Troubleshooting JDK 14: Handling Switch Expression Errors
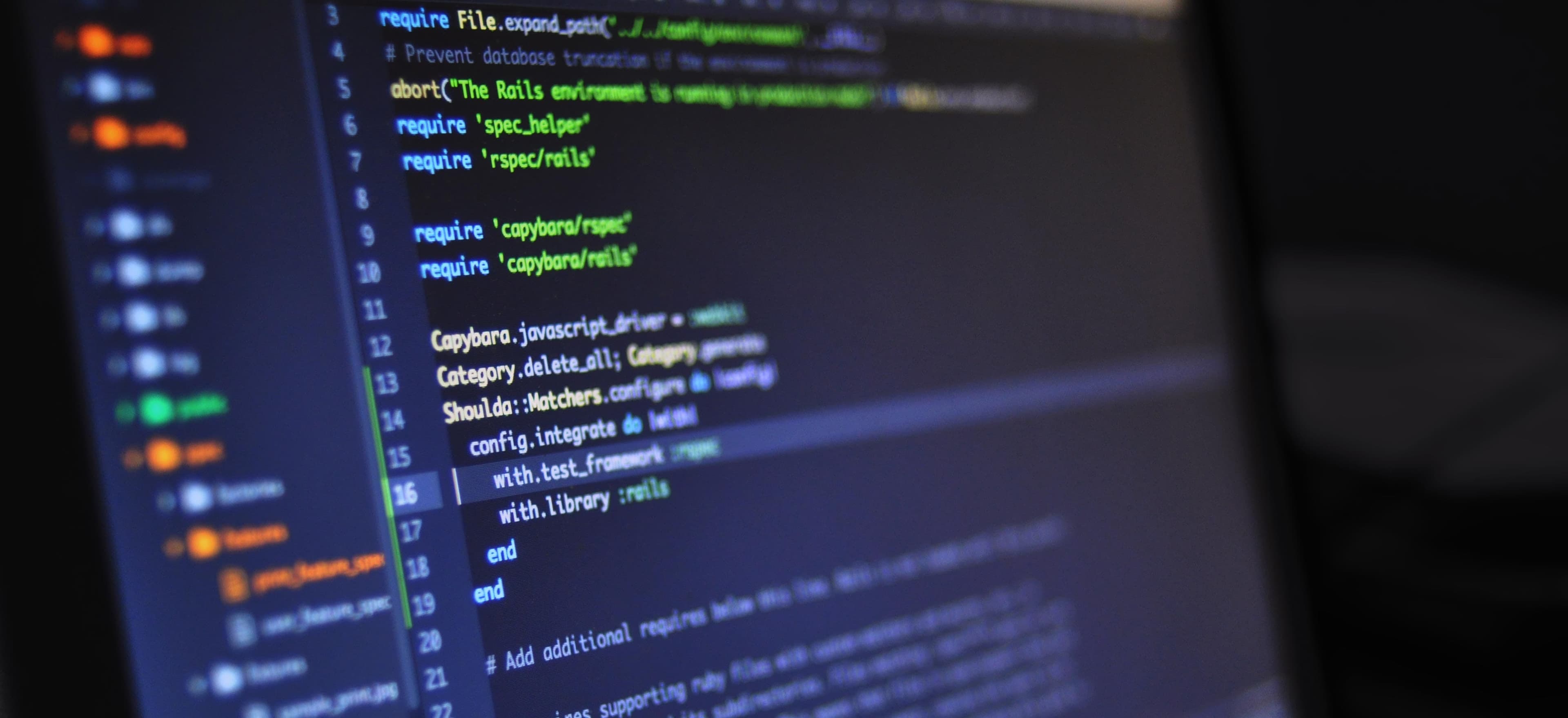
- Published on
Troubleshooting JDK 14: Handling Switch Expression Errors
Java Development Kit (JDK) 14 introduced several new features, including the long-awaited switch expressions. While switch expressions offer a more concise and flexible way to write switch statements, they also introduce new error types that developers need to be aware of. In this post, we will explore common errors related to switch expressions in JDK 14 and how to handle them effectively.
Understanding Switch Expressions in JDK 14
Switch expressions provide an enhanced approach to the traditional switch statement, allowing for a more flexible syntax and enabling the use of switch as an expression, which returns a value. This can lead to more readable and maintainable code, especially when handling multiple cases with different outcomes.
Here’s an example of a simple switch expression in JDK 14:
int day = 3;
String dayType = switch (day) {
case 1, 2, 3, 4, 5 -> "Weekday";
case 6, 7 -> "Weekend";
default -> "Invalid day";
};
System.out.println(dayType);
In this example, the switch expression takes an integer day
as input and returns a string dayType
based on the value of day
. The ->
operator separates the case labels and the corresponding expressions, providing a more readable way to express the logic.
Common Errors and How to Handle Them
1. "Constant expression required" Error
One common error that developers may encounter when working with switch expressions is the "constant expression required" error. This error occurs when the case labels or expressions do not evaluate to constant expressions at compile time.
For example, consider the following switch expression:
int number = 3;
String type = switch (number) {
case getNumber() -> "Type A";
case 3 -> "Type B";
default -> "Type C";
};
In this scenario, if the method getNumber()
does not return a constant expression, the compiler will raise the "constant expression required" error.
To resolve this error, ensure that all case labels in a switch expression are constant expressions. If a dynamic value is required, consider using if-else statements instead.
2. "Statement expected, but expression found" Error
Another common error when working with switch expressions is the "statement expected, but expression found" error. This error occurs when an expression is used where a statement is expected within the switch block.
Consider the following switch expression:
int number = 3;
String type = switch (number) {
case 1 -> "Type A";
case 2 -> "Type B";
default -> {
System.out.println("Invalid number");
}
};
In this example, the default case attempts to use a statement (printing to the console) instead of returning a value. Switch expressions require that all cases return a value, so using a statement in a case where an expression is expected will result in a compilation error.
To address this error, ensure that all cases in the switch expression return a value consistent with the type of the expression.
3. "Switch expressions are not supported in -source 8" Error
If you encounter the "Switch expressions are not supported in -source 8" error, it indicates that the switch expressions are not supported in the specified Java source version. This error often occurs when using older versions of the Java compiler that do not support switch expressions.
To resolve this error, ensure that the project is configured to use a Java compiler version that supports switch expressions, such as JDK 14 or later.
A Final Look
Switch expressions in JDK 14 offer a more concise and flexible approach to handling multiple cases, but they also introduce new error types that developers need to be mindful of. By understanding and addressing common switch expression errors, developers can effectively leverage this new feature to write more readable and maintainable code.
In this post, we have explored common errors such as "constant expression required" and "statement expected, but expression found," providing insights into how to handle them effectively. As you continue to work with JDK 14 and switch expressions, keep these troubleshooting tips in mind to streamline your development process and create robust, error-free code.
For more information on JDK 14 and switch expressions, refer to the official JDK 14 documentation and the Java Language Specification.