Comparing Betweeness Centrality in Neo4j's Cypher and GraphStream
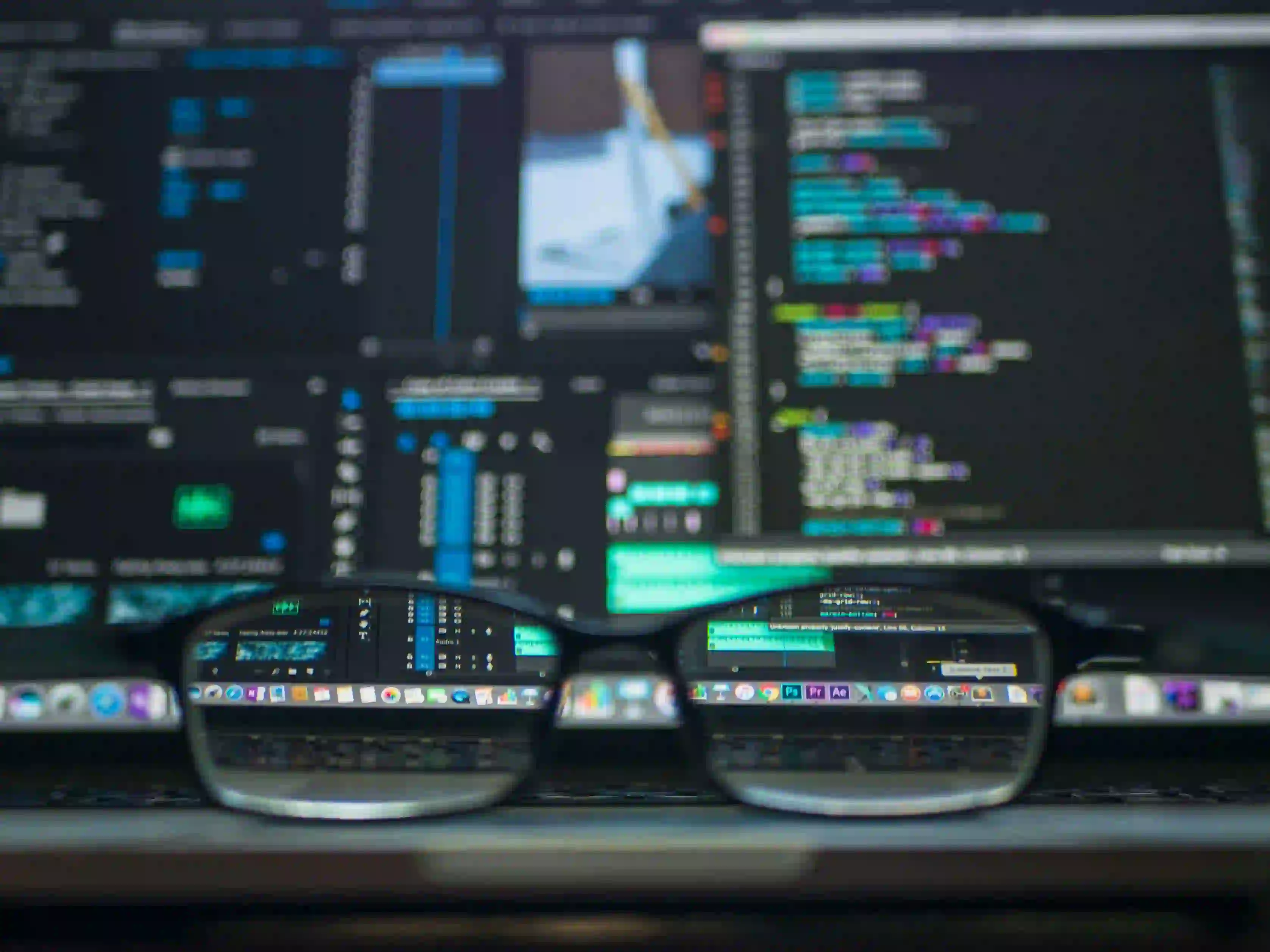
Comparing Betweeness Centrality in Neo4j's Cypher and GraphStream
When it comes to analyzing the importance and influence of nodes within a graph, betweenness centrality is a key metric. It quantifies the number of shortest paths that pass through a particular node, making it a crucial measure in network analysis, social network studies, and infrastructure management.
