Overcoming Naming Conventions: The Method Equality Dilemma
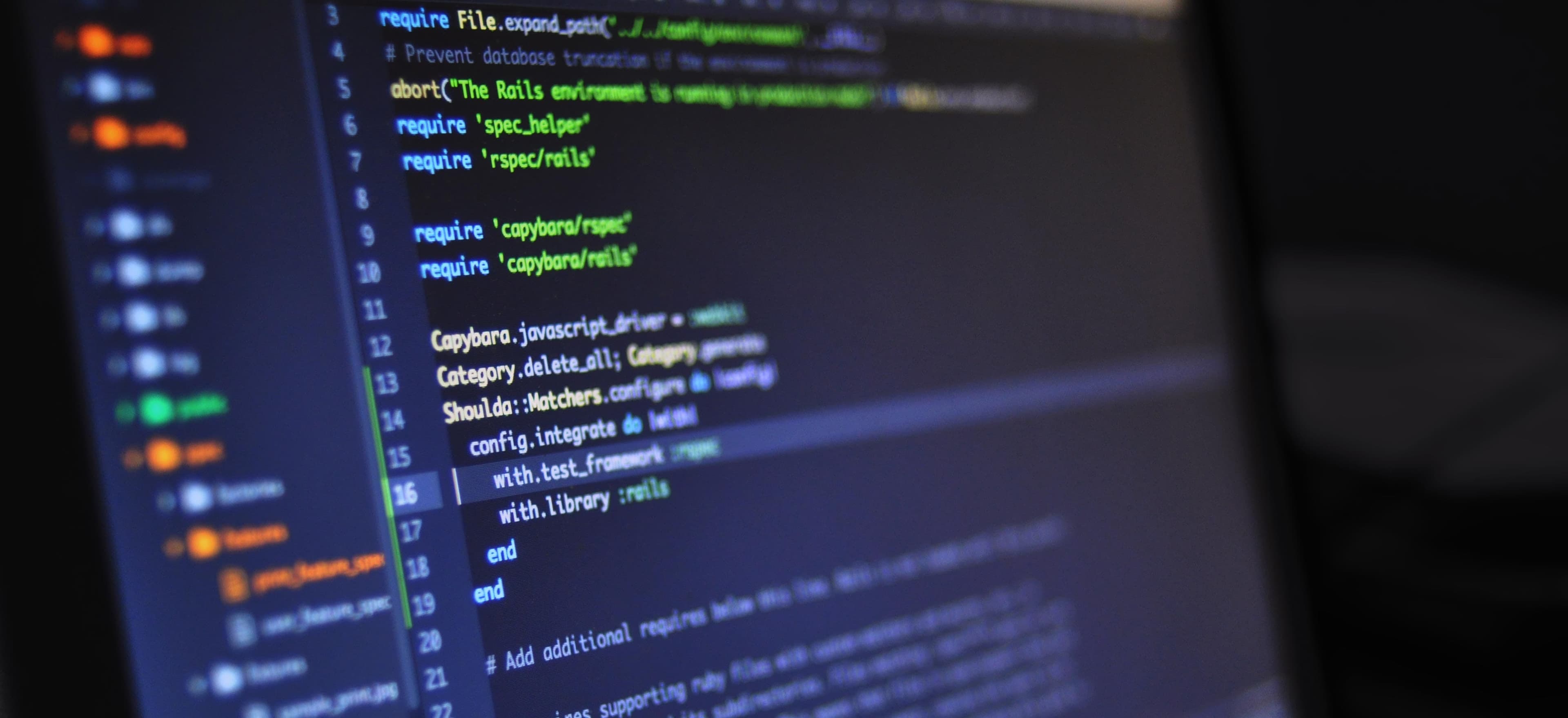
- Published on
Overcoming Naming Conventions: The Method Equality Dilemma
When working with Java, one of the fundamental concepts that a developer encounters is the notion of method equality. Understanding how Java determines the equality of methods is crucial for creating robust and maintainable code. In this article, we will delve into the intricacies of method equality in Java, explore the associated naming conventions, and discuss best practices for overcoming the method equality dilemma.
