Managing Concurrent ActiveMQ Instances: Potential Issues
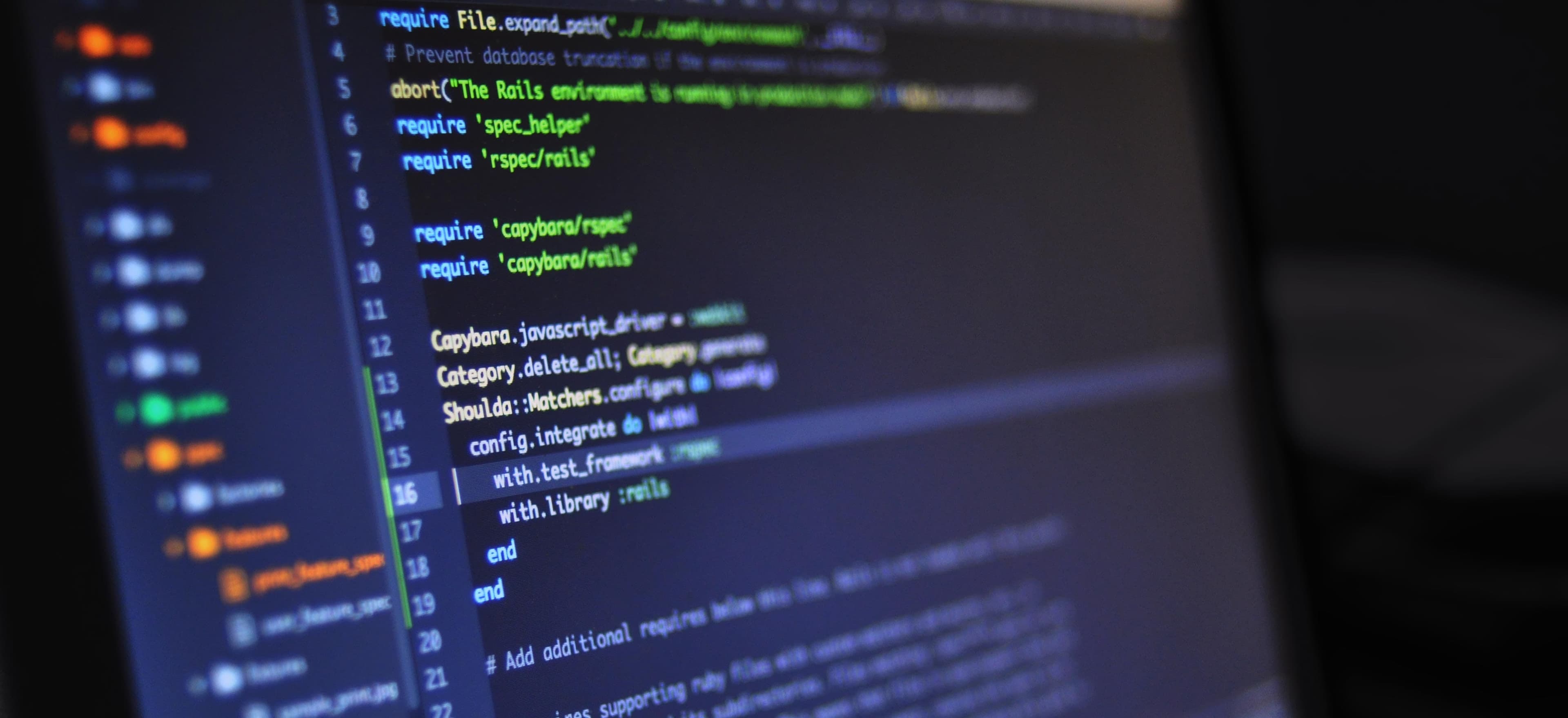
- Published on
Managing Concurrent ActiveMQ Instances: Potential Issues
ActiveMQ is a popular open-source message broker that is widely used for building integrations between applications. While it offers great flexibility and scalability, managing concurrent ActiveMQ instances can lead to potential issues that need to be carefully addressed. In this article, we'll explore some common problems that can arise when working with multiple ActiveMQ instances and discuss strategies to mitigate these issues.
The Challenge of Concurrent ActiveMQ Instances
When dealing with multiple ActiveMQ instances, several challenges can emerge, including:
-
Message Duplication: It's essential to ensure that messages are not duplicated across different instances, which can lead to data inconsistency and other problems.
