Resolve Gradle Push to Maven Repository Authentication Issue
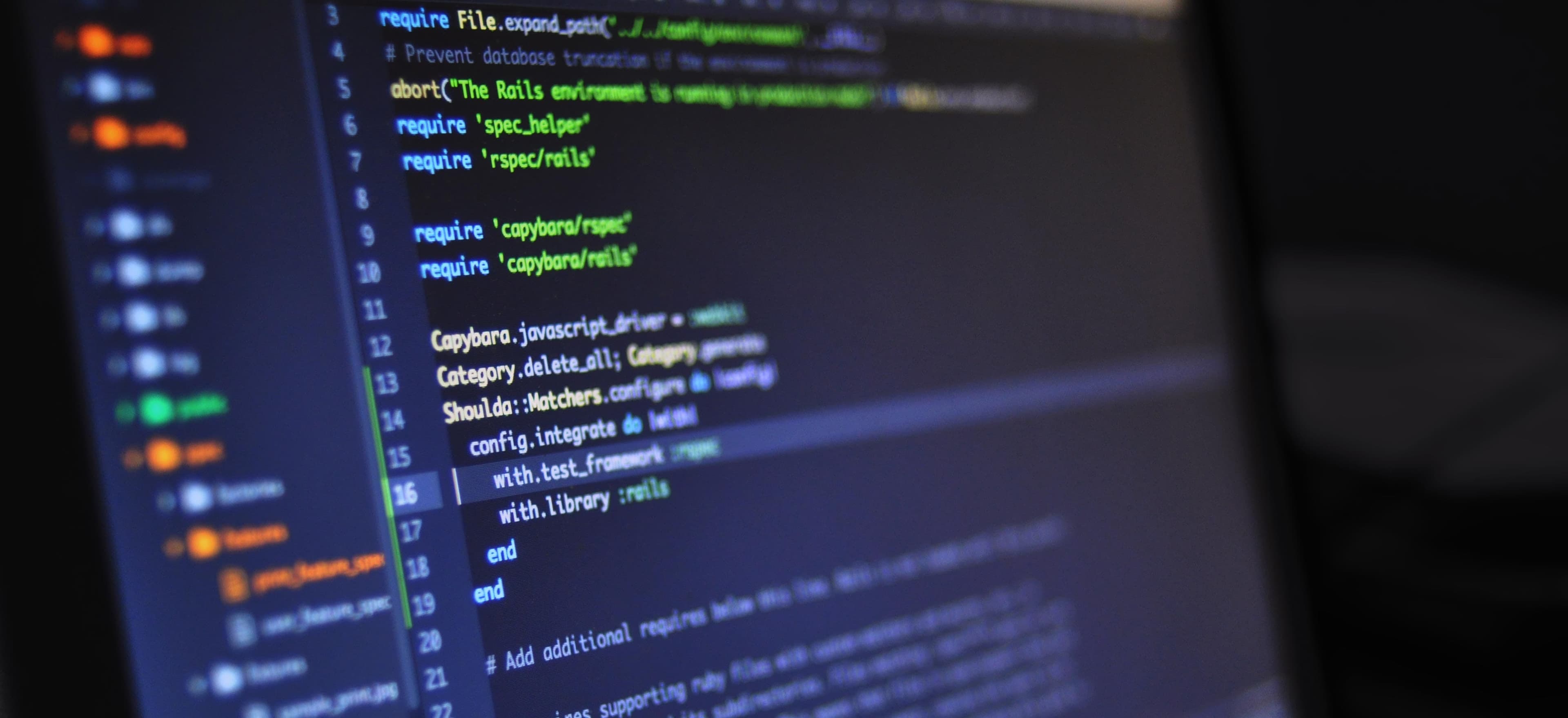
- Published on
Resolving Gradle Push to Maven Repository Authentication Issue
When working with Java projects, using Gradle as a build automation tool is quite common. However, when it comes to pushing artifacts to a Maven repository, you might encounter authentication issues, especially if you are using a private repository. In this blog post, we will explore the steps to resolve the Gradle push to Maven repository authentication issue.
Understanding the Problem
Before diving into the solution, it's crucial to understand the problem. When you attempt to push artifacts from Gradle to a Maven repository, you may encounter authentication errors. These errors occur when Gradle is unable to authenticate with the Maven repository due to incorrect credentials or misconfiguration.
Verifying Maven Repository Configuration
The first step in resolving the authentication issue is to verify the Maven repository configuration in your Gradle build file. Ensure that the repository URL, credentials, and other configuration details are correctly specified.
repositories {
maven {
url "https://your-maven-repo-url"
credentials {
username 'your-username'
password 'your-password'
}
}
}
Why is this important?
By verifying the Maven repository configuration, you ensure that Gradle has the correct information to authenticate and push artifacts to the repository.
Using Gradle Properties for Credentials
Hardcoding credentials in the build file is not recommended for security reasons. Instead, you can use Gradle properties to provide the credentials securely.
In your gradle.properties
file:
mavenUsername=your-username
mavenPassword=your-password
In your build file:
repositories {
maven {
url "https://your-maven-repo-url"
credentials {
username project.findProperty("mavenUsername") as String
password project.findProperty("mavenPassword") as String
}
}
}
Why is this recommended?
Using Gradle properties for credentials allows you to keep sensitive information out of the build file, enhancing security and maintainability.
Configuring Maven Repository Authentication
If you are pushing artifacts to a private Maven repository that requires authentication, it's essential to configure the authentication details properly. The repository URL, username, and password should be accurately specified.
publishing {
repositories {
maven {
url "https://your-maven-repo-url"
credentials {
username = project.findProperty("mavenUsername") as String
password = project.findProperty("mavenPassword") as String
}
}
}
}
What's the benefit?
Configuring Maven repository authentication ensures that Gradle uses the correct credentials when pushing artifacts, thereby resolving authentication issues.
Using Encrypted Maven Credentials
For an added layer of security, you can encrypt your Maven repository credentials using the gradle-encrypted-credentials
plugin. This plugin allows you to encrypt sensitive information to safeguard it from unauthorized access.
After applying the plugin to your Gradle build, you can encrypt the credentials:
./gradlew encryptCredentials
Then, use the encrypted values in your build file:
repositories {
maven {
url "https://your-maven-repo-url"
credentials {
username project.findProperty("encryptedMavenUsername") as String
password project.findProperty("encryptedMavenPassword") as String
}
}
}
How does this enhance security?
Encrypting Maven credentials adds an extra layer of protection to sensitive information, reducing the risk of unauthorized access.
Final Thoughts
In conclusion, resolving the Gradle push to Maven repository authentication issue involves verifying and configuring the Maven repository details, utilizing Gradle properties for credentials, and optionally encrypting the credentials for enhanced security.
By following these steps, you can ensure that Gradle successfully authenticates with the Maven repository and pushes artifacts without encountering authentication issues. This not only streamlines your build process but also enhances the security of sensitive information.
By implementing the best practices and making use of the available tools and techniques, you can overcome authentication challenges and maintain a seamless workflow when working with Maven repositories in your Java projects.
For additional reading on Gradle and Maven, check out the Gradle documentation and Maven documentation.