is reached, the next request is assigned to the first server, and the process continues in a circular manner.
Example of Round Robin Scheduling in Java
Let's consider a simple Java implementation of round robin scheduling using an array to represent a pool of servers.
public class RoundRobinLoadBalancer {
private String[] servers;
private int currentIndex;
public RoundRobinLoadBalancer(String[] servers) {
this.servers = servers;
this.currentIndex = 0;
}
public String getNextServer() {
String nextServer = servers[currentIndex];
currentIndex = (currentIndex + 1) % servers.length;
return nextServer;
}
}
In this example, the RoundRobinLoadBalancer
class maintains an array of servers and a currentIndex
to keep track of the next server to which a request will be routed. The getNextServer
method returns the next server in a round-robin fashion.
Challenges of Round Robin Scheduling
Unequal Server Capacities
One of the significant challenges of round robin scheduling is its inability to consider the varying capacities and workloads of individual servers. As requests are distributed equally regardless of each server's capabilities, some servers may become overloaded while others remain underutilized. This results in suboptimal resource utilization and can lead to performance degradation.
Inefficient with Long-Running Requests
Round robin scheduling does not account for the execution time of requests. When long-running requests are assigned to a server, it ties up the server's resources, potentially causing delays in processing subsequent requests. This can impact the overall responsiveness of the system and result in uneven request processing times.
Lack of Health Monitoring
Another challenge of round robin scheduling is the absence of health monitoring for servers. If a server becomes unhealthy or experiences degraded performance, round robin scheduling continues to route requests to the problematic server, potentially impacting the overall system's reliability and availability.
Solutions and Mitigations
While round robin scheduling presents these challenges, there are several strategies and mitigations to address them in the context of Java-based load balancing implementations.
Weighted Round Robin Scheduling
To mitigate the issue of unequal server capacities, a weighted round robin approach can be employed. This variant of the algorithm assigns different weights to servers based on their capacities. Servers with higher capacities are assigned higher weights, allowing them to receive a proportionally larger share of requests.
public class WeightedRoundRobinLoadBalancer {
private String[] servers;
private int[] weights;
private int currentWeightIndex;
public WeightedRoundRobinLoadBalancer(String[] servers, int[] weights) {
this.servers = servers;
this.weights = weights;
this.currentWeightIndex = 0;
}
public String getNextServer() {
String nextServer = servers[currentWeightIndex];
decrementWeight(weights, currentWeightIndex);
currentWeightIndex = selectNextServer(weights, currentWeightIndex);
return nextServer;
}
// Helper methods to handle weighted selection
// decrementWeight and selectNextServer methods implementation will be based on the weight selection logic
}
In this example, the WeightedRoundRobinLoadBalancer
class maintains an array of servers and their corresponding weights. The getNextServer
method selects the next server based on the weighted round robin logic.
Dynamic Health Monitoring and Load Adjustment
To address the lack of health monitoring, a dynamic health monitoring system can be integrated into the load balancing mechanism. Health checks can be performed regularly, and unhealthy servers can be temporarily removed from the pool until they recover. Java provides libraries such as Hystrix and Spring Cloud for implementing dynamic health monitoring and load adjustment in distributed systems.
Load-Based Routing
In scenarios where long-running requests can significantly impact server performance, load-based routing can be employed to consider the current load on servers. This approach ensures that requests are directed to servers based on their current utilization and capacity, thereby preventing overloading due to long-running tasks.
Wrapping Up
While round robin scheduling offers a simple and easy-to-implement load balancing strategy, it poses inherent challenges in handling varying server capacities, long-running requests, and server health monitoring. By understanding these challenges and exploring potential solutions such as weighted round robin scheduling, dynamic health monitoring, and load-based routing, Java-based systems can effectively address the limitations of round robin scheduling and achieve improved performance, scalability, and reliability.
In conclusion, the challenges posed by round robin scheduling can be mitigated through strategic enhancements and proactive monitoring, enabling Java applications to achieve efficient and balanced load distribution across server clusters.