Building a RESTful JDBC HTTP server with JOOQ
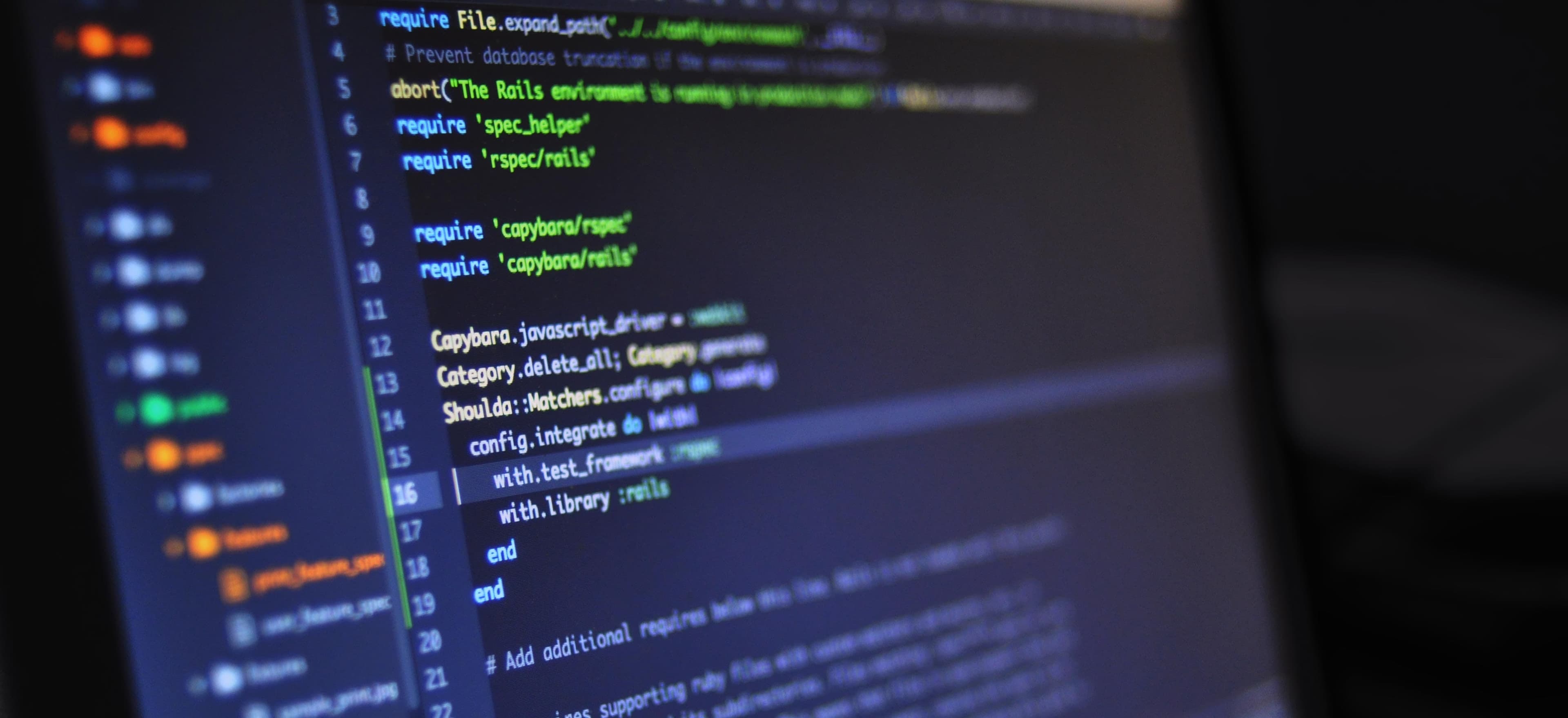
- Published on
Building a RESTful JDBC HTTP server with JOOQ
In this blog, we will explore how to create a RESTful HTTP server using Java, JDBC, and JOOQ. We will walk through building a simple CRUD (Create, Read, Update, Delete) API for a hypothetical users
table in a database.
