Hadoop & JBoss Data Virtualization Integration Challenges
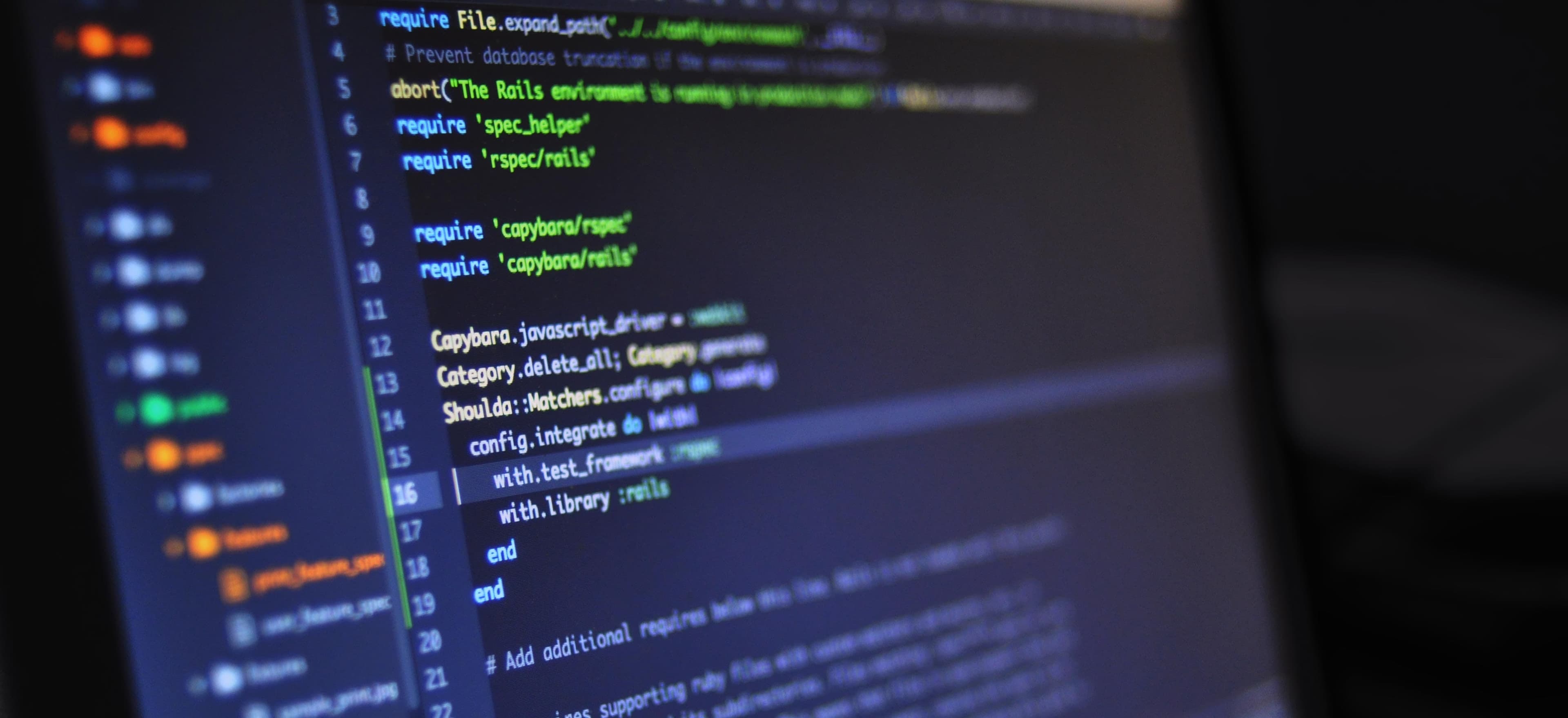
- Published on
Integrating Hadoop with JBoss Data Virtualization: Overcoming Challenges
In today's data-driven world, businesses are increasingly leveraging technologies like Hadoop and JBoss Data Virtualization to manage and derive insights from large volumes of data. Hadoop, with its distributed file system and parallel processing capabilities, has become a go-to choice for handling big data, while JBoss Data Virtualization provides a unified view of multiple data sources in real-time. Integrating these two powerful platforms can unlock new possibilities for organizations, but it also presents several challenges. In this article, we will discuss the key challenges of integrating Hadoop with JBoss Data Virtualization and explore how to overcome them using Java.
Challenge 1: Data Access and Connectivity
The Challenge:
One of the primary challenges when integrating Hadoop with JBoss Data Virtualization is enabling seamless connectivity and access to the data stored in Hadoop Distributed File System (HDFS). HDFS has its own way of storing and managing data, which is different from traditional relational databases.
