Cracking the Code: Creating an Unambiguous Software Versioning Scheme
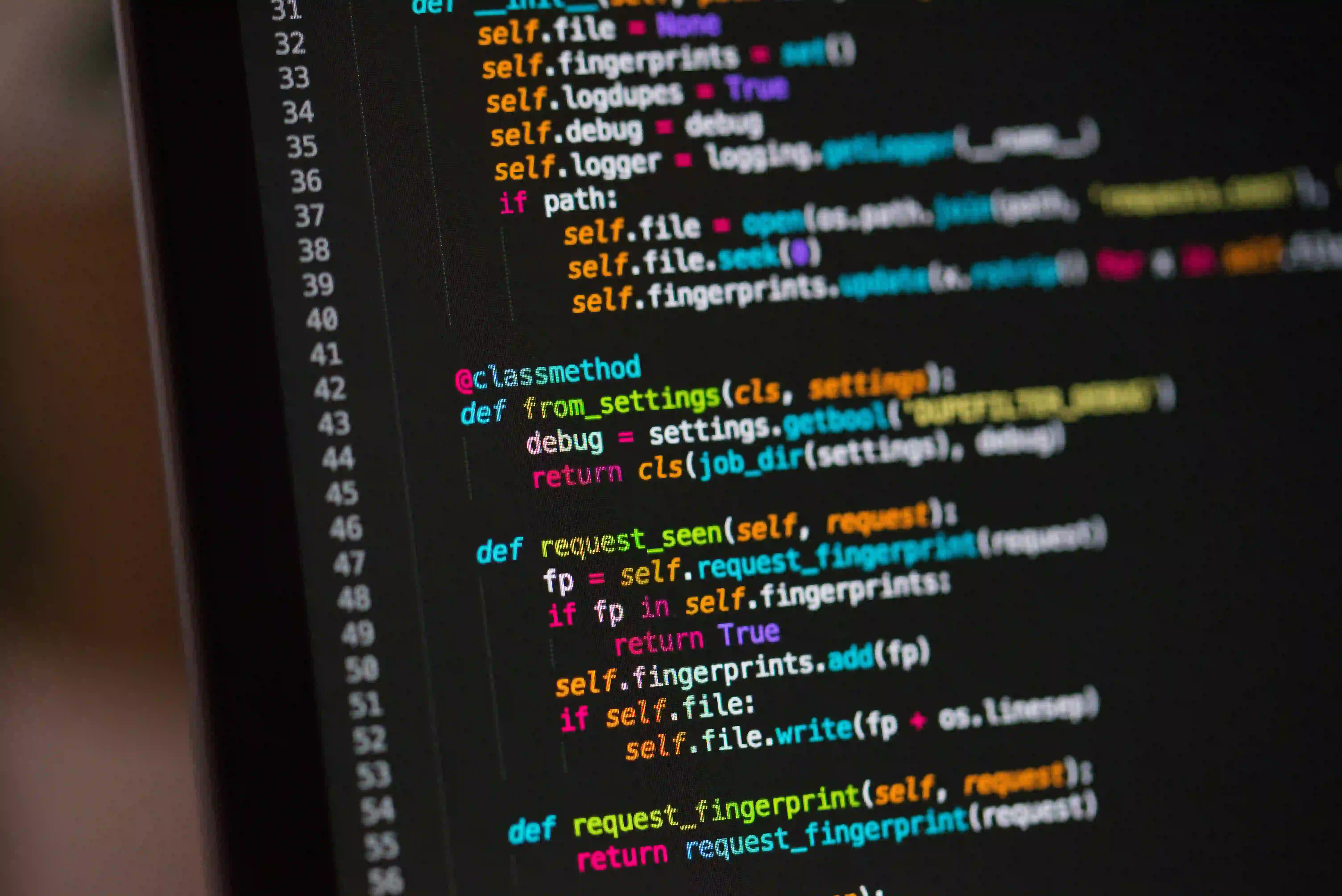
Decoding Software Versioning Scheme in Java
Software versioning plays a crucial role in managing and tracking the progression of software products. An unambiguous versioning scheme is essential to ensure seamless collaboration between developers and efficient deployment of software. In this blog post, we'll delve into the intricacies of creating a clear and effective software versioning scheme using Java, and explore best practices to maintain consistency and clarity in version numbering.
Why Software Versioning Matters
A well-defined versioning scheme serves as a communication tool, conveying significant information about the software's evolution. It allows developers, users, and automated systems to understand the stage of development, the presence of new features, and the resolution of issues through each version release. A carefully constructed versioning scheme also aids in providing clear release notes and in ensuring smooth upgrades for end-users.
