Handling Null Pointer Exceptions: Best Practices
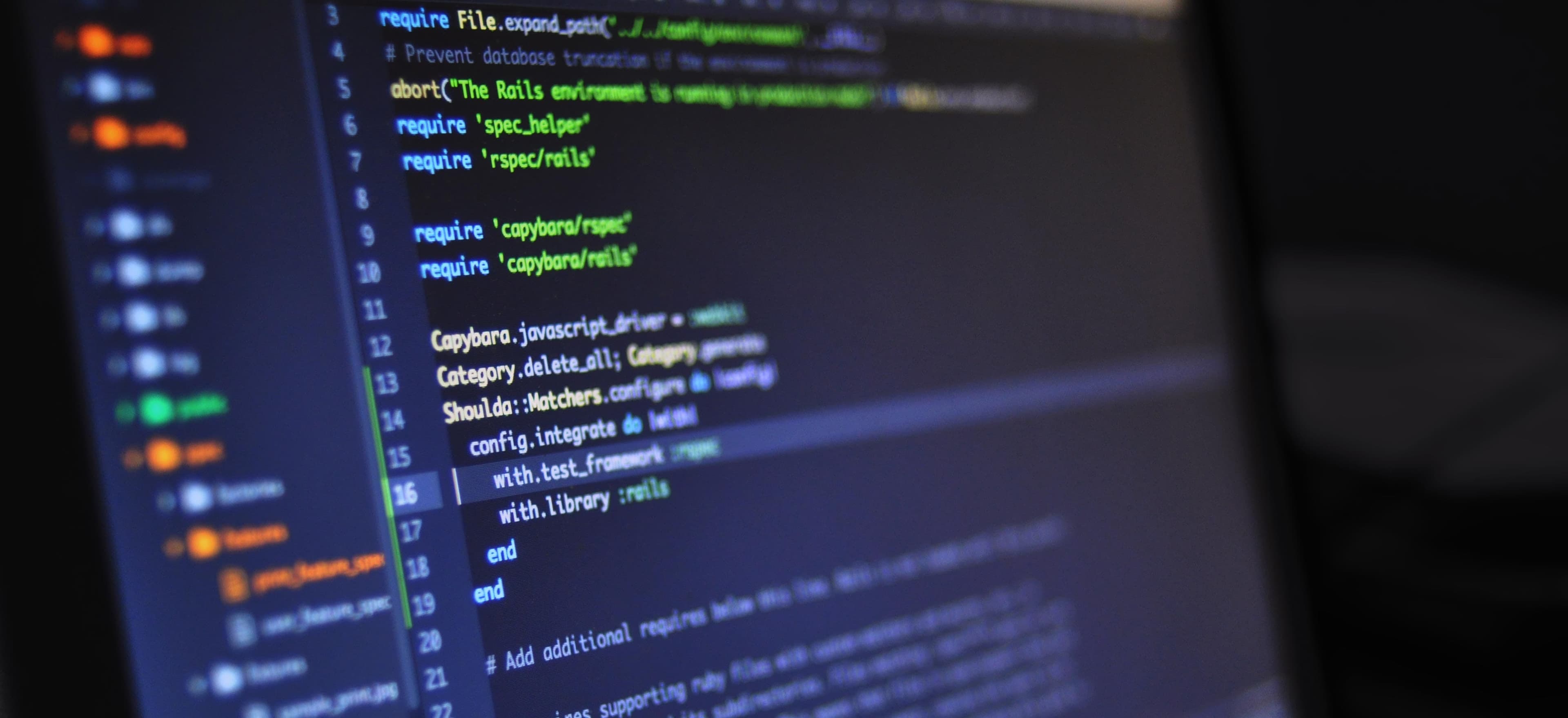
- Published on
Handling Null Pointer Exceptions in Java: Best Practices
Null Pointer Exceptions (NPEs) are one of the most common exceptions encountered by Java developers. These exceptions occur when trying to access a method or field on a null object reference. In this blog post, we'll explore the best practices for handling Null Pointer Exceptions in Java, along with code examples and strategies to minimize their occurrence.
Understanding Null Pointer Exceptions
A Null Pointer Exception is thrown when the code attempts to dereference a null object. This can happen when invoking a method or accessing a field on an object that has not been properly initialized. Consider the following example:
String name = null;
int length = name.length(); // This line will throw a Null Pointer Exception
In the above code, the variable 'name' is assigned a null value, and when the length()
method is invoked on it, a Null Pointer Exception is thrown.
Best Practices for Handling Null Pointer Exceptions
1. Avoid Returning Null Values
One of the best ways to prevent Null Pointer Exceptions is to avoid returning null values from methods whenever possible. Instead of returning null, consider using Optional, which was introduced in Java 8. Optional forces the client to actively handle the absence of a value, thus reducing the risk of NPEs.
public Optional<String> findNameById(int id) {
// If name is found, return Optional with name, else return Optional.empty()
}
2. Null Checking Before Dereferencing
Always perform null checks before dereferencing an object to avoid NPEs. This can be done using an if-else statement or the more concise approach of Java 8's Optional class.
String name = // retrieve name from source
if (name != null) {
int length = name.length(); // Perform operation if name is not null
}
3. Use Objects.requireNonNull() for Method Parameters
When dealing with method parameters, using the Objects.requireNonNull()
method is a good practice to ensure that null values are not passed inadvertently. This approach can help detect and handle null values early in the code.
public void processName(String name) {
Objects.requireNonNull(name, "Name cannot be null");
// Processing continues if name is not null
}
4. Defensive Programming with Objects.requireNonNullElse()
Java 9 introduced Objects.requireNonNullElse()
and Objects.requireNonNullElseGet()
methods, which provide a more flexible way to handle default values for null objects.
String name = // retrieve name from source
String defaultName = "Unknown";
String validName = Objects.requireNonNullElse(name, defaultName);
5. Use Optional with FlatMap for Chained Operations
When dealing with chained operations where the result of one operation might be null, using Optional
with flatMap
can help in handling the absence of values at any stage of the operation chain.
Optional<Detail> detail = getUser()
.flatMap(User::getAddress)
.flatMap(Address::getDetail);
6. Logging and Diagnosing Null Pointer Exceptions
When encountering a Null Pointer Exception, it's essential to log the exception with detailed information to aid in diagnosing the cause. Tools like SLF4J and Log4j can be used for logging purposes.
try {
// Code that may throw Null Pointer Exception
} catch (NullPointerException e) {
logger.error("Null Pointer Exception occurred: {}", e.getMessage());
}
The Bottom Line
Null Pointer Exceptions are common pitfalls in Java programming, but by following the best practices outlined in this post, developers can minimize the occurrence of NPEs and write more robust, stable code. Avoiding null returns, performing null checks, utilizing Optional, and logging exceptions are key strategies in handling and preventing Null Pointer Exceptions. By incorporating these practices into your Java development workflow, you can enhance the reliability and maintainability of your codebase.
Remember, handling Null Pointer Exceptions not only improves the quality of your code but also enhances the overall user experience by reducing unexpected errors.
In closing, always strive to write defensive code that anticipates and handles null references to ensure the smooth functioning of your Java applications.
For more in-depth insights into Java exception handling, consider exploring the official Oracle documentation on Exception Handling and the best practices suggested by experienced Java developers on platforms like Stack Overflow.