Troubleshooting Knative Serving with Ambassador Gateway
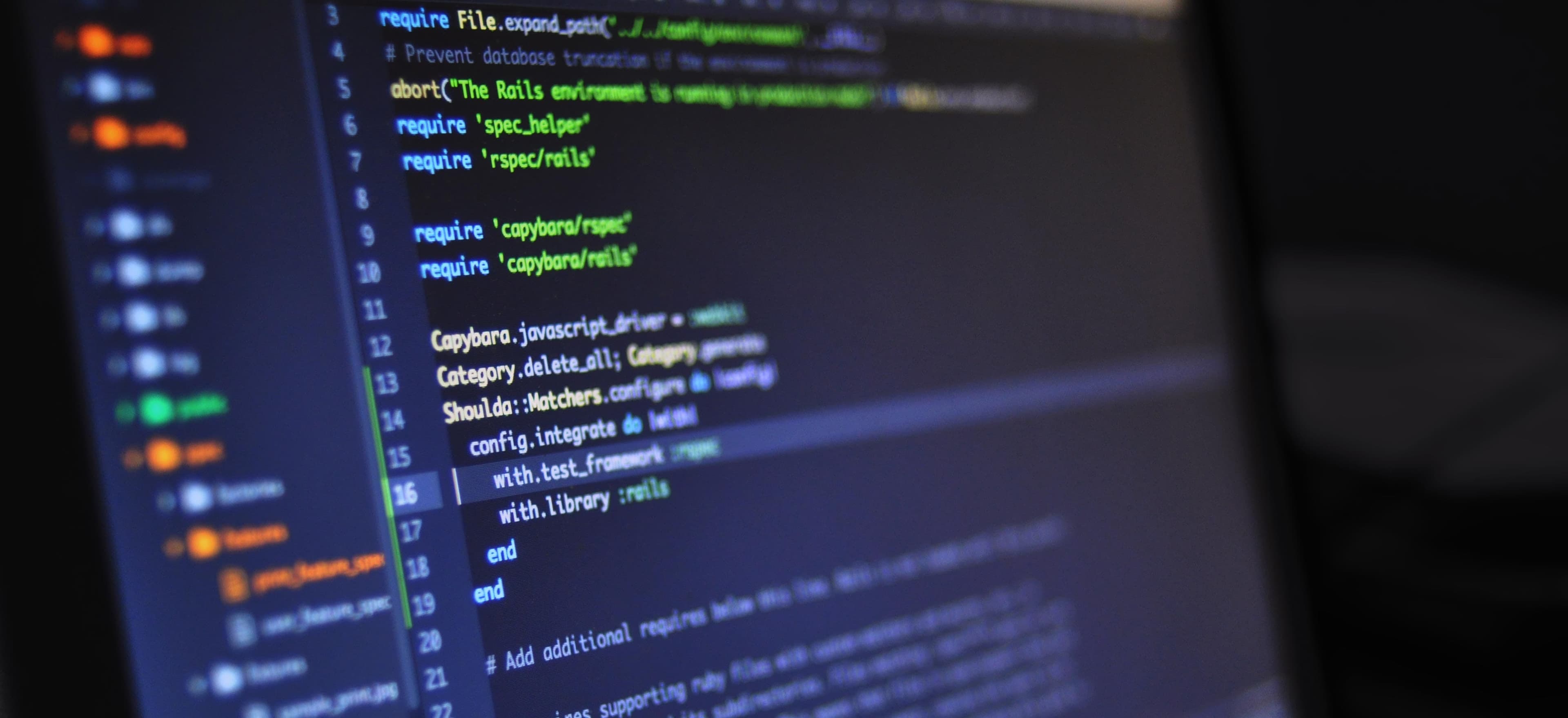
- Published on
Troubleshooting Knative Serving with Ambassador Gateway
Knative Serving and Ambassador Gateway are powerful tools for building and managing serverless applications on Kubernetes. However, like any complex technology, issues can arise when working with these tools. In this post, we'll explore some common troubleshooting steps when using Knative Serving with Ambassador Gateway, and how to resolve them using Java.
