Common Causes of A-Class Loading Errors
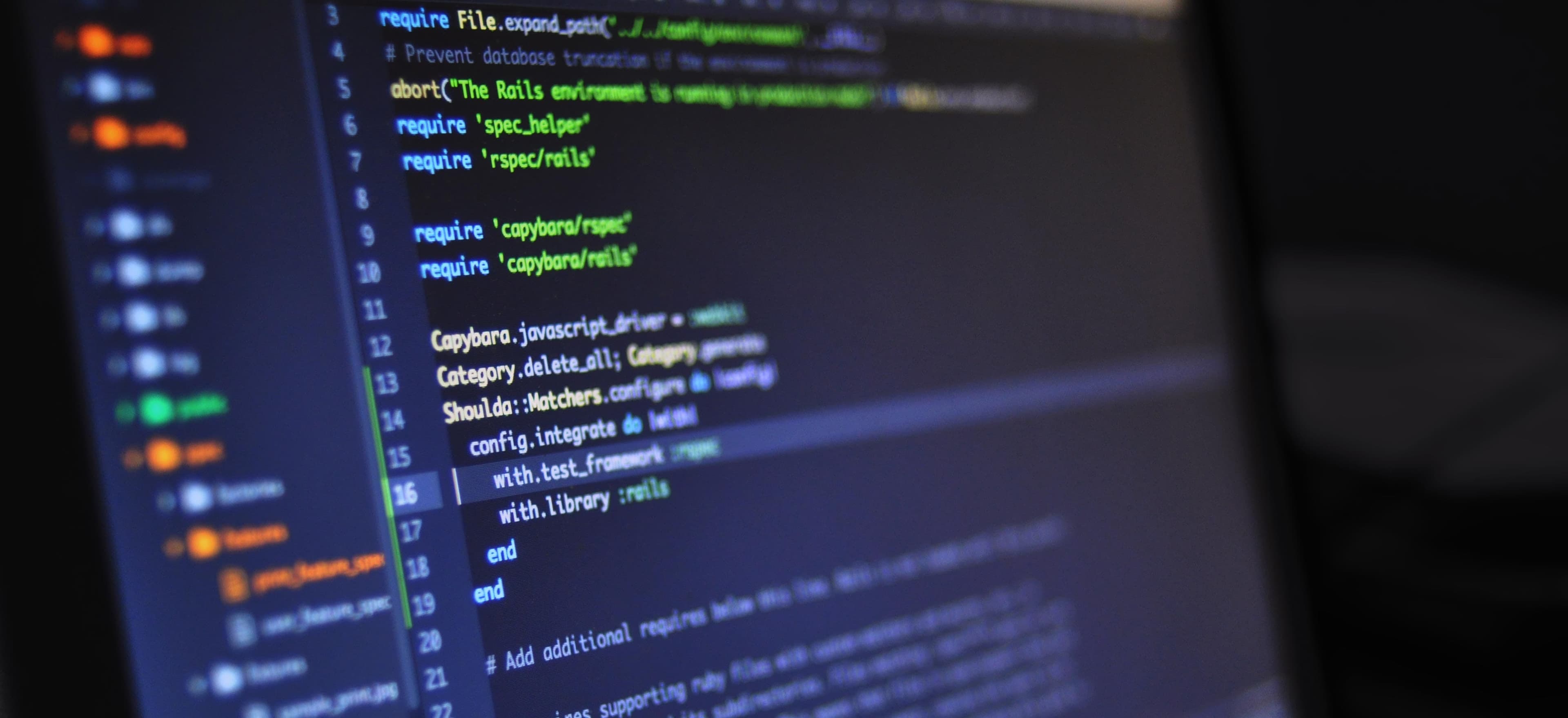
- Published on
Understanding A-Class Loading Errors in Java
In Java, A-Class Loading Errors can be quite a common nuisance for developers. These errors occur when the Java Virtual Machine (JVM) encounters issues while loading classes. Understanding the common causes of A-Class Loading Errors is crucial for efficient debugging and resolution. In this article, we will delve into some of the prevalent root causes behind these errors and explore potential solutions.
