Handling Unmapped Properties in JAXB Serialization
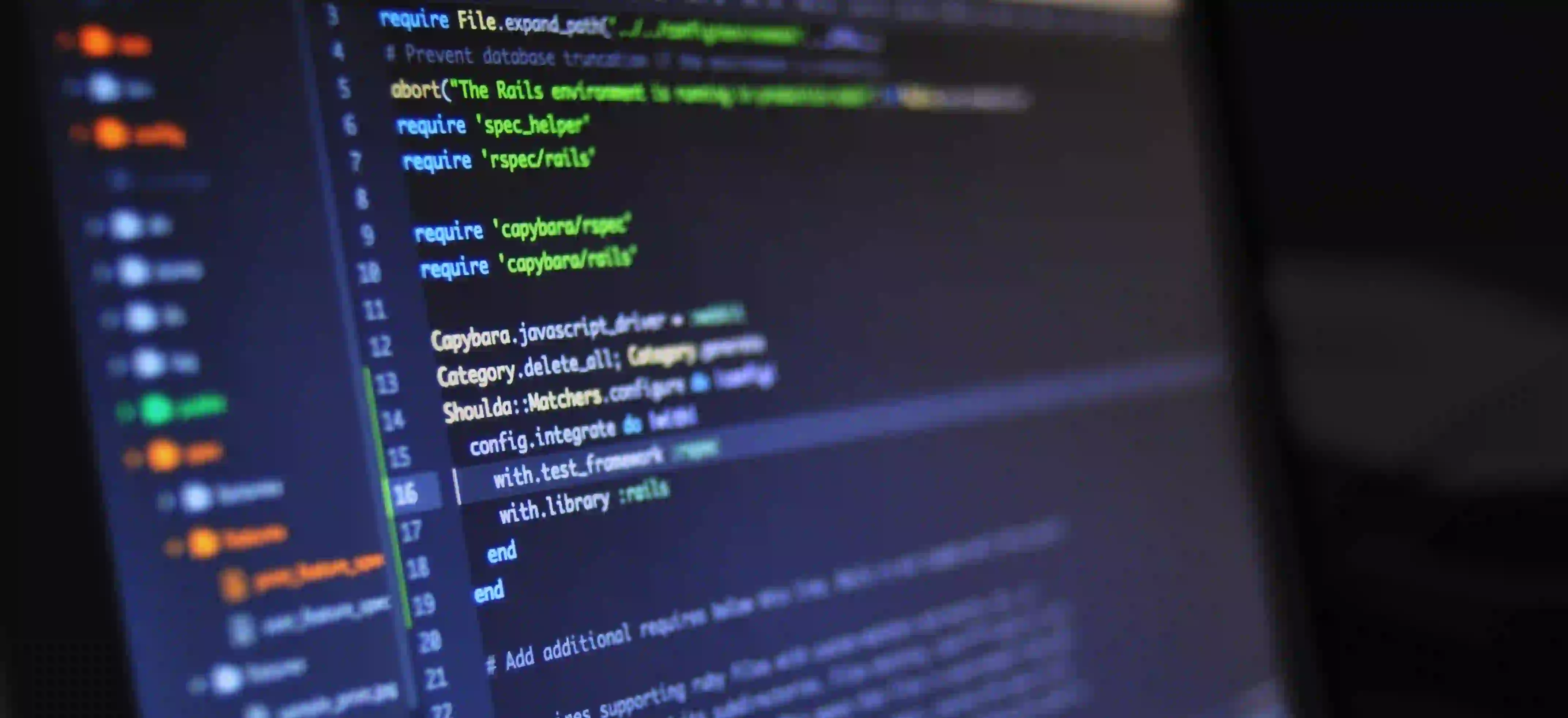
Handling Unmapped Properties in JAXB Serialization
When working with JAXB (Java Architecture for XML Binding), it's common to encounter scenarios where the Java classes used for XML binding contain properties not present in the XML schema. These unmapped properties might be useful for application-specific purposes, but their presence may cause issues during JAXB serialization.
In this blog post, we'll explore how to handle unmapped properties in JAXB serialization, discussing the problems they can cause and providing solutions to address them effectively.
Understanding Unmapped Properties
In JAXB, when we annotate a Java class for XML binding, the properties of the class are mapped to elements or attributes in the XML representation. However, if the Java class contains properties that do not have corresponding XML elements or attributes, these properties are considered unmapped.
An example of such unmapped properties can be auxiliary fields or transient properties used for internal processing within the Java application, but are irrelevant for XML representation.
When these unmapped properties are encountered during JAXB serialization, they can lead to unexpected behavior, such as incomplete XML representation or errors.
Problems Caused by Unmapped Properties
The presence of unmapped properties in JAXB serialization can result in several issues:
-
Incomplete XML Output: Unmapped properties may not be included in the XML output, leading to an incomplete representation of the object.
-
Serialization Errors: JAXB may throw serialization errors when it encounters unmapped properties, causing the serialization process to fail.
-
Unexpected Behavior: Unmapped properties might interfere with the serialization of mapped properties, leading to unexpected behavior in the XML output.
To address these issues, it's essential to handle unmapped properties properly during JAXB serialization.
Solution: Using @XmlAnyElement
and @XmlAnyAttribute
JAXB provides annotations such as @XmlAnyElement
and @XmlAnyAttribute
to handle unmapped properties during serialization. These annotations allow for the inclusion of unmapped properties in the XML output without explicitly mapping them to XML elements or attributes.
Example: Using @XmlAnyElement
Consider a class UserData
that contains unmapped properties for additional information:
import javax.xml.bind.annotation.XmlAnyElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAnyAttribute;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import java.util.Map;
@XmlRootElement
public class UserData {
private String name;
private Map<String, String> additionalInfo;
// Getters and setters for name and additionalInfo
@XmlAnyElement
@XmlJavaTypeAdapter(MapAdapter.class)
public Map<String, String> getAdditionalInfo() {
return additionalInfo;
}
}
In this example, the UserData
class includes the @XmlAnyElement
annotation for the additionalInfo
property. This annotation allows unmapped properties to be included as XML elements in the output.
Explanation
The @XmlAnyElement
annotation tells JAXB to include any unmapped properties as XML elements in the serialized output. Additionally, the @XmlJavaTypeAdapter
annotation is used to specify a custom adapter for the Map<String, String>
type, ensuring proper serialization of the additionalInfo
property.
Handling Unmapped Properties with Custom Serialization
Another approach to handling unmapped properties in JAXB serialization is to implement custom serialization and deserialization logic for the Java class using XmlAdapter
.
Example: Custom Serialization with XmlAdapter
Consider a simplified User
class with an unmapped property internalId
that does not need to be included in the XML output:
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
public class User {
private String name;
private int age;
private transient String internalId; // Unmapped property
// Getters and setters for name, age, and internalId
@XmlElement
public String getName() {
return name;
}
@XmlElement
public int getAge() {
return age;
}
@XmlJavaTypeAdapter(InternalIdAdapter.class)
public String getInternalId() {
return internalId;
}
}
In this example, the InternalIdAdapter
class acts as a custom adapter for the internalId
property to exclude it from the XML output:
import javax.xml.bind.annotation.adapters.XmlAdapter;
public class InternalIdAdapter extends XmlAdapter<String, String> {
@Override
public String unmarshal(String v) {
return v; // No need to unmarshal for this example
}
@Override
public String marshal(String v) {
return null; // Exclude internalId from XML output
}
}
Explanation
In this example, the InternalIdAdapter
class extends XmlAdapter
and overrides the marshal
method to exclude the internalId
property from the XML output. By using a custom adapter, we can control the serialization of unmapped properties according to our requirements.
Key Takeaways
In JAXB serialization, handling unmapped properties is crucial for ensuring a consistent and accurate XML representation of Java objects. By using annotations such as @XmlAnyElement
and @XmlAnyAttribute
, or implementing custom serialization with XmlAdapter
, we can effectively manage unmapped properties and control their serialization behavior.
By understanding the problems caused by unmapped properties and applying the provided solutions, developers can maintain control over the XML output of JAXB serialization, avoiding unexpected issues and ensuring the integrity of their XML representations.
For more information on JAXB and XML binding, refer to the official documentation for detailed insights into JAXB annotation usage and best practices.
In conclusion, addressing unmapped properties in JAXB serialization not only ensures the correctness of XML representations but also enhances the flexibility and control of Java object serialization in XML format.