Understanding Knative Serving and Ambassador Gateway
Knative Serving is a Kubernetes-based platform for deploying, serving, and managing serverless workloads. It provides a set of middleware components that are essential to build modern, source-centric, and container-based applications.
On the other hand, Ambassador Gateway is a Kubernetes-native API Gateway built on the Envoy Proxy. It provides a powerful, yet easy-to-use control plane for managing ingress traffic to your applications. Ambassador can be configured to work seamlessly with Knative Serving to manage traffic to serverless applications.
Identifying Common Issues
Issue 1: Knative Service Not Accessible via Ambassador Gateway
Possible Cause
The Knative service may not be properly exposed through the Ambassador Gateway due to misconfigured networking or incorrect resource configurations in Knative and Ambassador.
Solution
To resolve this issue, first ensure that the Knative service is properly configured to expose the necessary routes and traffic can reach the Ambassador Gateway. Verify that the Knative service is using the correct network configuration and is targeting the Ambassador Gateway endpoint. Additionally, double-check the Ambassador Gateway configuration to ensure that it correctly forwards traffic to the Knative service.
Issue 2: Degraded Performance of Java Applications
Possible Cause
Java applications running within Knative Serving may experience degraded performance due to incorrect resource allocations, JVM tuning, or inefficient code.
Solution
To address performance issues with Java applications, it's important to properly configure the resource limits and requests for the Knative service. Additionally, tuning the JVM parameters, such as heap size and garbage collection settings, can significantly improve the performance of Java applications. Profiling and optimizing the Java code itself can also lead to performance improvements.
Resolving Issues with Java
Example: Configuring Knative Service for Ambassador Gateway
import io.kubernetes.client.openapi.ApiClient;
import io.kubernetes.client.openapi.apis.NetworkingV1Api;
import io.kubernetes.client.openapi.models.V1beta1Ingress;
import io.kubernetes.client.openapi.models.V1beta1IngressRule;
public class KnativeAmbassadorConfig {
public void configureKnativeServiceForAmbassador(String serviceName, String ambassadorGateway) {
ApiClient client = Configuration.getDefaultApiClient();
NetworkingV1Api api = new NetworkingV1Api(client);
V1beta1Ingress ingress = new V1beta1Ingress();
V1beta1IngressRule rule = new V1beta1IngressRule();
// Configure Ingress rule for Ambassador Gateway
rule.setHost(ambassadorGateway);
// Set paths and other configurations as needed
ingress.addRulesItem(rule);
api.createNamespacedIngress("default", ingress, null, null, null);
}
}
In this Java example, we demonstrate how to configure a Knative service to expose routes through an Ambassador Gateway. By using the Kubernetes client library for Java, we interact with the Kubernetes API to create an Ingress resource that specifies the routing rules for the Ambassador Gateway.
Example: JVM Tuning for Improved Performance
public class JavaAppPerformanceTuning {
public static void main(String[] args) {
// JVM tuning for better performance
// Example: Set heap size to 1GB
// -Xms1g -Xmx1g
// Example: Enable G1 garbage collector
// -XX:+UseG1GC
}
}
When dealing with degraded performance of Java applications within Knative Serving, tuning the JVM parameters is crucial. In this example, we showcase how JVM parameters can be set when running a Java application. By configuring the heap size and garbage collector, the performance of the Java application can be optimized for the Knative Serving environment.
Wrapping Up
Troubleshooting Knative Serving with Ambassador Gateway often requires a deep understanding of both technologies, as well as the underlying infrastructure. By identifying common issues and leveraging Java for resolving them, developers can ensure the smooth operation of serverless applications on Kubernetes. With the right tools and techniques, Knative Serving and Ambassador Gateway can provide a reliable and efficient platform for running Java applications in a serverless environment.
For more in-depth information about Knative Serving and Ambassador Gateway, you can refer to the official documentation:
In conclusion, effectively troubleshooting Knative Serving with Ambassador Gateway involves a combination of understanding, analysis, and proactive Java programming. By addressing the nuances of these technologies through Java, developers can overcome challenges and optimize their serverless applications for peak performance.