Unresolved issue with symbol manipulation in Scala lists
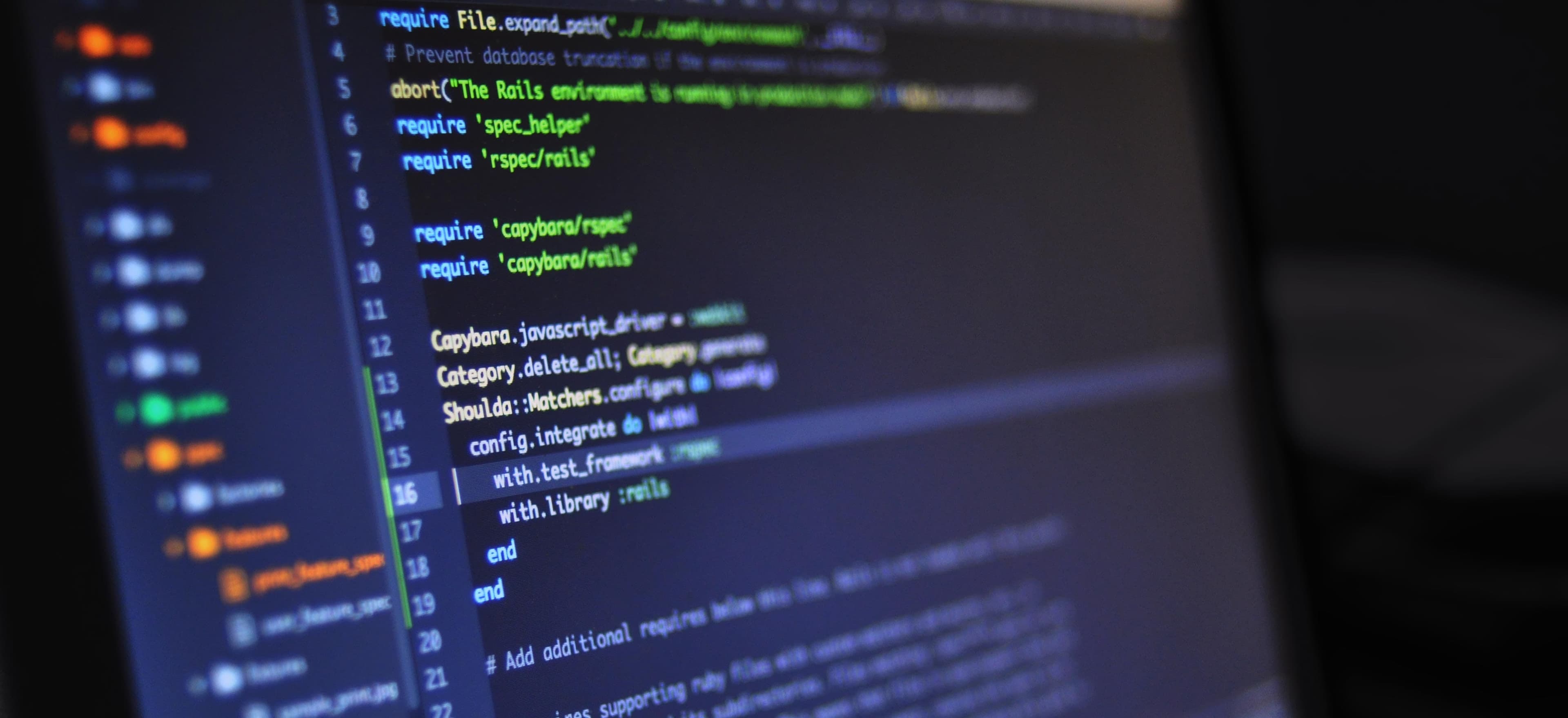
- Published on
Resolving Symbol Manipulation in Scala Lists
When working with Scala lists, you may encounter challenges related to symbol manipulation. This can be a roadblock, especially when you need to perform operations on the symbols within the lists. In this article, we will explore this issue and provide insights into how to effectively manipulate symbols in Scala lists.
Understanding Symbols in Scala
In Scala, symbols are a data type represented by the Symbol
class. They are often denoted by a prefix of a single quote, such as 'symbol
. Unlike strings, symbols are interned, meaning that any two occurrences of the same symbol literal refer to the same Symbol
object. This characteristic makes symbols particularly useful for representing identifiers in a program.
The Issue at Hand
When dealing with Scala lists that contain symbols, it can be challenging to perform operations that involve manipulating these symbols. This is due to the immutability of symbols, which prevents direct manipulation. Additionally, the distinction between symbols and strings adds a layer of complexity when working with list elements.
Let's consider a scenario where we have a list of symbols and we want to transform each symbol in the list. We may encounter difficulties due to the unique characteristics of symbols and the immutability associated with them.
Resolving the Issue
To effectively manipulate symbols within Scala lists, we can leverage functional programming concepts and higher-order functions.
Using map
to Transform Symbols
The map
function allows us to apply a given function to each element of a list, creating a new list of the results. This is a powerful tool for transforming the elements within the list, including symbols.
Let's consider an example where we have a list of symbols representing colors, and we want to convert each symbol to its corresponding string representation. We can achieve this using the map
function as follows:
val colorSymbols = List('red, 'green, 'blue)
val colorStrings = colorSymbols.map(_.name)
In this example, we utilize the map
function to transform each symbol into its string representation by accessing the name
property of the symbol. This approach allows us to effectively manipulate the symbols within the list without directly modifying the symbols themselves.
Leveraging Pattern Matching
Pattern matching is another powerful technique in Scala that can be used to manipulate symbols within lists. By defining custom patterns, we can effectively handle different cases based on the symbols present in the list.
Consider a scenario where we have a list of symbols representing geometric shapes, and we want to perform specific actions based on the type of shape. We can achieve this using pattern matching as demonstrated below:
val shapes = List('circle, 'square, 'triangle)
shapes.foreach {
case 'circle => println("Processing circle")
case 'square => println("Processing square")
case 'triangle => println("Processing triangle")
case _ => println("Unknown shape")
}
In this example, we use pattern matching within a foreach
loop to perform different actions based on the type of shape represented by each symbol in the list. This approach provides a concise and effective way to manipulate symbols within lists while accommodating different cases.
Final Thoughts
In conclusion, while symbol manipulation in Scala lists may pose initial challenges due to the immutability and unique characteristics of symbols, leveraging functional programming concepts such as map
and pattern matching empowers us to effectively manipulate symbols within lists. By embracing the principles of functional programming and understanding the distinct nature of symbols, we can navigate and resolve the issue of symbol manipulation in Scala lists efficiently.
For further exploration of Scala and functional programming, you can dive into the Scala documentation and Functional Programming in Scala book for comprehensive insights.