Troubleshooting Transient Attribute Activation
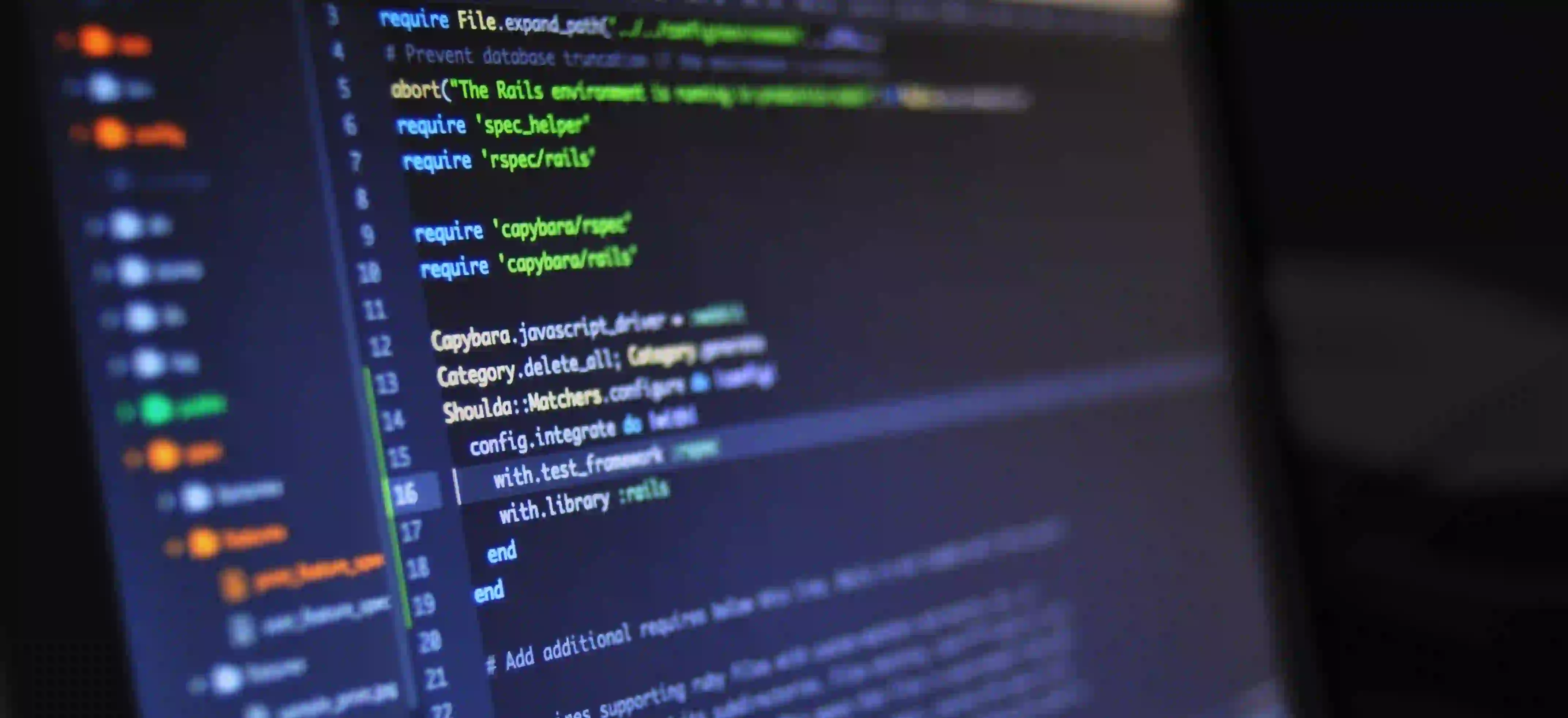
Troubleshooting Transient Attribute Activation in Java
Java, as a widely used programming language, offers various features. Among these, transient attributes play a crucial role in defining object serialization behavior. However, developers often encounter activation issues when working with transient attributes. In this blog post, we will explore the concept of transient attributes, the common problems associated with their activation, and how to troubleshoot these issues effectively.
Understanding Transient Attributes
In Java, the transient
keyword is used to indicate that a field should not be serialized when the object containing that field is passed across a network or converted into a persistent store. This keyword prevents the inclusion of specific fields in the serialization process, providing control over which parts of an object need to be serialized.
When an object with transient attributes is serialized, the transient fields' values are not persisted. Upon deserialization, transient attributes are initialized to their default values (e.g., null
for objects, 0
for numeric types, and false
for booleans).
Common Activation Issues with Transient Attributes
Despite the straightforward concept of transient attributes, developers often face challenges related to their activation. The following are some common issues encountered when working with transient attributes in Java:
1. Inconsistent Values after Deserialization
In certain scenarios, developers observe that the transient fields have inconsistent or unexpected values after deserialization. This can occur due to improper handling of transient attributes during the serialization and deserialization process.
2. Data Loss
Improper activation of transient attributes can lead to data loss during the serialization and deserialization of objects. This can result in missing values for transient fields or incorrect initialization upon deserialization.
3. Compatibility Issues
Changes to the transient attributes of a class can lead to compatibility issues when attempting to deserialize objects that were serialized using the previous version of the class. Incorrect activation of transient attributes can break the deserialization process, causing compatibility problems.
Troubleshooting Transient Attribute Activation
To effectively troubleshoot transient attribute activation issues, developers can follow the best practices outlined below:
1. Review Serialization and Deserialization Process
Thoroughly review the serialization and deserialization process to ensure that transient attributes are being handled correctly. Verify that the transient fields are excluded from the serialization process and appropriately initialized during deserialization.
private transient String transientField;
In the code above, the transient
keyword excludes the transientField
from the serialization process.
2. Check Object Versioning
When making changes to transient attributes, it is essential to consider object versioning to maintain compatibility during serialization and deserialization. Utilize techniques such as custom serialization to handle versioning and gracefully handle changes to transient attributes.
3. Utilize Serialization Callbacks
Java provides serialization callbacks through the readObject()
and writeObject()
methods to perform custom processing during the serialization and deserialization of objects. Utilize these callbacks to ensure proper activation of transient attributes based on specific requirements.
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
// Additional processing for transient attributes
}
By implementing the readObject()
method, developers can customize the activation of transient attributes during deserialization.
4. Log and Debug Serialization Process
Logging and debugging the serialization process can provide insights into how transient attributes are being handled. This includes verifying the exclusion of transient fields during serialization and examining their initialization upon deserialization.
5. Unit Testing
Develop comprehensive unit tests to validate the serialization and deserialization behavior of objects with transient attributes. Ensure that the tests cover various scenarios to detect activation issues and potential data loss.
Bringing It All Together
In Java programming, understanding and effectively handling transient attribute activation is essential for maintaining data integrity and serialization compatibility. By following the best practices and troubleshooting techniques discussed in this post, developers can mitigate transient attribute activation issues and ensure the seamless serialization and deserialization of objects.
For further exploration, refer to the Java Object Serialization Specification to gain a deeper understanding of object serialization concepts in Java.
In conclusion, mastering transient attribute activation empowers developers to harness the full potential of Java's object serialization capabilities while maintaining control over data persistence and compatibility. Happy coding!
Remember, the key to successful troubleshooting is a combination of thorough understanding, strategic thinking, and meticulous attention to detail. With these tools in hand, transient attribute activation issues will become a thing of the past.