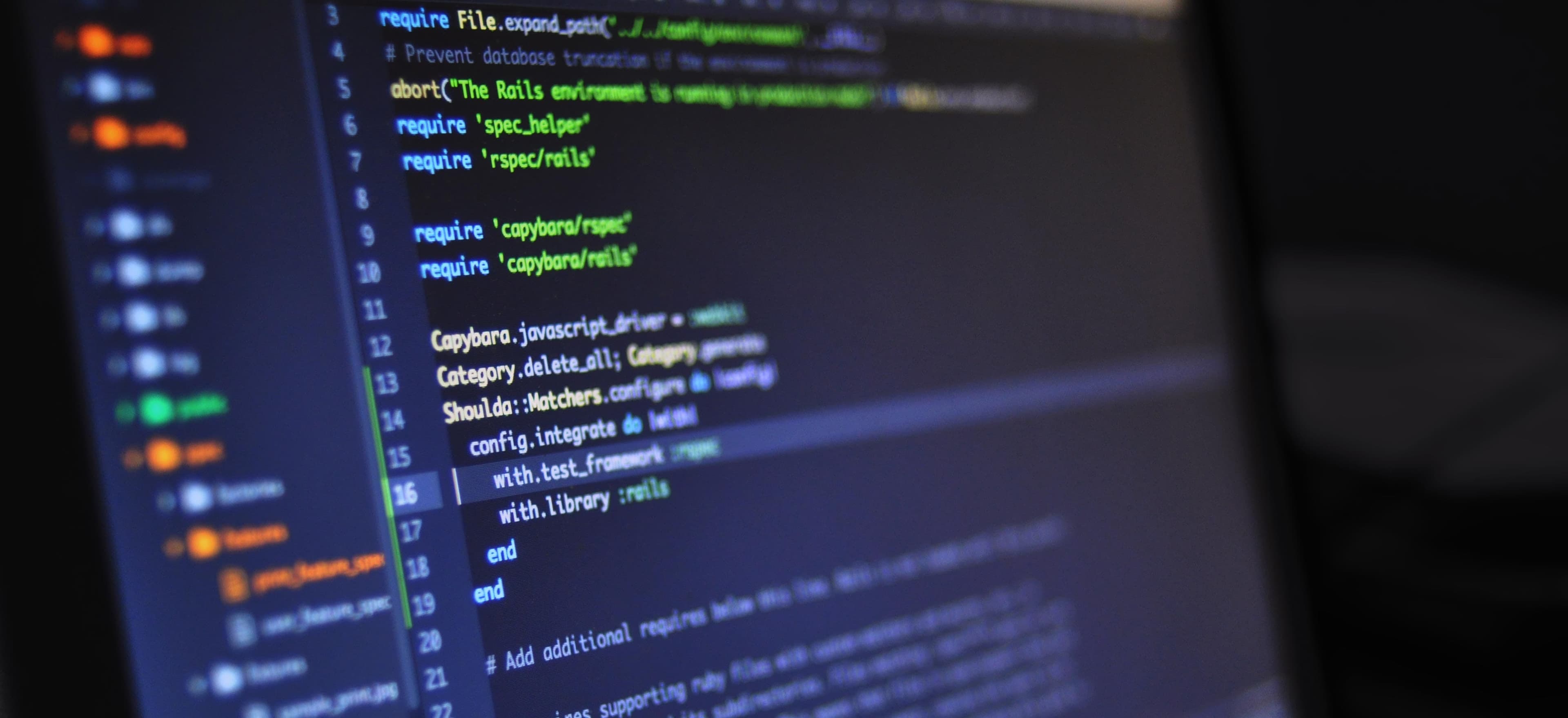
- Published on
Effective Keyboard Actions for Selenium Testing
In Selenium testing, user interactions with web elements are crucial for simulating real user behavior. This includes keyboard actions such as typing into input fields, pressing keys, and performing keyboard shortcuts. In this blog post, we will explore the effective use of keyboard actions in Selenium testing using the Java programming language.
Setting Up Selenium WebDriver
Before we delve into keyboard actions, let's ensure we have the necessary setup for Selenium WebDriver in Java. First, you need to have the Selenium WebDriver library in your project. You can either download the JAR file or use a build automation tool like Maven to manage dependencies.
// Maven dependency for Selenium WebDriver
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependency>
Now, let's set up a WebDriver instance for your chosen browser:
// Setting up WebDriver for Chrome
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
System.setProperty("webdriver.chrome.driver", "path_to_chromedriver_executable");
WebDriver driver = new ChromeDriver();
With the WebDriver set up, we can move on to performing keyboard actions.
Typing into Input Fields
Typing into input fields is one of the most common keyboard actions in Selenium testing. We can achieve this using the sendKeys()
method provided by Selenium.
// Locating an input field by its ID and typing into it
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
WebElement inputField = driver.findElement(By.id("username"));
inputField.sendKeys("example_username");
Here, we located the input field using its ID and then used the sendKeys()
method to type "example_username" into the field.
Pressing Keys
Sometimes, we need to simulate key presses such as Enter, Escape, or Arrow keys. Selenium provides the Keys
class for sending special keys.
// Simulating pressing the Enter key after filling a form
import org.openqa.selenium.Keys;
inputField.sendKeys("example_text");
inputField.sendKeys(Keys.ENTER);
In this example, after typing "example_text" into the input field, we simulate pressing the Enter key using Keys.ENTER
.
Performing Keyboard Shortcuts
Keyboard shortcuts can also be tested using Selenium. For example, to simulate the Ctrl+A (select all) shortcut, we can use the Actions
class.
// Simulating Ctrl+A (select all) shortcut
import org.openqa.selenium.interactions.Actions;
Actions actions = new Actions(driver);
actions.keyDown(Keys.CONTROL).sendKeys("a").keyUp(Keys.CONTROL).perform();
Here, we used the Actions
class to perform the keyboard shortcut for select all (Ctrl+A) by chaining the keyDown()
, sendKeys()
, and keyUp()
methods.
Handling Modifiers Keys
Modifier keys such as Ctrl, Shift, and Alt can be handled in combination with other keys.
// Simulating Ctrl + Click on a web element
Actions actions = new Actions(driver);
actions.keyDown(Keys.CONTROL).click(someElement).keyUp(Keys.CONTROL).perform();
In this example, we used the keyDown()
method to simulate pressing the Ctrl key, then performed a click on a web element, and finally released the Ctrl key using the keyUp()
method.
Closing the Chapter
In Selenium testing with Java, keyboard actions are essential for simulating user interactions with web elements. Whether it's typing into input fields, pressing keys, or performing keyboard shortcuts, Selenium provides robust capabilities for handling these actions. By leveraging the examples and techniques discussed in this blog post, you can effectively incorporate keyboard actions into your Selenium test automation to ensure comprehensive coverage of user interactions.
By mastering keyboard actions in Selenium, you can elevate the quality and reliability of your automated tests, leading to more robust web applications and enhanced user experiences.
For further information on Selenium WebDriver and Java, refer to the official Selenium documentation.
Checkout our other articles