Avoiding the Parameterless Generic Method Antipattern
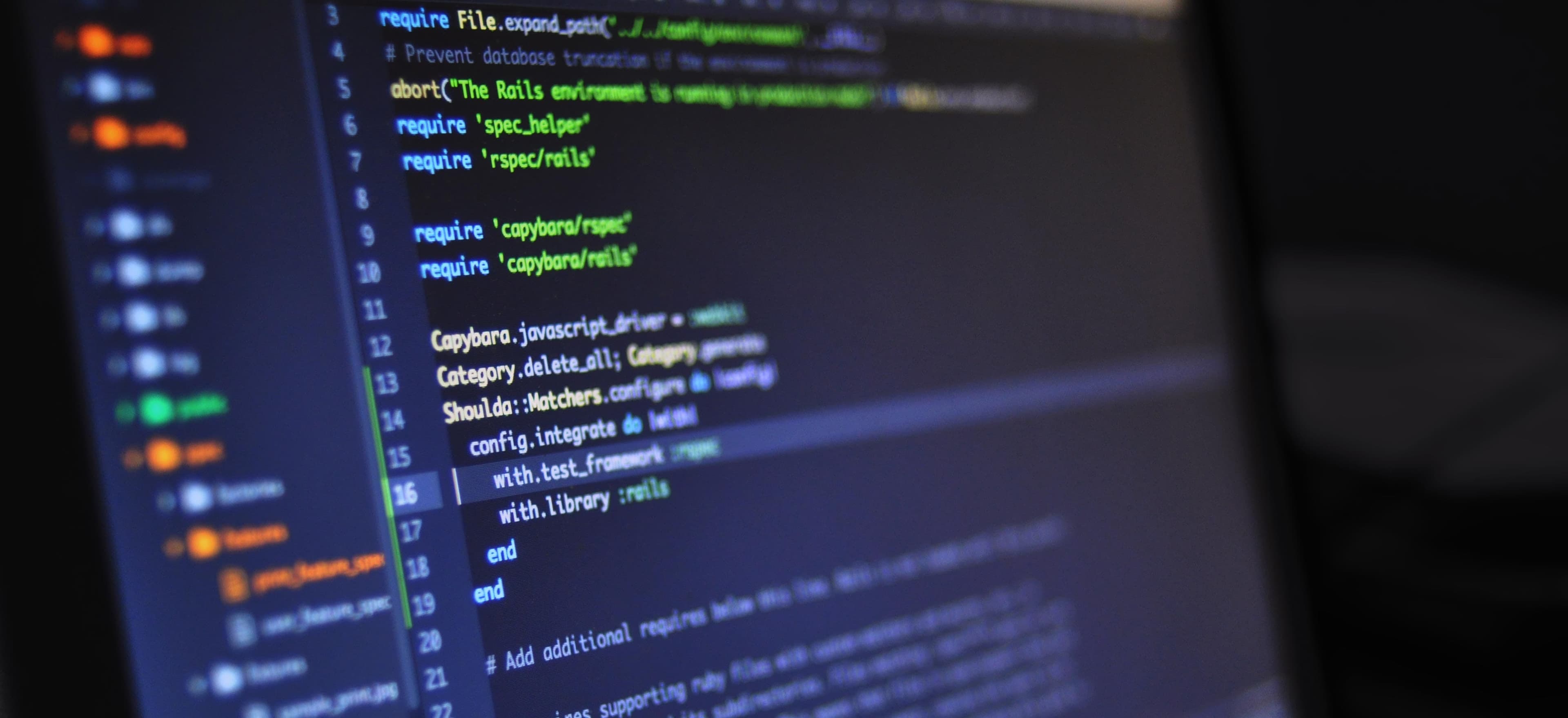
- Published on
Avoiding the Parameterless Generic Method Antipattern in Java
In today's ever-evolving world of software development, it's critical for developers to write clean, maintainable, and efficient code. One common pitfall that many Java developers encounter is the parameterless generic method antipattern. This blog post will explore what this antipattern is, why it occurs, and how you can effectively avoid it in your Java projects.
Understanding the Parameterless Generic Method Antipattern
A parameterless generic method is a method that uses generics but does not have any parameters. While this may seem harmless at first glance, it can lead to issues like reduced readability, anticipatory complexity, and unintended consequences for maintainability.
Why You Should Care
When developers encounter parameterless generic methods, they might unknowingly introduce ambiguity into their code. This can lead to misunderstanding when reading or maintaining code later. A developer unfamiliar with the code base might assume that the generic type can safely be used with any type, which ultimately complicates things.
Example of the Antipattern
Consider the following simple generic class in Java that demonstrates a parameterless generic method:
public class GenericContainer<T> {
private T item;
public void storeItem() {
// storing a new item without any parameters
item = null; // this line effectively does nothing useful
}
public T retrieveItem() {
return item;
}
}
In the above example, storeItem()
does not accept any parameters and does not perform any meaningful action with the generic type T
. The lack of parameters presents a clear problem—it leaves the method devoid of purpose, thereby fostering an unintentional misuse of generics.
Issues Arising from This Antipattern
-
Reduced Clarity: When someone reads the code, it becomes unclear what
storeItem()
is supposed to do. It's ambiguous and can confuse others reviewing your code or even your future self. -
Increased Misusage: Because the method does not require any parameters, it opens up the potential for misuse. Someone might think they can get away with calling it but have no control over what they're storing in the generic type.
-
Maintenance Nightmare: Over time, as the code evolves, the purpose of parameterless methods can get lost. Understanding why a method exists without context becomes significantly more challenging.
Best Practices to Avoid Parameterless Generic Method Antipattern
Now that we've laid out the problem, let’s explore how to rectify issues stemming from parameterless generic methods, including some viable strategies and exemplary implementations.
1. Use Method Parameters Effectively
To ensure clarity in your methods, they should accept parameters that make their purpose explicit. Here’s how we can refactor the earlier example:
public class GenericContainer<T> {
private T item;
public void storeItem(T newItem) {
item = newItem; // Store the new item
}
public T retrieveItem() {
return item; // Retrieve the previously stored item
}
}
Commentary on the Refactored Code
- Clarity: Now, in order to store an item, one must provide a specific object. This makes the method usage self-explanatory.
- Functional Context: Each method has a distinct purpose which can lead to more maintainable code.
2. Enforce Type Safety
Generics should become your friends. Use type constraints when necessary to enforce various types that a method can accept. Through this mechanism, you can achieve better type safety:
public class GenericNumberContainer<T extends Number> {
private T number;
public void storeNumber(T newNumber) {
number = newNumber; // stores only a Number or its subclasses
}
public T retrieveNumber() {
return number; // retrieves the stored number
}
}
Why This Matters
- Specificity: By constraining the type to
Number
, you're ensuring that only numeric types are stored, making the class more robust. - Reduce Runtime Errors: Using generics with specific constraints reduces the risk of runtime errors caused by type mismatches.
3. Document Generics Usage
If you find it necessary to implement a generic method that does not take parameters (although it is not recommended), make sure to document its usage clearly.
/**
* A utility class that performs operations on generic types.
* This method is designed to initialize the container without parameters.
*/
public class GenericInitializer<T> {
private T item;
public void init() {
// Initialization logic, consider writing what needs to happen here
}
}
4. Leverage Optional for Return Values
If your design insists you might not have anything to return, consider using Optional
. By doing that, you can signal the absence of a return value effectively without falling into the trap of parameterless methods:
import java.util.Optional;
public class OptionalGenericContainer<T> {
private T item;
public void storeItem(T newItem) {
item = newItem;
}
public Optional<T> retrieveItem() {
return Optional.ofNullable(item); // Use Optional to convey absence of value
}
}
Significance of Using Optional
- Explicit Intent: You convey the intention that the return value of
retrieveItem
can also be absent, addressing potential NullPointerExceptions more gracefully. - Enhanced Code Understanding: Other developers will recognize that they may need to check for a value before proceeding with usage.
5. Testing and Optimization
Ensure rigorous testing of your generics. Use JUnit to validate your generic classes and methods.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class GenericContainerTest {
@Test
public void testStoreAndRetrieve() {
GenericContainer<String> container = new GenericContainer<>();
container.storeItem("Hello");
assertEquals("Hello", container.retrieveItem());
}
@Test
public void testOptionalContainer() {
OptionalGenericContainer<Integer> container = new OptionalGenericContainer<>();
container.storeItem(42);
assertTrue(container.retrieveItem().isPresent());
}
}
To Wrap Things Up
Avoiding the parameterless generic method antipattern is crucial for writing robust Java code that is easy to maintain and understand. By ensuring methods accept meaningful parameters, enforcing type safety, and employing effective documentation, you can significantly enhance the quality of your software.
Moreover, leveraging Optional can provide a clearer signal regarding potential null returns, which will assist in creating more reliable applications.
For further reading on generics in Java, consider consulting the Java documentation on Generics.
Embrace these best practices and steer clear of the antipattern. Happy coding!