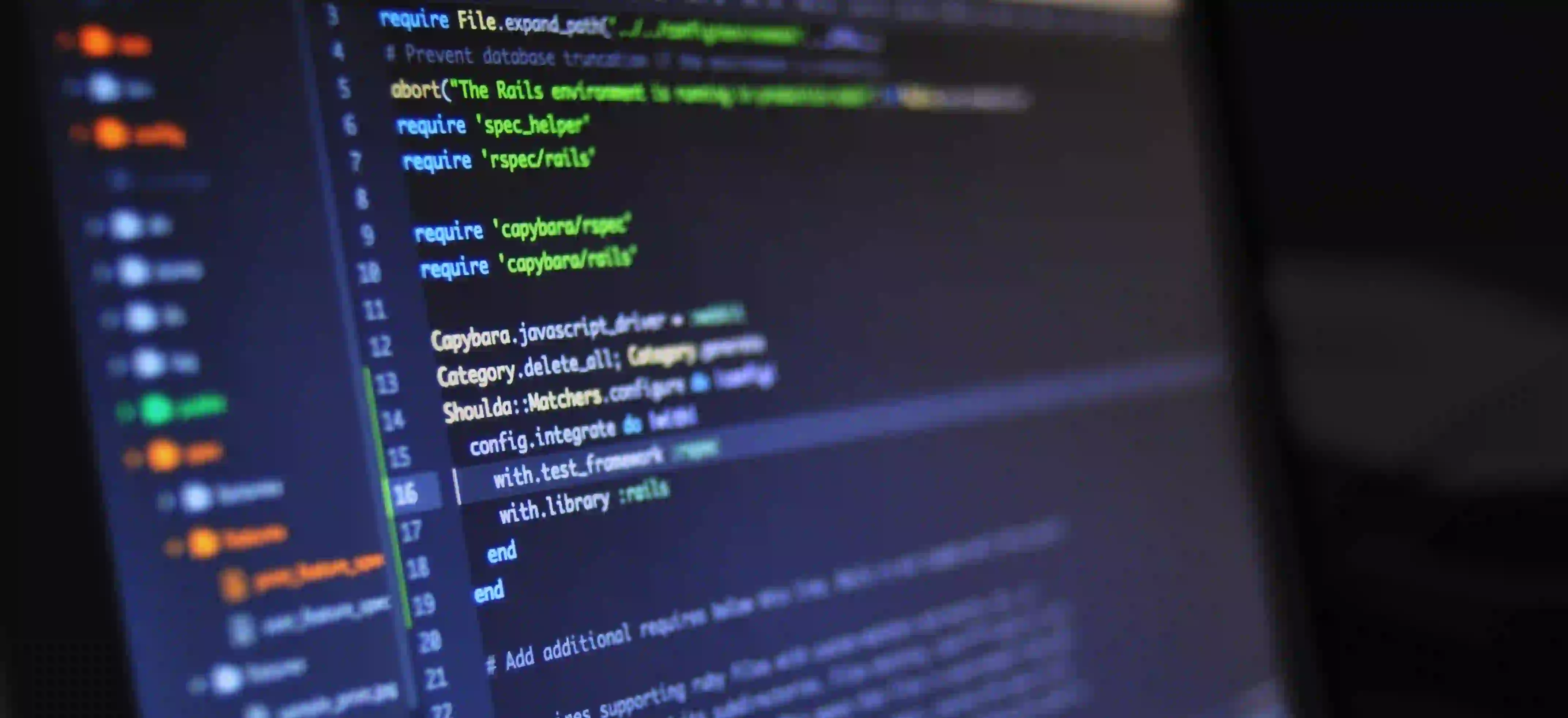
Leveraging Spring Integration MongoDB Adapters with Java DSL
In the realm of enterprise application development with Java, efficient data integration plays a pivotal role. The seamless integration of diverse data sources, including databases, is essential for streamlined application performance. In this context, leveraging Spring Integration MongoDB Adapters with Java DSL presents an efficient and robust approach.
