Handling Absolute Integral Numbers in JDK 15
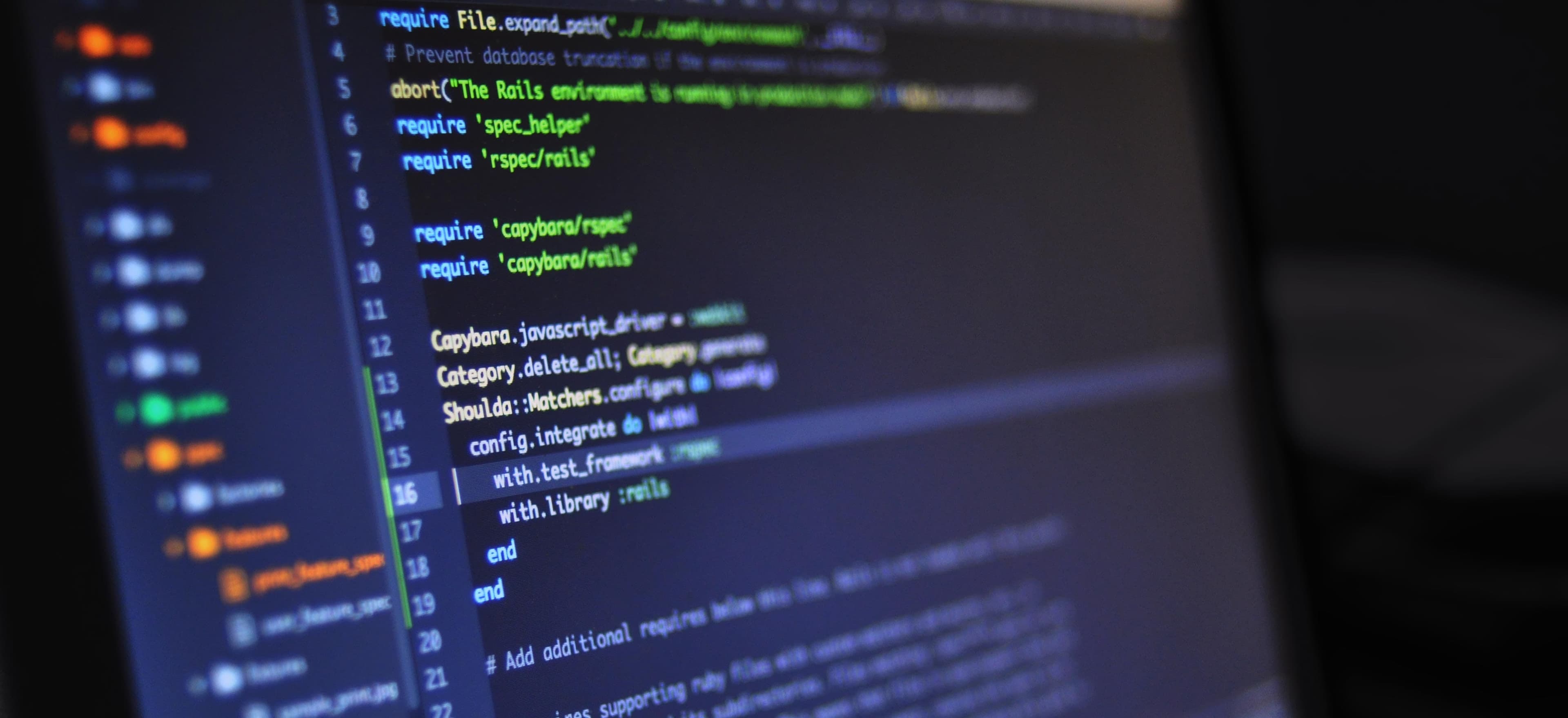
- Published on
Understanding Absolute Integral Numbers in Java
When working with numerical data in Java, it is important to have a thorough understanding of absolute integral numbers. In this article, we will explore how JDK 15 handles absolute integral numbers and how developers can effectively work with them in their programs.
What are Absolute Integral Numbers?
Absolute integral numbers, in the context of Java, refer to whole numbers that do not have a fractional or decimal component. These include integers and their corresponding wrapper classes such as int
, long
, short
, and byte
. When we talk about absolute integral numbers, we are primarily concerned with their magnitude irrespective of their sign.
Absolute Values in Java
In Java, obtaining the absolute value of an integral number can be achieved through several approaches. One of the most common methods is to use the Math.abs
method, which returns the absolute value of the specified integer argument. This method works for int
, long
, float
, and double
data types.
int num = -10;
int absNum = Math.abs(num);
System.out.println(absNum); // Output: 10
The above code snippet demonstrates how to obtain the absolute value of an int
using the Math.abs
method. It takes into account both positive and negative values and returns the absolute value accordingly.
New Features in JDK 15
In the latest release of JDK 15, there are new features that optimize the handling of absolute integral numbers. One notable addition is the Math.floorDiv
method, which performs integer division and returns the largest (closest to positive infinity) quotient value not greater than the exact mathematical quotient.
int quotient = Math.floorDiv(10, 3);
System.out.println(quotient); // Output: 3
In this example, the Math.floorDiv
method returns the integer division quotient of 10 divided by 3, which is 3. This method simplifies the process of obtaining integer division quotients, especially when dealing with positive and negative numbers.
The 'why' behind Math.floorDiv
The Math.floorDiv
method offers an improvement over the traditional division operator when working with integral numbers. It ensures consistent behavior in obtaining quotients, particularly in scenarios involving negative numbers where the standard division operator might produce unexpected results.
Moreover, the introduction of Math.floorDiv
aligns with Java's commitment to enhancing the language's capabilities for numerical operations, thereby providing developers with more robust tools to handle absolute integral numbers effectively.
Handling Overflows in Absolute Integral Numbers
When working with absolute integral numbers, developers often encounter situations where the value exceeds the maximum range of the data type. In JDK 15, the introduction of the Math.addExact
, Math.subtractExact
, Math.multiplyExact
, and Math.negateExact
methods addresses this concern by providing support for checked arithmetic operations.
try {
int result = Math.addExact(Integer.MAX_VALUE, 1);
System.out.println(result);
} catch (ArithmeticException e) {
System.err.println("Arithmetic overflow");
}
In the code snippet above, the Math.addExact
method adds 1 to the maximum value of an int
. Since the result exceeds the range of the int
data type, an ArithmeticException
is thrown. This behavior ensures that developers are aware of potential overflows and can handle them gracefully.
The 'why' behind Checked Arithmetic Operations
The introduction of checked arithmetic operations in JDK 15 represents a proactive approach to addressing potential overflows in absolute integral numbers. By providing methods such as Math.addExact
and Math.subtractExact
, Java empowers developers to write more robust and predictable code when performing arithmetic operations, thereby mitigating the risk of silent data corruption due to overflows.
Summary
In conclusion, JDK 15 introduces new features and enhancements that significantly improve the handling of absolute integral numbers in Java. The addition of methods such as Math.floorDiv
and checked arithmetic operations offers developers more robust tools to work with integral numbers effectively, ensuring consistent behavior and mitigating the risk of overflows.
As Java continues to evolve, it remains crucial for developers to stay abreast of these advancements and leverage them to write efficient and reliable code.
For further information on JDK 15 and its features, refer to the official release notes and the Java API documentation for detailed insights into absolute integral numbers and other improvements in the latest Java release.