Maximizing Efficiency: Navigating Case Tools
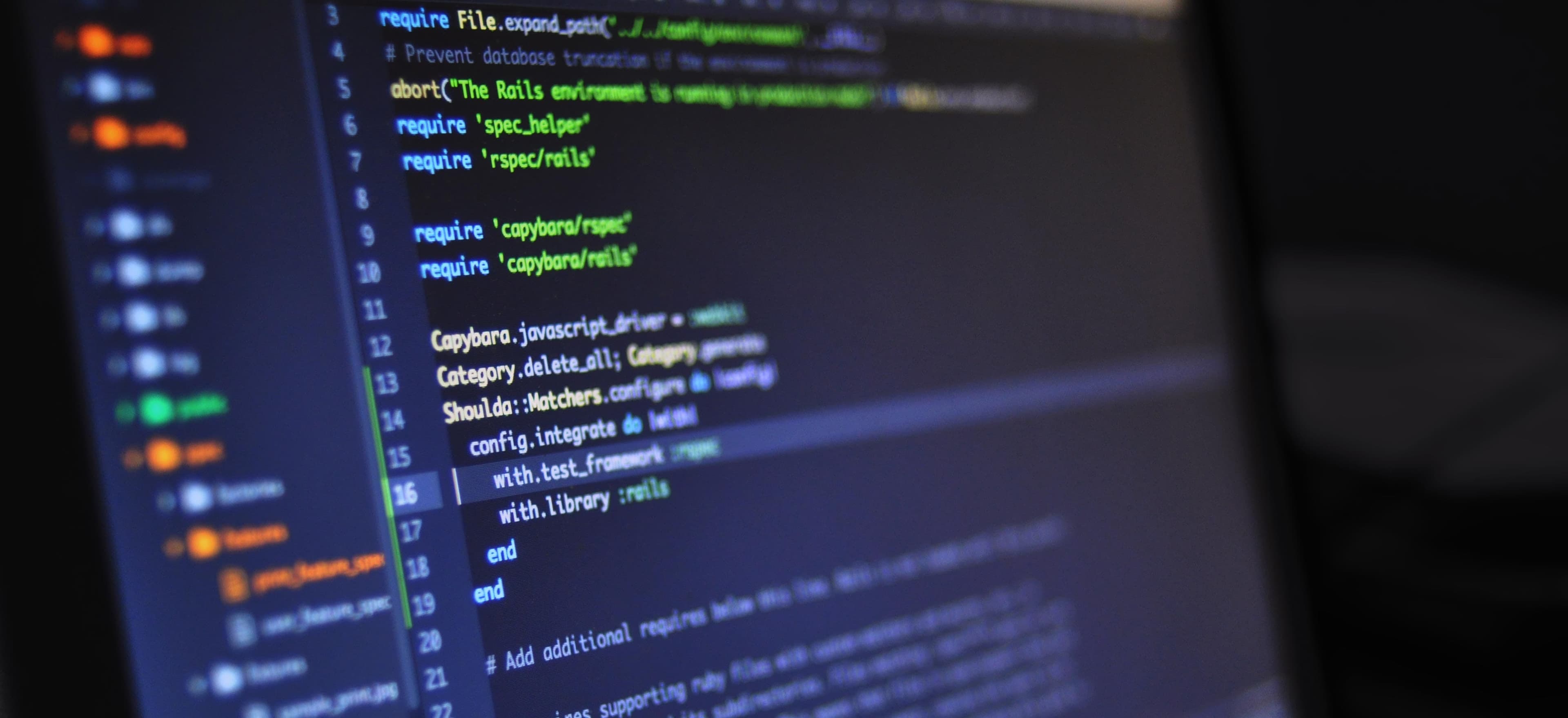
- Published on
Maximizing Efficiency: Navigating Case Tools
In the world of Java development, the choice of Case tools can significantly impact the efficiency and quality of your code. Case tools, short for Computer-Aided Software Engineering tools, aid developers in designing, analyzing, and implementing software systems. These tools enable visual modeling, automated code generation, and database design, among other functionalities, streamlining the development process and enhancing productivity.
In this article, we will delve into the significance of Case tools in Java development, explore the essential features to consider when selecting a Case tool, and provide insights into leveraging these tools to maximize efficiency and productivity in Java development.
Importance of Case Tools in Java Development
Java, being a versatile and widely-used programming language, demands robust and efficient development practices. Case tools play a pivotal role in Java development, offering functionalities that facilitate the entire software development lifecycle, from initial design to implementation and maintenance.
Visual Modeling
One of the primary advantages of Case tools is their support for visual modeling. Through visual representations such as UML (Unified Modeling Language) diagrams, developers can conceptualize and design the structure and behavior of software systems. This visual approach expedites the understanding of complex systems, aids in communication among developers, and serves as a blueprint for implementing the Java code.
Code Generation
Case tools provide the capability to generate code automatically based on the visual models created. This feature significantly reduces the time and effort required for writing repetitive or boilerplate code, while also ensuring consistency between the design and implementation phases.