Stepping into the Topic to Spring Integration MongoDB Adapters
Spring Integration, an extension of the Spring programming model, facilitates the integration of enterprise applications using messaging. It provides support for building messaging-driven systems, offering a wide array of adapters and gateways for seamless integration with various systems.
MongoDB, a NoSQL database, has gained immense popularity for its scalability and flexibility. Spring Integration MongoDB Adapters enable the integration of MongoDB with other systems, fostering efficient data exchange.
Setting Up the Environment
Before delving into the integration process, ensure that the necessary tools and dependencies are set up.
Add Spring Integration MongoDB Dependency
Integrate the Spring Integration MongoDB Dependency into your project using Maven:
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-mongodb</artifactId>
<version>5.5.0</version>
</dependency>
Configure MongoDB Connection
Configure the MongoDB connection details in the application properties or configuration class:
@Configuration
public class MongoDbConfig {
@Bean
public MongoClientFactoryBean mongo() {
MongoClientFactoryBean mongo = new MongoClientFactoryBean();
mongo.setHost("localhost");
return mongo;
}
@Bean
public MongoDbFactory mongoDbFactory(MongoClient mongo) {
return new SimpleMongoClientDbFactory(mongo, "your-database");
}
}
Integration with Java DSL
Spring Integration provides a Java DSL for configuring integration flows using a fluent and expressive API. Let's explore how to leverage the Java DSL to integrate MongoDB within the Spring Integration framework.
Outbound Adapter Configuration
To persist data to MongoDB using the Java DSL, configure an outbound adapter:
@Bean
public IntegrationFlow outboundMongoDB() {
return IntegrationFlows.from("inputChannel")
.handle(Mongo.outboundAdapter(mongoDbFactory)
.collectionNameExpression("'your-collection'")
.mongoTemplate(mongoTemplate))
.get();
}
In the above code snippet, IntegrationFlows
is used to start the integration flow configuration. The Mongo.outboundAdapter
sets up the outbound MongoDB adapter, specifying the collection name and the corresponding mongoDbFactory
and mongoTemplate
.
Inbound Adapter Configuration
For retrieving data from MongoDB, configure an inbound adapter using the Java DSL:
@Bean
public IntegrationFlow inboundMongoDB() {
return IntegrationFlows.from(Mongo.inboundAdapter(mongoDbFactory)
.collectionName("your-collection")
.query("{ 'status': 'active' }"))
.handle(m -> {
// Process retrieved data
})
.get();
}
The Mongo.inboundAdapter
configures the inbound MongoDB adapter, specifying the collection name and an optional query to retrieve specific data.
Error Handling
Incorporate error handling within the integration flows to gracefully manage exceptions and failures:
@Bean
public IntegrationFlow errorHandlingFlow() {
return IntegrationFlows.from("errorChannel")
.handle(m -> {
// Perform error handling
})
.get();
}
The above errorHandlingFlow
captures errors from any integration flow and provides a mechanism to handle them appropriately.
Benefits of Using Java DSL with MongoDB Adapters
- Simplified Configuration: Java DSL provides a more concise and readable way to configure integration flows, enhancing maintainability.
- Type Safety: Leveraging Java constructs ensures type safety and reduces the likelihood of configuration errors.
- Flexible and Extensible: The Java DSL allows for easy integration with custom components and business logic, providing flexibility in implementation.
A Final Look
Integrating MongoDB with Spring Integration using the Java DSL offers an effective and elegant means of data exchange. By utilizing the expressive and fluent Java DSL, developers can seamlessly configure integration flows for MongoDB, ensuring efficient communication between the application and the MongoDB database.
By following the outlined approach and customizing the configurations to suit specific requirements, developers can harness the power of Spring Integration MongoDB Adapters with Java DSL to build robust and seamlessly integrated enterprise applications.
For further information on Spring Integration MongoDB Adapters and Java DSL, consult the official documentation: