Handling Deprecated Desired Capabilities in Selenium Testing
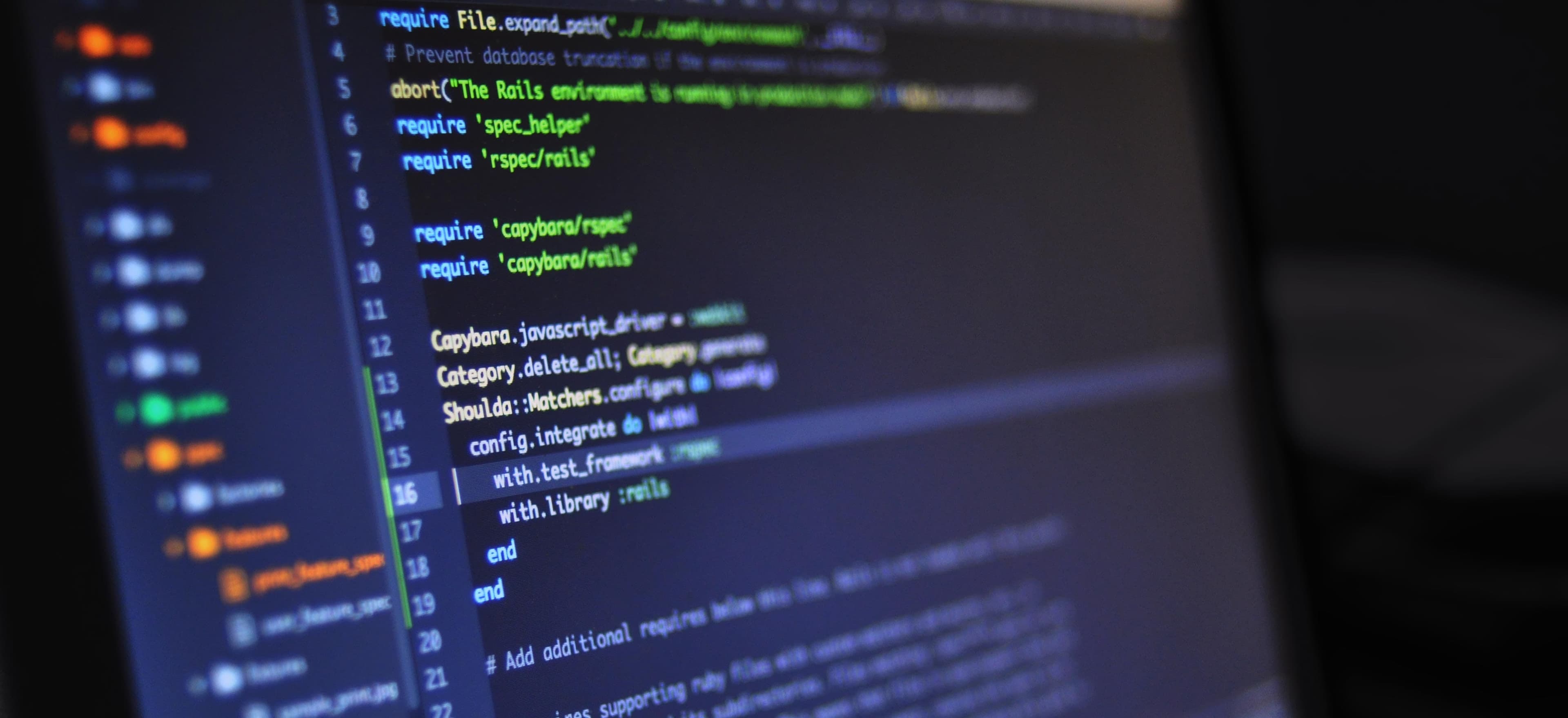
- Published on
Handling Deprecated Desired Capabilities in Selenium Testing
When it comes to automated testing with Selenium, Desired Capabilities play a vital role in specifying the attributes of the browser under test. However, with each new version, Selenium brings changes, and at times, certain capabilities are marked as deprecated. In this post, we’ll delve into handling deprecated Desired Capabilities in Selenium testing, understanding why this happens, and how to adapt our code to stay up-to-date and maintain smooth functionality.
Understanding Deprecated Desired Capabilities
As the Selenium library evolves, certain capabilities that were previously used might become obsolete or less relevant due to advancements in browser technology or changes in Selenium’s architecture. When a capability is marked as deprecated, it means that while it still works for the time being, it’s advised to transition away from using it. Ignoring deprecation messages can lead to compatibility issues and unreliable test runs.
Why Do Capabilities Get Deprecated?
The deprecation of a capability can occur due to various reasons, such as:
- Obsolete Technologies: As browsers and the web evolve, certain technology (e.g., Flash) becomes obsolete, rendering related capabilities unnecessary.
- Security Concerns: Certain capabilities might become deprecated due to security vulnerabilities associated with them.
- Selenium Updates: With newer versions, Selenium might introduce more efficient ways of achieving the same functionality, making older capabilities redundant.
How to Handle Deprecated Desired Capabilities
When you encounter a deprecated capability, the ideal approach is to find the modern alternative and update your code accordingly. Here’s a step-by-step guide to handling deprecated capabilities in your Selenium tests:
1. Check Selenium Documentation
Start by referring to the official Selenium documentation for the version you are using. The documentation typically provides details about deprecated capabilities and suggests alternatives.
2. Use Modern Options
Replace deprecated capabilities with their modern counterparts. For instance, if the PLATFORM
capability is deprecated, switch to using the PLATFORM_NAME
capability.
3. Keep Selenium Updated
Regularly update your Selenium dependencies to leverage the latest features and to ensure compatibility with recent browser versions.
4. Run Comprehensive Tests
After making the necessary code changes, run comprehensive tests to ensure that the updated capabilities work as expected across different scenarios and browsers.
5. Refactor Existing Code
Refactor existing test code to eliminate the use of deprecated capabilities, keeping your codebase clean and future-proof.
Handling Deprecated Desired Capabilities - Practical Example
Let’s consider an example where the VERSION
capability, which was previously used to specify the browser version, is deprecated. In the latest Selenium version, the recommended approach is to utilize the browserVersion
capability.
// Previous code using deprecated VERSION capability
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
capabilities.setCapability(CapabilityType.VERSION, "latest");
// Updated code using modern browserVersion capability
ChromeOptions options = new ChromeOptions();
options.setCapability("browserVersion", "latest");
In this example, the deprecated VERSION
capability is replaced with the modern browserVersion
capability, ensuring our test code remains compatible with the latest Selenium version.
My Closing Thoughts on the Matter
In the fast-paced world of web development and browser technologies, it’s crucial to stay updated with the latest changes in Selenium and adapt our testing code accordingly. By understanding why certain Desired Capabilities get deprecated, being proactive in making the necessary adjustments, and running thorough tests, we can ensure that our Selenium tests remain robust and reliable.
Adapting to changes is a fundamental aspect of software development, and handling deprecated Desired Capabilities in Selenium testing is no exception. By following the best practices mentioned in this post, you can effectively manage deprecated capabilities and maintain the effectiveness of your Selenium test suite.
Remember, staying informed about deprecation announcements and regularly updating your testing framework and codebase are instrumental in staying ahead of potential compatibility issues.
By keeping our testing code up-to-date, we ensure that it aligns with the latest browser technology, thus improving the reliability and effectiveness of our automated tests.
So, embrace change, stay informed, and keep your Selenium tests at their prime!
To learn more about Selenium and browser automation, you can explore the official Selenium website and the Selenium GitHub repository.