Scaling Microservices with Multi-Cluster Ingress
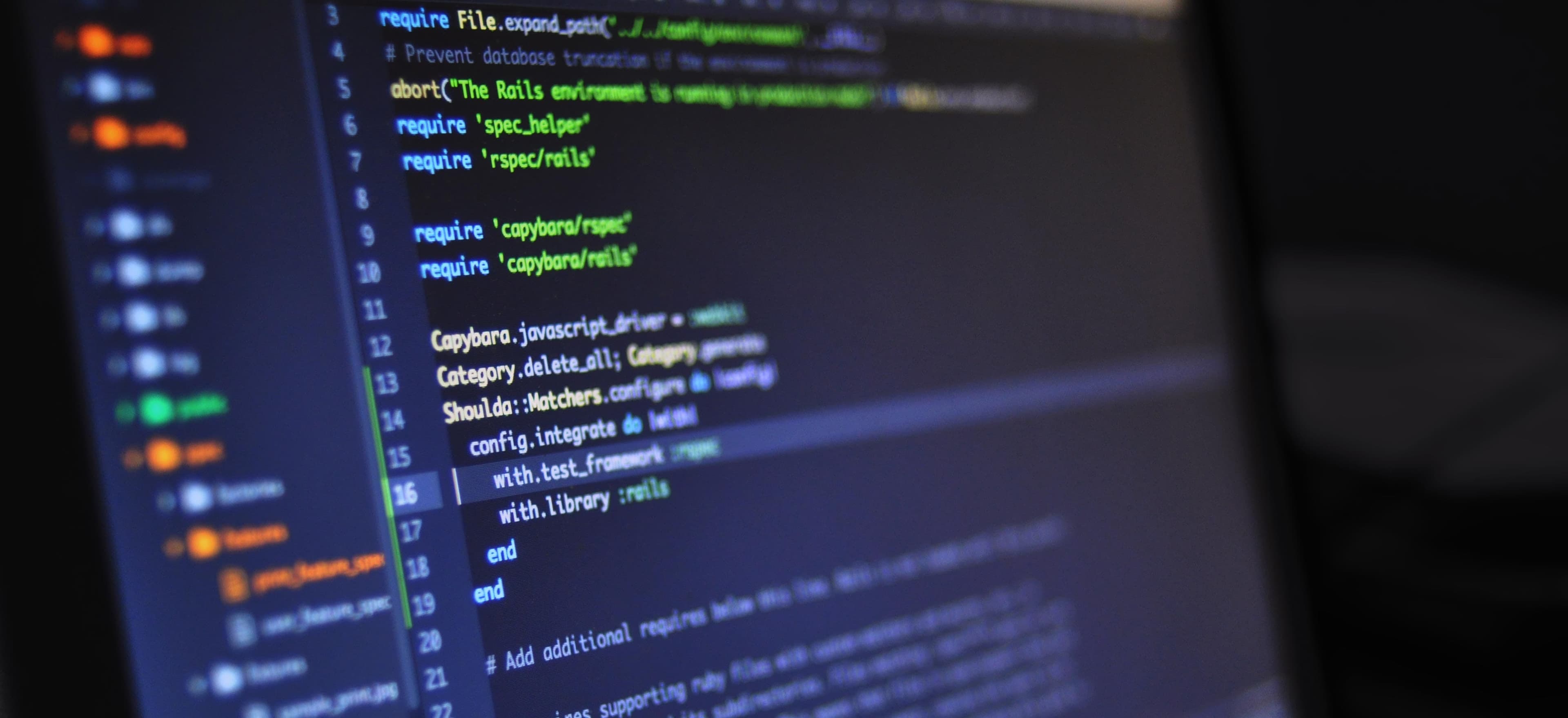
- Published on
Scaling Microservices with Multi-Cluster Ingress
Microservices have revolutionized the way we build and deploy applications, enabling teams to work independently, scale components, and use different technology stacks. However, scaling microservices across multiple clusters can be challenging, especially when it comes to managing the ingress of traffic to these services.
In this article, we'll explore how to scale microservices with multi-cluster ingress using Java, Kubernetes, and Istio. We'll discuss the challenges of managing ingress in a multi-cluster environment and provide a step-by-step guide to implementing multi-cluster ingress for your Java microservices.
Challenges of Multi-Cluster Ingress
In a multi-cluster environment, microservices are distributed across different Kubernetes clusters, each serving a specific purpose or region. Managing the ingress of traffic to these microservices can be complex for several reasons:
- Cross-Cluster Communication: Microservices in different clusters need to communicate with each other, requiring a robust networking solution.
