Security Flaw: Common Mistakes in Generating and Validating JWT Tokens
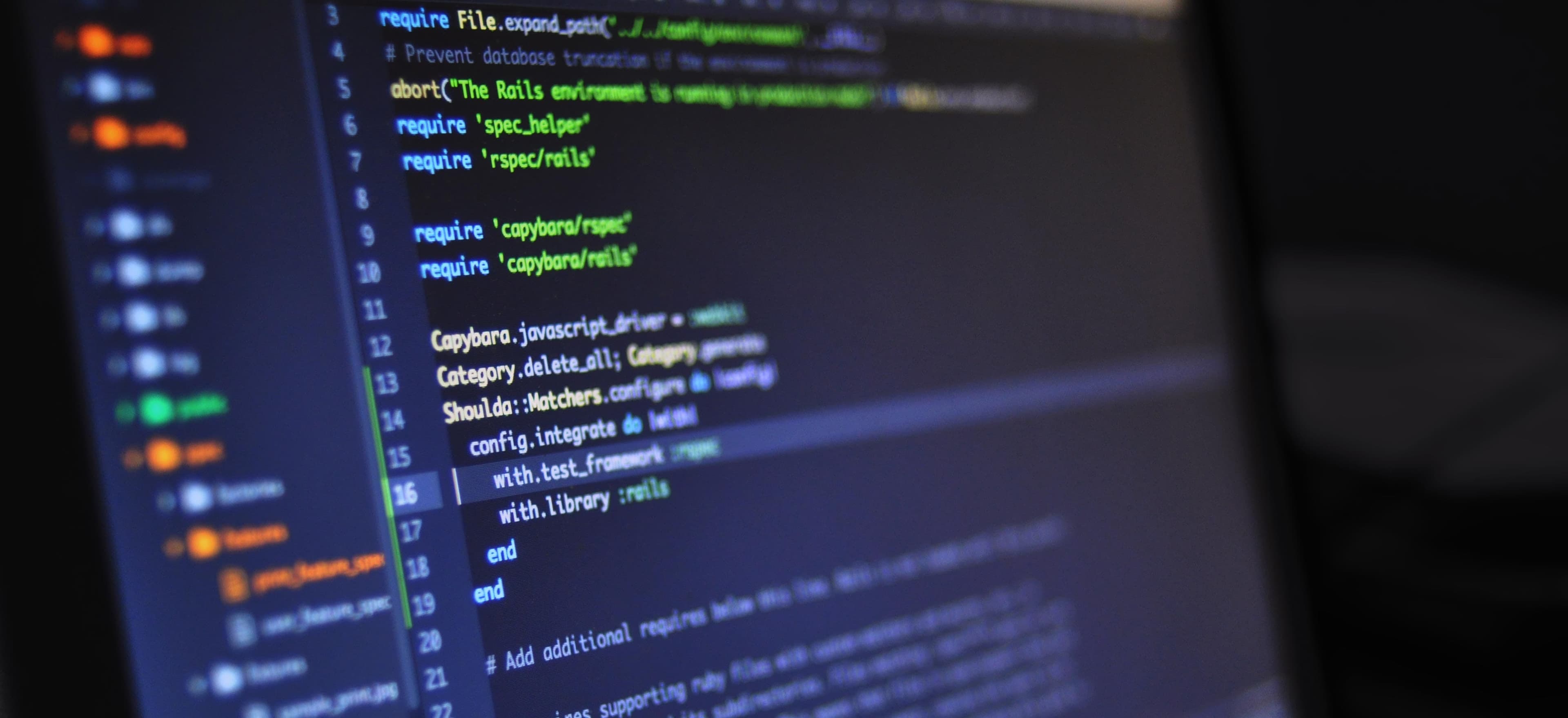
- Published on
Common Mistakes in Generating and Validating JWT Tokens
JSON Web Tokens (JWT) are widely used for handling user authentication and securing web applications. However, many developers struggle with properly generating and validating JWT tokens, which can lead to serious security vulnerabilities. In this article, we will discuss some common mistakes in generating and validating JWT tokens and explore best practices to mitigate these issues.