The Solution:
Java provides robust libraries such as Apache Hadoop FileSystem API which allows easy interaction with HDFS. You can use this API to read and write data to Hadoop from JBoss Data Virtualization. Let's take a look at a simple code snippet that demonstrates how to access a file in HDFS using Java:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class HdfsReader {
public static void main(String[] args) {
String uri = "hdfs://your-hdfs-uri/file.txt";
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(URI.create(uri), conf);
Path path = new Path(uri);
try (FSDataInputStream in = fs.open(path)) {
// Read data from the input stream
} catch (IOException e) {
e.printStackTrace();
}
}
}
In the above code, we use the FileSystem
and Path
classes from the Hadoop library to open a file in HDFS and read data from it. This approach allows seamless connectivity to Hadoop from within your Java application, thus addressing the data access and connectivity challenge.
Challenge 2: Data Transformation and Integration
The Challenge:
Another significant challenge is transforming and integrating the data from Hadoop into a format that can be seamlessly consumed by JBoss Data Virtualization. Hadoop stores data in its native format, and it may require preprocessing and transformation before it can be integrated into the virtual data layer provided by JBoss Data Virtualization.
The Solution:
Java offers a plethora of data processing and transformation libraries that can be utilized to address this challenge. Apache Spark, a popular big data processing framework, provides a rich set of APIs for manipulating data, and it seamlessly integrates with Hadoop. You can leverage the power of Apache Spark within your Java application to perform data transformation tasks on the data retrieved from Hadoop before integrating it into JBoss Data Virtualization.
Here's a simplified example of using Apache Spark with Java to transform data from Hadoop before integrating it into JBoss Data Virtualization:
import org.apache.spark.api.java.JavaRDD;
import org.apache.spark.sql.*;
public class SparkDataTransformation {
public static void main(String[] args) {
SparkSession spark = SparkSession.builder()
.appName("DataTransformation")
.master("local")
.getOrCreate();
// Load data from Hadoop into Spark
Dataset<Row> rawData = spark.read().format("csv")
.option("header", "true")
.load("hdfs://your-hdfs-uri/data.csv");
// Perform transformation using Spark SQL or DataFrame APIs
rawData.createOrReplaceTempView("rawData");
Dataset<Row> transformedData = spark.sql("SELECT * FROM rawData WHERE condition = 'met'");
// Write the transformed data back to Hadoop or another storage
transformedData.write().format("parquet").save("hdfs://your-hdfs-uri/transformedData.parquet");
}
}
In this example, we use Apache Spark to read data from Hadoop, apply a transformation using SQL queries, and then write the transformed data back to Hadoop. This demonstrates how Java can be used to leverage the data transformation capabilities of Apache Spark to process data from Hadoop before integrating it with JBoss Data Virtualization.
Challenge 3: Performance Optimization
The Challenge:
Integrating Hadoop with JBoss Data Virtualization also brings forth the challenge of performance optimization. When dealing with large volumes of data, ensuring optimal performance becomes crucial. Data retrieval, transformation, and integration processes need to be optimized to minimize latency and maximize throughput.
The Solution:
Java provides powerful tools and techniques to optimize the performance of data processing tasks when integrating Hadoop with JBoss Data Virtualization. Utilizing multithreading and asynchronous programming can significantly improve performance by parallelizing data retrieval and transformation tasks.
Let's consider a code snippet that showcases how Java's multithreading capabilities can be utilized to improve the performance of data retrieval from Hadoop:
import java.util.concurrent.*;
public class DataRetrieval {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
executor.execute(new DataRetrievalTask("file" + i + ".txt"));
}
executor.shutdown();
while (!executor.isTerminated()) {
// Wait for all tasks to finish
}
System.out.println("Data retrieval completed.");
}
static class DataRetrievalTask implements Runnable {
private String fileName;
public DataRetrievalTask(String fileName) {
this.fileName = fileName;
}
@Override
public void run() {
// Retrieve data from Hadoop for the given file
}
}
}
In this example, we use Java's ExecutorService
to execute data retrieval tasks concurrently, thereby improving the overall performance of the data retrieval process from Hadoop.
The Closing Argument
Integrating Hadoop with JBoss Data Virtualization presents various challenges, including data access and connectivity, data transformation and integration, and performance optimization. By leveraging the capabilities of Java and its rich ecosystem of libraries and frameworks, these challenges can be effectively addressed. The code snippets provided throughout this article illustrate how Java can be used to overcome these challenges and enable seamless integration between Hadoop and JBoss Data Virtualization, ultimately empowering organizations to harness the full potential of their big data assets.
In summary, the combination of Hadoop and JBoss Data Virtualization can be a powerful asset for organizations seeking to extract value from their big data. With the right approach and the utilization of Java's strengths in data processing and integration, the challenges of integrating these two platforms can be transformed into opportunities for innovation and insight.
By addressing these challenges, businesses can capitalize on the wealth of data stored in Hadoop and gain a unified view of their data through JBoss Data Virtualization, ultimately driving informed decision-making and competitive advantage in today's data-driven landscape.