- Traffic Distribution: Incoming traffic needs to be distributed across clusters based on various criteria such as load, proximity, or specific routing rules.
- Security and Compliance: Ensuring consistent security policies and compliance across multiple clusters can be challenging.
Istio and Multi-Cluster Ingress
Istio is an open-source service mesh that provides a unified way to connect, secure, and manage microservices. It offers advanced traffic management, policy enforcement, and telemetry features, making it an ideal solution for managing multi-cluster ingress.
By leveraging Istio's capabilities, we can achieve seamless communication and traffic management across multiple Kubernetes clusters. Istio's Virtual Mesh enables us to treat multiple Kubernetes clusters as a single mesh, simplifying the management of cross-cluster communication and traffic routing.
Implementing Multi-Cluster Ingress with Java and Istio
Prerequisites
Before we dive into the implementation, ensure that you have the following prerequisites in place:
- Kubernetes Clusters: Set up multiple Kubernetes clusters, such as those provided by Google Kubernetes Engine (GKE) or Amazon Elastic Kubernetes Service (EKS).
- Istio Installation: Install Istio on each Kubernetes cluster. Refer to the official documentation for detailed instructions.
- Java Microservices: Have Java microservices ready for deployment to the Kubernetes clusters.
Step 1: Configure Istio Virtual Mesh
In a multi-cluster setup, we need to configure Istio's Virtual Mesh to connect the Kubernetes clusters. This involves defining a single Istio mesh across multiple clusters.
Create a virtualmesh.yaml
file with the following configuration:
apiVersion: networking.istio.io/v1alpha3
kind: VirtualMesh
metadata:
name: my-virtual-mesh
spec:
meshes:
- name: cluster1
- name: cluster2
# Add more clusters as needed
Apply the configuration to each Kubernetes cluster using the following command:
kubectl apply -f virtualmesh.yaml
Step 2: Deploy Java Microservices
Next, deploy your Java microservices to the Kubernetes clusters. Ensure that the microservices are instrumented with the Istio sidecar proxy to enable Istio's features such as traffic routing, security policies, and telemetry.
Here's an example of deploying a Java microservice with Istio sidecar injection:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-java-app
spec:
replicas: 3
selector:
matchLabels:
app: my-java-app
template:
metadata:
labels:
app: my-java-app
spec:
containers:
- name: my-java-app
image: my-registry/my-java-app:latest
# Inject Istio sidecar
sidecar.istio.io/inject: "true"
Step 3: Define Istio Gateway and VirtualService for Multi-Cluster Ingress
In each Kubernetes cluster, define an Istio Gateway and VirtualService to manage the ingress of traffic for the Java microservices.
Create a gateway.yaml
file for the Istio Gateway:
apiVersion: networking.istio.io/v1alpha3
kind: Gateway
metadata:
name: my-gateway
spec:
selector:
istio: ingressgateway
servers:
- port:
number: 80
name: http
protocol: HTTP
hosts:
- "my-java-app.com"
Apply the gateway.yaml
configuration using the following command:
kubectl apply -f gateway.yaml
Next, define the VirtualService to route traffic to the Java microservices:
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: my-virtual-service
spec:
hosts:
- "my-java-app.com"
gateways:
- my-gateway
http:
- route:
- destination:
host: my-java-app
subset: v1
Apply the VirtualService configuration to each Kubernetes cluster.
Step 4: Test Multi-Cluster Ingress
Once the configurations are in place, test the multi-cluster ingress by sending traffic to my-java-app.com
. Istio will intelligently route the traffic to the appropriate Kubernetes cluster based on the defined routing rules and load balancing policies.
Bringing It All Together
Scaling microservices across multiple clusters requires a robust approach to managing ingress, traffic distribution, and security. By leveraging Istio's capabilities, particularly its Virtual Mesh feature, we can achieve seamless multi-cluster ingress for Java microservices.
In this article, we've walked through the process of configuring Istio Virtual Mesh, deploying Java microservices with Istio sidecar injection, and defining Istio Gateway and VirtualService for multi-cluster ingress. With these steps, you can effectively scale and manage Java microservices across a multi-cluster environment.
Remember, while Istio provides powerful features for multi-cluster ingress, it's essential to stay updated with the latest best practices and security considerations when working with microservices and service meshes. Happy scaling!
Now, go ahead and give multi-cluster ingress a try for your Java microservices. Happy coding!
Checkout our other articles