Improving Error Messages in Bean Validation 1.1
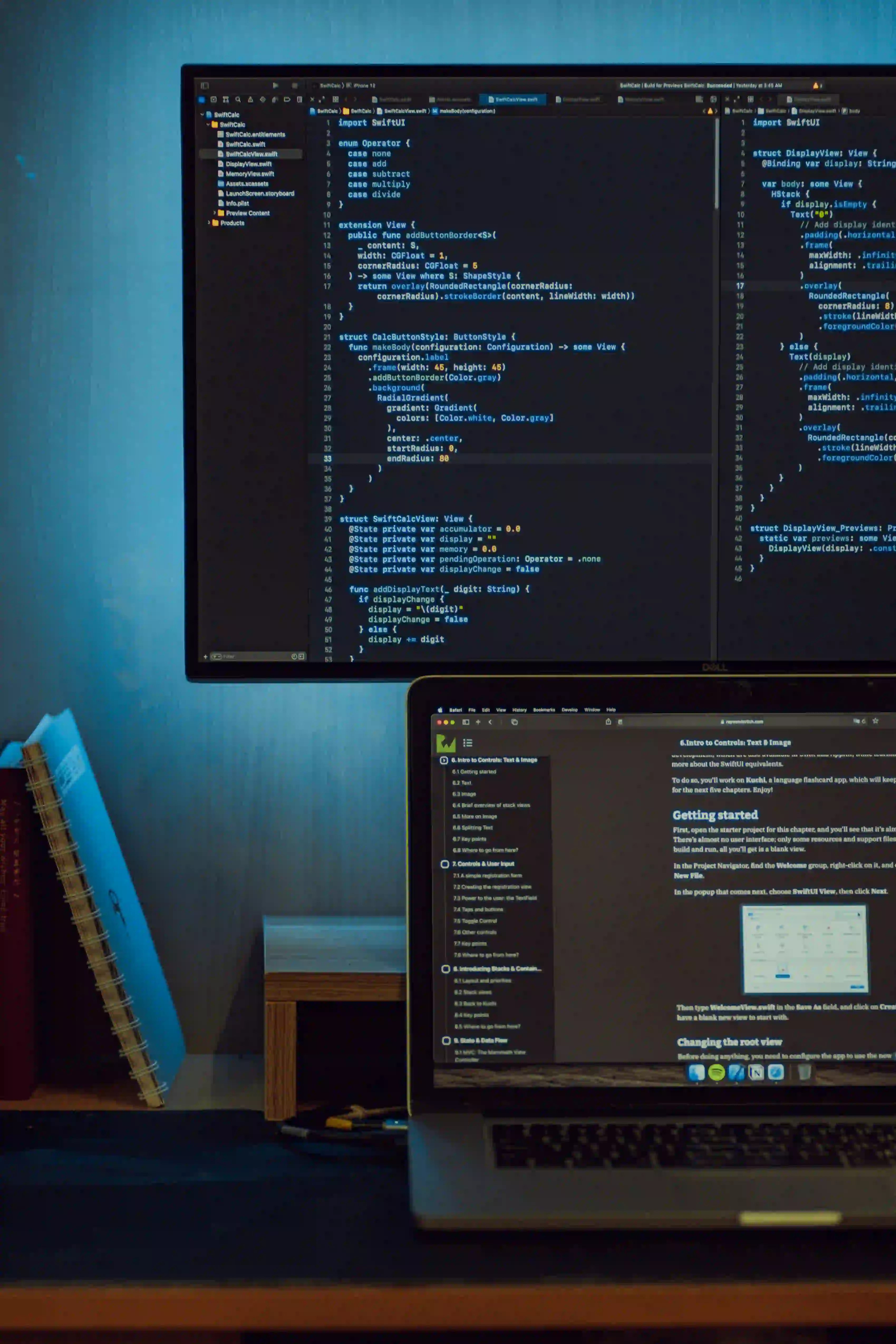
Improving Error Messages in Bean Validation 1.1
Bean Validation is a popular Java EE specification that provides a unified and standardized way to define and enforce constraints on domain model entities. While it offers a variety of built-in constraints for validating properties, one common complaint is the lack of user-friendly error messages when validation fails. This blog post will explore how to improve error messages in Bean Validation 1.1 to provide more informative feedback to users.
Understanding the Default Error Messages
When a validation constraint fails, Bean Validation produces a default error message that may not be very user-friendly or informative. For example, consider the following simple entity class with validation constraints:
public class User {
@NotNull
private String username;
@Email
private String email;
// Getters and setters
}
If we attempt to validate an instance of this class that fails the constraints, the default error messages may look like this:
username: must not be null
email: must be a well-formed email address
While these messages technically convey the validation errors, they lack context and are not very user-friendly.
Customizing Error Messages
Bean Validation allows for customizing error messages by providing a more descriptive message directly within the annotation:
public class User {
@NotNull(message = "Username is required")
private String username;
@Email(message = "Invalid email format")
private String email;
// Getters and setters
}
With these custom messages, the validation errors will now produce more user-friendly feedback:
username: Username is required
email: Invalid email format
Customizing error messages directly within the annotations is a simple and effective way to provide better feedback to users when validation fails.
Externalizing Error Messages to Properties Files
While customizing error messages within annotations works well for simple applications, it may not be the best approach for larger projects with multiple constraints and multiple languages.
Bean Validation provides the ability to externalize error messages to properties files. By default, Bean Validation will look for a file named ValidationMessages.properties
on the classpath. You can customize this file, specifying error messages for each constraint violation.
For example, here's how you can define custom error messages in the ValidationMessages.properties
file:
javax.validation.constraints.NotNull.message=This field is required
javax.validation.constraints.Email.message=Invalid email format
By externalizing error messages to a properties file, you can easily manage and translate messages for different languages or maintain a consistent set of messages across the application.
Using Message Interpolators
Bean Validation also provides message interpolation, which allows you to create dynamic error messages based on the validated value.
Let's consider a scenario where we have a custom constraint that ensures a user's age is between 18 and 99 years. We can use message interpolation to create a dynamic error message based on the validated value:
@Target({ METHOD, FIELD, ANNOTATION_TYPE })
@Retention(RUNTIME)
@Constraint(validatedBy = { })
@Documented
public @interface AgeConstraint {
String message() default "Invalid age: ${validatedValue}";
Class<?>[] groups() default { };
Class<? extends Payload>[] payload() default { };
}
In this example, we use ${validatedValue}
in the message to dynamically interpolate the validated value into the error message. So, when a validation error occurs, the message will include the actual value that failed validation.
Message interpolation provides a powerful way to create detailed and context-specific error messages, improving the overall user experience.
The Last Word
In this blog post, we have explored various techniques for improving error messages in Bean Validation 1.1. By customizing error messages, externalizing them to properties files, and utilizing message interpolation, you can provide more informative and user-friendly feedback when validation constraints are not met. This can greatly enhance the usability and overall user experience of your application.
By incorporating these best practices, developers can ensure that their applications provide clear and helpful error messages, ultimately leading to a more polished and professional end product.
For further reading on Bean Validation 1.1 and its features, you can explore the official Bean Validation documentation.