Generating JWT Tokens
Mistake 1: Using Weak Encryption Algorithms
When generating JWT tokens, it's crucial to use strong encryption algorithms to secure the token's payload. Many developers make the mistake of using weak algorithms such as HMAC with SHA-1, which is susceptible to collision attacks. Instead, it's recommended to use algorithms like HMAC with SHA-256 or RSA with at least a 2048-bit key for enhanced security.
// Bad practice: Using weak encryption algorithm
String token = Jwts.builder()
.setSubject("user123")
.signWith(SignatureAlgorithm.HS256, "secret")
.compact();
// Good practice: Using strong encryption algorithm
String token = Jwts.builder()
.setSubject("user123")
.signWith(SignatureAlgorithm.HS512, "secret")
.compact();
Mistake 2: Including Sensitive Information in the Payload
Another common mistake is including sensitive information such as passwords or private user data directly in the JWT token's payload. This can expose sensitive data if the token is compromised. Instead, JWT tokens should only contain non-sensitive information or a reference to the sensitive data stored securely on the server.
// Bad practice: Including sensitive information in the payload
String token = Jwts.builder()
.claim("password", "sensitive_password")
.compact();
// Good practice: Storing sensitive data on the server and including a reference in the payload
String token = Jwts.builder()
.claim("userId", "user123")
.compact();
Validating JWT Tokens
Mistake 1: Failing to Verify the Token's Signature
One of the most critical mistakes in JWT token validation is failing to verify the token's signature. Without proper signature verification, an attacker could tamper with the token and gain unauthorized access. Always ensure that the token's signature is verified using the appropriate secret key or public key, depending on the encryption algorithm used.
// Bad practice: Not verifying the token's signature
try {
Claims claims = Jwts.parser()
.setSigningKey("secret")
.parseClaimsJws(token)
.getBody();
// Process the token claims
} catch (SignatureException e) {
// Invalid token signature
}
// Good practice: Verifying the token's signature
try {
Claims claims = Jwts.parser()
.setSigningKey("secret")
.parseClaimsJws(token)
.getBody();
// Process the token claims
} catch (SignatureException e) {
// Invalid token signature
}
Mistake 2: Not Checking Token Expiration
Another common mistake in JWT token validation is failing to check the token's expiration (exp) claim. If the expiration check is omitted, an attacker could use an expired token to gain unauthorized access. Always validate the token's expiration time to ensure it hasn't expired before processing the token claims.
// Bad practice: Not checking token expiration
try {
Claims claims = Jwts.parser()
.setSigningKey("secret")
.parseClaimsJws(token)
.getBody();
// Process the token claims without checking expiration
} catch (ExpiredJwtException e) {
// Token expired
}
// Good practice: Checking token expiration
try {
Claims claims = Jwts.parser()
.setSigningKey("secret")
.require("exp", new Date())
.parseClaimsJws(token)
.getBody();
// Process the token claims after checking expiration
} catch (ExpiredJwtException e) {
// Token expired
}
Best Practices for Generating and Validating JWT Tokens
-
Use Strong Encryption Algorithms: Always use strong encryption algorithms like HMAC with SHA-256 or RSA with a sufficient key size to prevent cryptographic vulnerabilities.
-
Store Sensitive Data Securely: Avoid including sensitive information in the JWT token's payload. Store sensitive data securely on the server and include a reference in the token's payload.
-
Verify the Token's Signature: Always verify the token's signature to ensure its integrity and authenticity before processing the token claims.
-
Check Token Expiration: Validate the token's expiration time to prevent the use of expired tokens for unauthorized access.
By following these best practices, developers can significantly reduce the risk of security flaws in generating and validating JWT tokens, enhancing the overall security of their applications.
In conclusion, understanding the common mistakes in generating and validating JWT tokens is crucial for building secure and robust authentication mechanisms. Developers must be vigilant in implementing best practices to mitigate these issues and protect their applications from potential security vulnerabilities.
For further reading on JWT best practices and security considerations, check out the RFC 7519 specification for JWT and the OWASP JWT Cheat Sheet for comprehensive guidance.