1. Class Not Found Errors
One of the most frequent A-Class Loading Errors is the ClassNotFoundException
. This error occurs when the JVM cannot find the class specified in the code. It can be caused by various factors, such as incorrect classpath configurations or missing dependencies.
Let's consider an example:
public class Main {
public static void main(String[] args) {
try {
// Attempting to load a class that does not exist
Class.forName("com.example.NonExistentClass");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
In this snippet, the ClassNotFoundException
will be thrown as the class "com.example.NonExistentClass" does not exist. To fix this, ensure that the classpath is accurately configured and that all required dependencies are present.
2. Incompatible Class Versions
Another common A-Class Loading Error is the UnsupportedClassVersionError
. This error occurs when the JVM encounters a class file with a version it does not support. This typically happens when trying to run a class file compiled with a newer version of Java on an older JVM.
Consider the following scenario:
public class Main {
public static void main(String[] args) {
// Attempting to load a class file compiled with a newer version of Java
SomeClass someObject = new SomeClass();
}
}
If SomeClass
was compiled with Java 11 and is being run on a JVM that only supports Java 8, an UnsupportedClassVersionError
will be thrown. To resolve this, ensure that the Java compiler and the JVM versions are compatible.
3. Incorrect Package Structure
A-Class Loading Errors can also stem from incorrect package structures. The NoClassDefFoundError
is a notable example, which occurs when the JVM is unable to locate a class despite the class being present during compilation.
Let's illustrate this with a simple code snippet:
package com.example.utilities;
public class Utility {
// Utility implementation
}
package com.example.main;
// Incorrectly referencing the Utility class
public class Main {
public static void main(String[] args) {
Utility utility = new Utility(); // Causes NoClassDefFoundError
}
}
In this example, the incorrect reference to the Utility
class leads to a NoClassDefFoundError
. To rectify this, ensure that the package and class references are accurately specified.
4. Problems with Custom Class Loaders
Custom class loaders can introduce complexities that lead to A-Class Loading Errors. Issues such as incorrectly overridden class loading behavior or mishandled class resolution can result in errors such as NoClassDefFoundError
or ClassNotFoundException
.
Consider the following custom class loader:
public class CustomClassLoader extends ClassLoader {
@Override
public Class<?> findClass(String name) throws ClassNotFoundException {
// Custom class loading logic
}
}
If the findClass
method does not implement the class loading logic correctly, it can lead to various A-Class Loading Errors. When working with custom class loaders, thorough testing and adherence to the class loading protocols are crucial.
5. Solutions and Best Practices
To preemptively address A-Class Loading Errors and maintain a robust Java application, several best practices can be employed:
a. Validate Classpath Configurations
Ensure that the classpath is correctly configured, including all necessary library dependencies.
b. Version Compatibility Checks
Verify that the Java compiler and the target JVM version are compatible, especially when introducing new language features.
c. Package Structure Verification
Regularly review and confirm the accuracy of package and class references to mitigate NoClassDefFoundError
occurrences.
d. Custom Class Loader Testing
Rigorously test custom class loaders and adhere to established loading protocols to avoid unforeseen issues.
In Conclusion, Here is What Matters
A-Class Loading Errors in Java can arise from a variety of causes, ranging from simple configuration mishaps to complex class loading intricacies. By understanding the common causes behind these errors and implementing best practices, developers can effectively tackle and prevent A-Class Loading Errors, ensuring the smooth functioning of their Java applications.
By delving into the depths of A-Class Loading Errors, developers can gain a comprehensive understanding of the intricacies involved in class loading, equipping them with the knowledge to navigate and resolve these issues effectively in their Java projects.
Remember, ensuring a robust class loading mechanism is pivotal for the seamless execution of Java applications.
For further insights into managing class loading and Java development best practices, Oracle's Java Documentation and Baeldung are valuable resources.
Happy coding!