In this blog post, we'll delve into the calculation of betweenness centrality in two prominent tools: Neo4j's Cypher query language and the Java library GraphStream. By comparing the approaches of these two platforms, we'll aim to provide a comprehensive understanding of how betweenness centrality is computed and leveraged in different contexts.
Understanding Betweenness Centrality
Before we dive into the practical implementation, it's important to establish a clear understanding of betweenness centrality. In essence, a node's betweenness centrality is determined by the number of shortest paths between all pairs of nodes in the graph that pass through that specific node. While a higher betweenness centrality value indicates greater influence, it also suggests that the removal of the node could disrupt the flow of information or resources in the network.
Calculating Betweenness Centrality in Neo4j's Cypher
Given the widespread use of Neo4j as a powerful graph database, calculating betweenness centrality using Cypher is a valuable skill for graph analysts and developers. Neo4j provides the APOC library, which offers a convenient implementation of the betweenness centrality algorithm.
// Calculate Betweenness Centrality using APOC in Neo4j
CALL apoc.betweenness(['Person'],null) YIELD node, score
RETURN node.name AS Node, score AS BetweennessCentrality
ORDER BY score DESC
In the above Cypher query, we use the apoc.betweenness
procedure from the APOC library to compute the betweenness centrality for nodes labeled as 'Person'. The algorithm computes the score for each node and returns the results along with the node names and their respective betweenness centrality scores.
Exploring Betweenness Centrality with GraphStream in Java
On the other hand, GraphStream is a Java library that provides various algorithms for analyzing and visualizing graphs. To calculate betweenness centrality using GraphStream, we can utilize its built-in functionality and leverage it within a Java application.
// Calculate Betweenness Centrality using GraphStream in Java
Graph graph = new SingleGraph("ExampleGraph");
// Add nodes and edges to the graph
BetweennessCentrality bcb = new BetweennessCentrality();
bcb.init(graph);
bcb.compute();
for(Node node : graph) {
System.out.println("Node: " + node.getId() + " Betweenness Centrality: " + bcb.getCentrality(node));
}
In the Java example above, we create a graph using GraphStream, add nodes and edges, and then initialize the BetweennessCentrality
algorithm to compute the betweenness centrality for each node in the graph. Subsequently, we iterate through the nodes and retrieve their respective betweenness centrality scores.
Contrasting the Approaches
While both Neo4j's Cypher and GraphStream provide means to calculate betweenness centrality, the approaches differ fundamentally. Neo4j's Cypher language, being tailored for graph database queries, offers a declarative and SQL-like syntax that seamlessly integrates with graph data manipulation. On the other hand, GraphStream in Java necessitates a more programmatic approach, requiring explicit graph creation and algorithm initialization.
The use of Cypher in Neo4j is advantageous for directly querying graph data stored in the database and incorporating the results into broader data processing pipelines. Conversely, GraphStream's Java implementation provides a flexible way to interact with graph structures and algorithms within custom applications, offering greater control and customization.
Evaluating Performance and Use Cases
When comparing the two methods, performance and use cases play a pivotal role in determining the most suitable approach. Neo4j's Cypher query for betweenness centrality is well-suited for scenarios where the graph data is primarily stored and managed in Neo4j, enabling seamless integration with other graph-related queries and operations within the database.
On the other hand, GraphStream's Java implementation is advantageous in custom graph processing applications, simulations, and visualizations. It provides extensive control over graph manipulation and analysis, making it an ideal choice for scenarios where customized graph algorithms and dynamic graph modifications are paramount.
The Last Word
In conclusion, the calculation of betweenness centrality in Neo4j's Cypher and GraphStream in Java caters to distinct operational contexts. While Neo4j's Cypher offers a succinct and database-integrated approach for graph analysis, GraphStream's Java library provides a versatile platform for custom graph manipulation and algorithm implementation.
Understanding the nuances of each approach equips graph analysts and developers with the knowledge to select the most fitting method based on the specific requirements of their graph-related tasks. Whether leveraging Cypher in the context of Neo4j or harnessing the capabilities of GraphStream within intricate Java applications, the calculation of betweenness centrality remains a fundamental aspect of comprehensive graph analysis and interpretation.
By exploring the contrasting implementations of betweenness centrality in Neo4j's Cypher and GraphStream in Java, we've elucidated the diverse avenues through which this pivotal metric can be harnessed to derive valuable insights from graph data. As the realm of graph analysis continues to evolve, the choice between these methodologies remains integral to optimizing the exploration and interpretation of graph structures and relationships.