Efficient Pagination with jOOQ & Spring: Best Practices
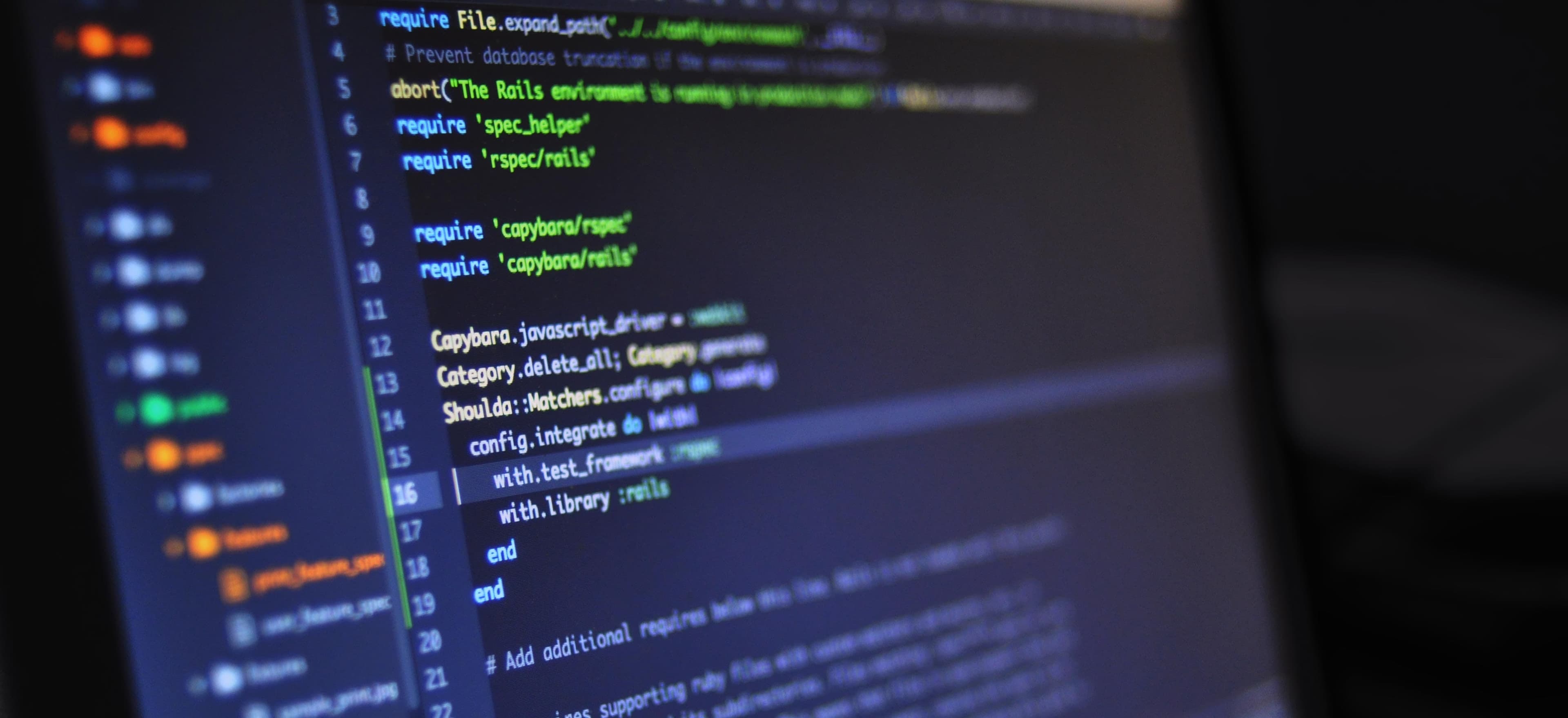
- Published on
Efficient Pagination with jOOQ & Spring: Best Practices
Pagination is a crucial aspect of any modern application that deals with large datasets. In this blog post, we will explore how to efficiently implement pagination using jOOQ and Spring, while also discussing best practices to maximize performance and user experience.