-
Synchronization: Managing the synchronization of message consumption and processing across instances is crucial for ensuring the correct processing order and preventing race conditions.
-
Load Balancing: Balancing the message load across instances to ensure optimal utilization of resources while avoiding overloading any particular instance.
-
Failover and High Availability: Implementing failover mechanisms to ensure high availability in case of instance failures.
Let's delve into each of these challenges and discuss potential strategies to address them.
Message Duplication
When running multiple instances of ActiveMQ, preventing message duplication is a crucial concern. One way to address this is by utilizing unique message IDs and implementing message deduplication mechanisms. By leveraging features such as Message Groups in ActiveMQ, you can ensure that messages with the same message group ID are processed sequentially by a single consumer, thus avoiding duplication issues.
Exemplary Code Snippet
// Set the message group ID
message.setStringProperty("JMSXGroupID", "uniqueGroupId");
In the above code snippet, we set the JMSXGroupID
property of the message to a unique value, ensuring that messages with the same group ID are consumed sequentially by a single consumer.
Synchronization
Ensuring message consumption and processing synchronization across multiple instances is vital for maintaining data integrity and reliable message processing. One strategy to achieve this is by using distributed locking mechanisms, such as Apache ZooKeeper, to coordinate the message consumption process among the instances. By acquiring a distributed lock before processing a message, instances can ensure that only one of them processes a particular message at a time, avoiding concurrency issues.
Exemplary Code Snippet
// Acquire a distributed lock using Apache Curator
InterProcessMutex lock = new InterProcessMutex(curatorFramework, "/messageLockPath");
if (lock.acquire(10, TimeUnit.SECONDS)) {
try {
// Process the message
} finally {
lock.release();
}
}
In the code snippet above, we use Apache Curator to acquire a distributed lock, ensuring that only one instance processes a message at a time, thus synchronizing message processing across instances.
Load Balancing
Efficient load balancing is essential when working with multiple ActiveMQ instances to ensure optimal resource utilization and prevent overload conditions. ActiveMQ supports various load balancing strategies, including message group load balancing and message priority sorting. By appropriately configuring these strategies, you can distribute message load across instances based on predefined criteria, such as message group ID or message priority, thereby achieving a balanced message processing workload.
Exemplary Code Snippet
<!-- Configure message group load balancing -->
<destinationPolicy>
<policyMap>
<policyEntries>
<policyEntry queue="yourQueue" messageGroup="groupId" messageGroupType="comparable" />
</policyEntries>
</policyMap>
</destinationPolicy>
In the XML configuration above, we define a message group load balancing policy for a queue, ensuring that messages with the same group ID are distributed across instances in a balanced manner.
Failover and High Availability
Maintaining high availability when working with concurrent ActiveMQ instances involves implementing robust failover mechanisms. ActiveMQ provides built-in support for failover and clustering, allowing you to configure multiple instances to work together in a high-availability setup. By setting up a failover transport and configuring network connectors, you can ensure that if one instance fails, the others automatically take over the message processing, thus providing continuous service availability.
Exemplary Code Snippet
<!-- Configure failover transport -->
<transportConnectors>
<transportConnector name="openwire" uri="tcp://localhost:61616" updateClusterClients="true" rebalanceClusterClients="true" />
</transportConnectors>
<networkConnectors>
<networkConnector name="staticBridge" uri="static:(tcp://remote1:61616,tcp://remote2:61616)" duplex="true" networkTTL="3" decreaseNetworkConsumerPriority="true" dynamicOnly="true" />
</networkConnectors>
In the XML configuration above, we configure a failover transport and network connectors to enable failover and clustering among multiple ActiveMQ instances.
Closing Remarks
Managing concurrent ActiveMQ instances comes with its set of challenges, but with the right strategies and techniques, you can effectively mitigate potential issues and build a robust and scalable messaging infrastructure. By addressing concerns such as message duplication, synchronization, load balancing, and failover, you can ensure reliable and efficient message processing across multiple instances of ActiveMQ.
In this article, we've explored some common issues and provided actionable strategies to tackle them. By implementing these approaches and leveraging the capabilities of ActiveMQ, you can build and manage a resilient messaging system capable of handling concurrent instances seamlessly.