The Approach to JOOQ
JOOQ (Java Object Oriented Querying) is a powerful library that allows for type-safe SQL query construction in Java. It provides a DSL that closely resembles SQL syntax while leveraging the strong typing of Java.
Setting up the Project
To get started, let's initialize a new Maven project and add the necessary dependencies. We'll need JOOQ, an HTTP server library such as SparkJava, and a JDBC driver for our database. Once the project is set up, we can define our database schema and generate JOOQ classes.
Creating the Database Schema
Assuming we have a PostgreSQL database, let's create a users
table with columns id
, username
, and email
. With JOOQ, we can define the schema using its code generation tool, which will generate corresponding Java classes representing the database schema.
Generating JOOQ Classes
After defining the database schema, we can use the JOOQ code generation tool to generate Java classes that represent the tables and records in the database. These generated classes will provide type-safe access to the database and help prevent runtime errors.
Setting Up the HTTP Server
Next, we'll set up our RESTful HTTP server using SparkJava. We'll define endpoints for creating, reading, updating, and deleting user records. When handling HTTP requests, we'll interact with the database using JOOQ's generated classes to perform CRUD operations.
// Define a route for retrieving a user by ID
get("/users/:id", (req, res) -> {
int userId = Integer.parseInt(req.params("id"));
UserRecord user = DSL.using(configuration)
.selectFrom(USERS)
.where(USERS.ID.eq(userId))
.fetchOne();
if (user != null) {
return user.into(User.class);
} else {
res.status(404);
return "User not found";
}
}, new JsonTransformer());
// Define a route for creating a new user
post("/users", (req, res) -> {
User user = new Gson().fromJson(req.body(), User.class);
UserRecord newUser = DSL.using(configuration)
.insertInto(USERS, USERS.USERNAME, USERS.EMAIL)
.values(user.getUsername(), user.getEmail())
.returning()
.fetchOne();
res.status(201);
return newUser.into(User.class);
}, new JsonTransformer());
In the above code snippet, we define routes for retrieving a user by ID and creating a new user. We use JOOQ's generated classes to interact with the database and convert the database records to Java objects.
Implementing CRUD Operations
Now that our HTTP server is set up, we can implement the remaining CRUD operations - updating and deleting user records. We will use JOOQ's generated classes to perform these operations and handle the corresponding HTTP requests.
// Define a route for updating a user by ID
put("/users/:id", (req, res) -> {
int userId = Integer.parseInt(req.params("id"));
User user = new Gson().fromJson(req.body(), User.class);
int updated = DSL.using(configuration)
.update(USERS)
.set(USERS.USERNAME, user.getUsername())
.set(USERS.EMAIL, user.getEmail())
.where(USERS.ID.eq(userId))
.execute();
if (updated > 0) {
return "User updated successfully";
} else {
res.status(404);
return "User not found";
}
});
// Define a route for deleting a user by ID
delete("/users/:id", (req, res) -> {
int userId = Integer.parseInt(req.params("id"));
int deleted = DSL.using(configuration)
.deleteFrom(USERS)
.where(USERS.ID.eq(userId))
.execute();
if (deleted > 0) {
return "User deleted successfully";
} else {
res.status(404);
return "User not found";
}
});
In the above code snippet, we implement routes for updating and deleting user records. We again use JOOQ's generated classes to interact with the database and handle the corresponding HTTP requests.
Final Considerations
In this blog post, we explored how to build a RESTful HTTP server using Java, JDBC, and JOOQ. We set up a simple CRUD API for a users
table in a database, leveraging JOOQ's type-safe SQL query construction and interactions with the database.
By following this example, you can extend this approach to build more complex APIs and leverage JOOQ's powerful features for working with databases in a type-safe and efficient manner.
For more information on JOOQ, JDBC, and RESTful API development in Java, check out the following resources:
Checkout our other articles