Method Equality in Java
In Java, method equality refers to the comparison of two methods to determine if they are the same. This comparison is essential for various tasks, including reflection, method overriding, and method overloading. When two methods are considered equal, it means that they have the same name, parameter types, and return type.
Let's consider the following example:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
In this scenario, the Calculator
class contains two add
methods. The first method takes two int
parameters and returns an int
, while the second method takes two double
parameters and returns a double
. Despite both methods being named add
, they are considered different due to their distinct parameter types and return types.
Naming Conventions for Overloading Methods
When overloading methods in Java, adhering to naming conventions is crucial for writing readable and maintainable code. The method naming should reflect the operation being performed and the types of parameters involved. It's important to choose meaningful and descriptive names that accurately convey the functionality of each method.
Consider the following example that demonstrates method overloading while adhering to naming conventions:
public class StringUtils {
public String merge(String str1, String str2) {
return str1 + str2;
}
public String merge(String str1, String str2, String str3) {
return str1 + str2 + str3;
}
}
In this case, the merge
method is overloaded to handle different numbers of input parameters. By using the same base method name and providing distinct parameter lists, the code remains intuitive and self-explanatory.
The Importance of Method Equality
Understanding method equality is vital when working with Java's reflection API, which allows for the inspection and invocation of classes, methods, and fields at runtime. When using reflection, knowing whether two methods are equal enables developers to accurately locate and manipulate specific methods within a class or its hierarchy.
Method[] methods = Calculator.class.getMethods();
for (Method method : methods) {
if (method.getName().equals("add") && method.getParameterCount() == 2) {
// Perform operations based on the matched method
}
}
In this snippet, the code utilizes reflection to iterate through the methods of the Calculator
class. By comparing method names and parameter counts, the program can selectively execute operations based on the matched method, showcasing the significance of method equality in practical scenarios.
Overcoming the Method Equality Dilemma
When faced with the task of ensuring method equality, developers can leverage the java.lang.reflect.Method
class, which provides various methods for comparing and inspecting methods.
Let's consider an example where method equality is evaluated using the Method
class:
import java.lang.reflect.Method;
public class MethodEqualityExample {
public static void main(String[] args) {
Method[] methods = StringUtils.class.getMethods();
for (Method method : methods) {
if (method.getName().equals("merge") && method.getParameterCount() == 2) {
System.out.println("Matching method found: " + method);
}
}
}
}
In this illustration, the MethodEqualityExample
class uses reflection to retrieve the methods of the StringUtils
class. By comparing the method name and parameter count, the program identifies and outputs the matching method.
Best Practices for Method Equality
To ensure clarity and maintainability in code that involves method equality, it's essential to follow best practices:
-
Descriptive Method Names: Choose method names that clearly indicate their purpose and operation, aiding in the understanding of method overloads and overrides.
-
Consistent Parameter Types: Maintain consistency in the types and ordering of parameters across overloaded methods, enabling accurate method equality comparisons.
-
Utilize Annotations: Consider utilizing annotations such as
@Override
to explicitly indicate method overriding, enhancing code readability and reducing ambiguity. -
Unit Testing: Write comprehensive unit tests to validate method equality and ensure the expected behavior across different scenarios, safeguarding against unintended method discrepancies.
Final Thoughts
Understanding method equality and adhering to naming conventions are essential aspects of Java development. By comprehending the nuances of method equality and employing best practices, developers can create robust, maintainable, and reflective Java code. Remember, method equality goes beyond just comparing method names; it encapsulates the entire signature of the method, including its parameters and return type. Embracing these principles will lead to code that is not only efficient but also comprehensible to both present and future developers.
For further reading on method equality and best practices in Java, you can refer to the Java SE Documentation.
In conclusion, method equality in Java presents a vital consideration for developers, impacting various facets of the language's functionality. Through a combination of understanding, adherence to naming conventions, and utilization of best practices, developers can navigate the method equality dilemma and craft robust, reflective Java code.