Database Design
Efficient handling of data is fundamental in Java development. Many Case tools offer features for designing and managing databases, including entity-relationship diagrams and the generation of database scripts, fostering seamless integration between the Java application and its data storage.
Essential Features of Case Tools for Java Development
When evaluating Case tools for Java development, several key features and capabilities should be considered to ensure they align with the requirements and goals of the development projects.
UML Support
Unified Modeling Language (UML) is a standard visual language for expressing the design of a software system. Case tools with comprehensive support for UML enable the creation of class diagrams, sequence diagrams, activity diagrams, and other UML diagrams, empowering developers to model their Java applications effectively.
Code Generation Customization
The ability to customize code generation templates and rules is crucial for adapting the generated code to specific coding standards, architectural patterns, and project conventions. This feature ensures that the generated Java code conforms to the established guidelines and best practices.
Integration with IDEs
Seamless integration with popular Integrated Development Environments (IDEs) such as IntelliJ IDEA, Eclipse, or NetBeans enhances the developer experience by allowing the Case tool to harmoniously coexist with the preferred development environment, enabling a smooth transition between modeling and coding phases.
Collaboration and Version Control
Collaboration features, including the ability to concurrently work on models, track changes, and integrate with version control systems like Git, are essential for team-based Java development. This fosters collaboration, ensures versioning of models, and facilitates the management of changes within the development team.
Database Management
For Java applications that interact with databases, robust database design and management capabilities within the Case tool are invaluable. The tool should support the visual design of database schemas, generate DDL (Data Definition Language) scripts, and offer synchronization with the evolving application data model.
Leveraging Case Tools for Maximum Efficiency in Java Development
Once a suitable Case tool is selected, maximizing its potential to enhance efficiency and productivity in Java development requires deliberate utilization of its features and integration into the development workflow.
Visual Modeling for Design Clarity
When initiating a Java project, leveraging visual modeling capabilities to create UML diagrams provides a clear and structured depiction of the system's architecture and behavior. By visually mapping out classes, associations, and interactions, the development team gains a shared understanding of the system, aiding in making informed design decisions.
// Example of a simple UML class diagram in Java
public class Order {
private int orderId;
private Date orderDate;
private List<Item> items;
public void addItems(Item item) {
// Add item to the order
}
// Getters and setters
}
In the above code snippet, visualizing the Order
class and its attributes as well as relationships with other classes in a UML class diagram would provide a clearer overview of the system's structure.
Automated Code Generation for Efficiency
By utilizing the code generation capabilities of the Case tool, developers can automate the generation of Java classes, interfaces, and methods based on the defined models. This automation minimizes manual coding efforts, reduces the likelihood of errors, and ensures consistency between the design and implementation artifacts.
// Example of generated Java code for the Order class
public class Order {
private int orderId;
private Date orderDate;
private List<Item> items;
public void addItems(Item item) {
// Add item to the order
}
// Getters and setters
}
The generated Java code aligns with the UML model, eliminating the need for manual implementation and preserving the integrity of the design.
Database Design Synchronization
In scenarios where the Java application interacts with a database, maintaining synchronization between the application's data model and the database schema is critical. Leveraging the Case tool's database design features ensures that changes in the application's data model are seamlessly reflected in the database schema, thereby reducing inconsistencies and simplifying data management.
-- Example of a generated database script for creating the Order table
CREATE TABLE Order (
orderId INT PRIMARY KEY,
orderDate DATE,
...
);
The generated DDL script aligns with the defined data model, demonstrating the synchronization between the application's data representation and the database schema.
Collaboration and Version Control Integration
In a team setting, exploiting the collaboration and version control capabilities of the Case tool fosters seamless teamwork, concurrent modeling, change tracking, and the ability to merge and reconcile model modifications. Integrating with version control systems ensures the traceability of changes and facilitates collaborative development.
Integration with IDEs for Streamlined Workflow
Integrating the selected Case tool with the preferred IDE ensures a cohesive development experience, allowing developers to seamlessly transition between modeling and coding tasks within a familiar environment. This integration reduces context switching overhead and promotes a fluid development workflow.
Lessons Learned
In the realm of Java development, Case tools serve as invaluable assets for enhancing efficiency, maintaining design integrity, and enabling systematic development practices. By embracing the visual modeling, code generation, database design, collaboration, and integration capabilities offered by Case tools, Java developers can navigate the intricate landscape of software engineering with confidence and agility.
Selecting a Case tool that encompasses the essential features tailored to Java development requirements and adeptly leveraging its functionalities are paramount to maximizing efficiency and productivity. As Java continues to evolve and diversify, the judicious adoption of Case tools will remain integral to the pursuit of excellence in software development.
Incorporating Case tools into the Java development workflow empowers developers to transcend the constraints of traditional coding approaches, fostering a more streamlined, collaborative, and visually driven development environment.
Remember, the right Case tool is not just a companion—it's a catalyst for innovation and efficiency in your Java development journey!
References:
- What are CASE tools?
- Top 5 UML tools for Java developers
- UML Class Diagrams Tutorial
- IntelliJ IDEA
- Eclipse IDE
- NetBeans IDE