Importance of Semantic Versioning
Before diving into the implementation, it's important to understand the concept of Semantic Versioning (SemVer). SemVer provides a standard for version numbers that convey meaning, making it easy to understand the impact of changes in a given release. The version number is divided into three parts: MAJOR.MINOR.PATCH. Incrementing each part conveys specific information about the changes made:
- MAJOR version: Incremented when making incompatible API changes.
- MINOR version: Incremented when adding new functionality in a backward-compatible manner.
- PATCH version: Incremented when making backward-compatible bug fixes.
Implementing Semantic Versioning in Java
Java, being a versatile and widely used programming language, offers robust support for implementing Semantic Versioning. By following best practices and leveraging the inherent features of Java, we can create a coherent and transparent versioning scheme.
Using Enums for Version Components
public enum VersionComponent {
MAJOR, MINOR, PATCH;
}
In this snippet, we define an enum VersionComponent
representing the three components of a semantic version - MAJOR, MINOR, and PATCH. This enumeration provides an explicit and meaningful way to represent the version components, making the code more readable and maintainable.
Designing the Version Class
public class Version {
private int major;
private int minor;
private int patch;
// Constructor and methods for version manipulation
}
In the Version
class, we encapsulate the version components and provide methods to manipulate the version according to the rules of Semantic Versioning. Encapsulation ensures that the version components are modified in a controlled manner, maintaining the integrity of the version number.
Applying SemVer Rules for Version Increment
public class Version {
// Existing code
public void increment(VersionComponent component) {
switch (component) {
case MAJOR:
major++;
minor = 0;
patch = 0;
break;
case MINOR:
minor++;
patch = 0;
break;
case PATCH:
patch++;
break;
default:
throw new IllegalArgumentException("Invalid version component");
}
}
}
In the Version
class, the increment
method increments the specified version component following the SemVer rules. This ensures that version increments align with the intended impact of the changes, allowing for consistent and understandable version progression.
Managing Pre-release and Metadata
In some cases, software versions may also include pre-release identifiers (such as alpha, beta, rc) and build metadata. These additions provide further context about the version, aiding in testing and tracking specific builds. Incorporating support for pre-release and metadata in the versioning scheme enhances its comprehensiveness.
Extending the Version Class for Pre-release and Metadata
public class Version {
// Existing code
private String preRelease;
private String metadata;
// Constructors, accessors, and methods for pre-release and metadata handling
}
By extending the Version
class to accommodate pre-release and metadata, we ensure the flexibility and inclusivity of the versioning scheme. This allows for precise identification and categorization of different builds, helping in effectively managing and communicating the status of pre-release versions.
Ensuring Compatibility and Usage
While creating a clear versioning scheme is essential, ensuring its adoption and understanding among the development team and stakeholders is equally crucial. Proper documentation, training, and integration of versioning practices into the development workflow can promote consistent usage and alignment with the intended SemVer guidelines.
Documenting Versioning Practices
Documenting the versioning practices in a project's README or developer documentation ensures that all contributors are aware of the established versioning guidelines. Additionally, providing examples and explanations regarding version increments and pre-release usage can facilitate seamless adoption and adherence to the versioning scheme.
Integration with Build and Release Process
Integrating the versioning scheme with the build and release process, such as incorporating version increment scripts in the continuous integration pipeline, automates version updates and maintains consistency across builds. This reduces the likelihood of human error in version management, ensuring that the versioning scheme is diligently followed.
Bringing It All Together
A well-crafted versioning scheme serves as a linchpin in software development, facilitating clarity, consistency, and collaboration. By embracing Semantic Versioning principles and leveraging Java's capabilities, we can construct an unambiguous and intuitive versioning scheme that elucidates the evolution of software releases. The adoption of such a scheme fosters transparency, streamlines communication, and engenders confidence in both development teams and end-users, ensuring a smooth and informed software evolution.
Incorporating Semantic Versioning guidelines and implementing a well-defined versioning scheme using Java empowers software projects with a robust framework for version management, enabling them to convey meaningful insights about each release and facilitate seamless collaboration in the software development lifecycle. With a clear and consistent versioning scheme in place, software projects can navigate the ever-evolving landscape of development with enhanced coherence and comprehension.
Software versioning may seem like a small detail, but its impact on collaboration, deployment, and user experience cannot be overstated. By embracing Semantic Versioning and implementing a clear versioning scheme in Java, software projects can sow the seeds of transparency and compatibility, reaping the rewards of efficient communication and streamlined progression.