Why Pagination Matters
When dealing with large datasets, fetching and displaying all the results at once can significantly impact the application's performance. Pagination allows us to divide the dataset into manageable chunks, making it easier to display and navigate through the data.
Using jOOQ for Database Querying
jOOQ is a Java library that provides a fluent API for building type-safe SQL queries. It allows us to interact with the database using a domain-specific language, making database querying in Java more intuitive and type-safe.
Implementing Pagination with jOOQ and Spring
Let's dive into an example of how we can implement efficient pagination using jOOQ and Spring.
Setting Up the Project
First, we need to set up a Spring Boot project with jOOQ. We can use the org.springframework.boot:spring-boot-starter-jooq
starter to include jOOQ in our project.
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jooq</artifactId>
</dependency>
</dependencies>
Creating a Repository
Next, we can create a repository interface that extends org.jooq.DSLContext
. This interface will provide methods for fetching paginated data from the database.
import org.jooq.DSLContext;
public interface PaginatedRepository extends DSLContext {
List<Record> fetchPaginatedData(int offset, int limit);
}
Implementing Pagination Logic
Inside the repository implementation, we can use jOOQ's fetchOffset(int, int)
method to implement pagination logic.
import static com.example.generated.tables.MyTable.MY_TABLE;
@Component
public class PaginatedRepositoryImpl implements PaginatedRepository {
@Override
public List<Record> fetchPaginatedData(int offset, int limit) {
return select()
.from(MY_TABLE)
.offset(offset)
.limit(limit)
.fetch();
}
}
In this example, MY_TABLE
is a generated jOOQ table object representing the table from which we want to fetch paginated data.
Utilizing Pagination in the Service Layer
We can then use the PaginatedRepository
in a service layer to fetch paginated data based on the requested page and page size.
@Service
public class DataService {
private final PaginatedRepository paginatedRepository;
public DataService(PaginatedRepository paginatedRepository) {
this.paginatedRepository = paginatedRepository;
}
public List<Record> getPaginatedData(int page, int pageSize) {
int offset = (page - 1) * pageSize;
return paginatedRepository.fetchPaginatedData(offset, pageSize);
}
}
In the DataService
class, we calculate the offset
based on the requested page and page size, and then use the PaginatedRepository
to fetch the paginated data from the database.
Best Practices for Efficient Pagination
While implementing pagination with jOOQ and Spring, it's essential to keep in mind some best practices to ensure performance and scalability.
Indexing
Ensure that the database tables involved in pagination queries are properly indexed. Indexing the columns used in filtering and sorting can significantly improve query performance.
Caching
Consider caching the paginated results, especially for static or slowly changing datasets. This can reduce the load on the database and improve response times for subsequent pagination requests.
Efficient Data Retrieval
Avoid fetching unnecessary data from the database. Only retrieve the columns needed for display to minimize data transfer and processing overhead.
Asynchronous Pagination
For large datasets, consider implementing asynchronous pagination to offload the pagination logic to background tasks, providing a smoother user experience.
Use Query Execution Plan
Use the query execution plan provided by your database system to analyze and optimize the pagination queries. This can help identify potential bottlenecks and optimize query performance.
Key Takeaways
In this blog post, we explored how to efficiently implement pagination using jOOQ and Spring. By leveraging jOOQ's fluent API for building SQL queries and incorporating best practices for efficient pagination, we can ensure optimal performance and user experience when dealing with large datasets.
Implementing pagination effectively not only improves application performance but also provides a smoother and more responsive user interface. By following best practices and utilizing the capabilities of jOOQ and Spring, developers can create efficient and scalable pagination solutions for their applications.
To summarize, when dealing with large datasets, efficient pagination is not just a nice-to-have feature but a critical component in ensuring a high-performing and responsive application.
Start implementing efficient pagination with jOOQ and Spring today and witness the improvements in your application's performance and user experience.