Scaling Microservices: Overcoming Data Management Challenges
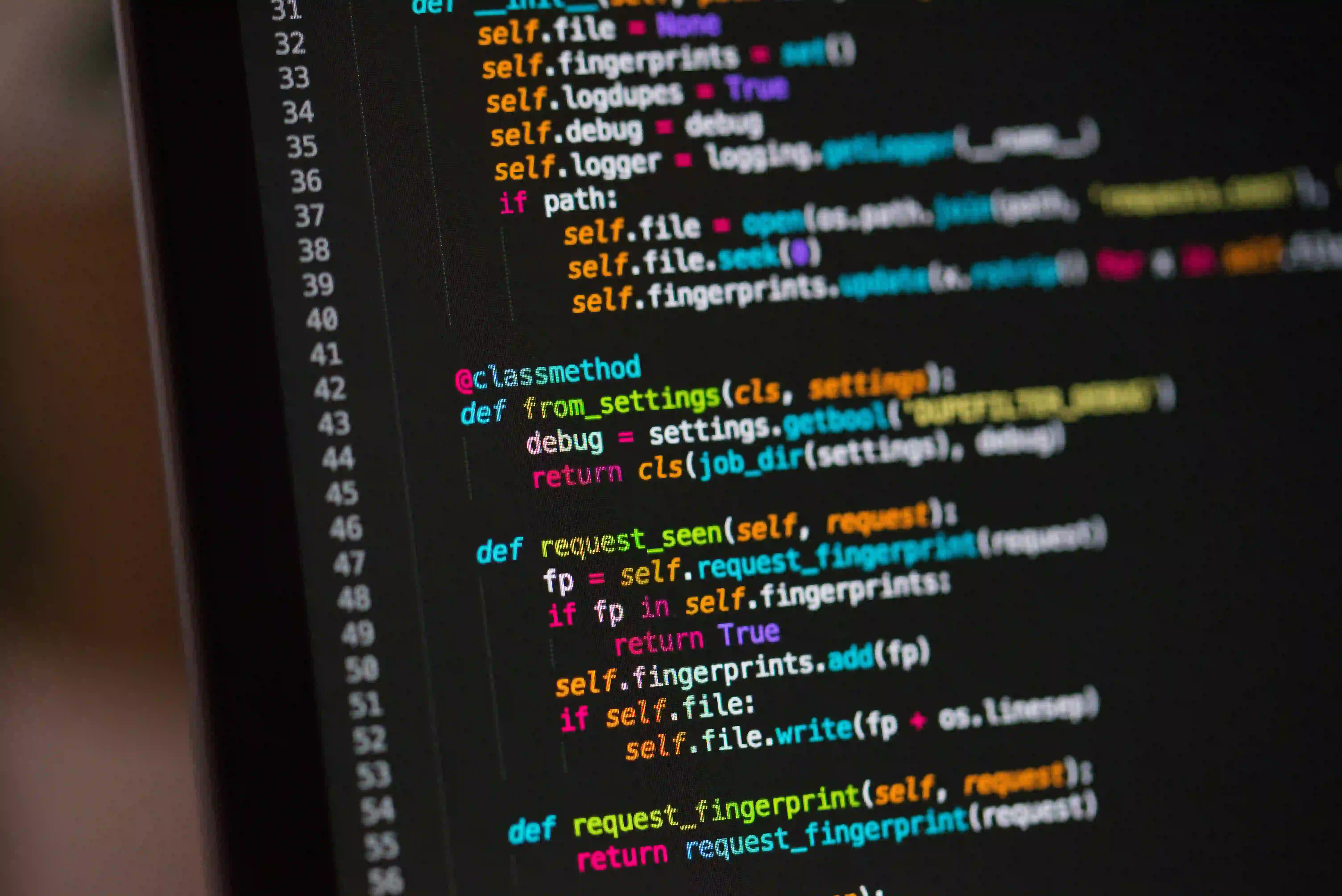
The Importance of Data Management in Microservices Architecture
Microservices architecture has become increasingly popular for building scalable and robust applications. By breaking down monolithic applications into smaller, independent services, organizations can enjoy greater flexibility, agility, and scalability. However, as the number of microservices grows, managing data across these services becomes a critical challenge.
In this article, we'll explore the data management challenges inherent in microservices architecture and discuss strategies for overcoming them using Java-based solutions.
Data Management Challenges in Microservices
1. Data Consistency
Maintaining data consistency across multiple microservices can be complex. Each service may have its own database, and ensuring that data remains consistent and synchronized in a distributed environment poses a significant challenge. Inconsistent data can lead to erroneous business decisions and impact the overall reliability of the system.
2. Data Access
Microservices often need to access and manipulate data from multiple sources. Managing these data access patterns and ensuring efficient communication between services while avoiding excessive network calls is crucial for maintaining performance and scalability.
3. Data Security
Securing data in a microservices environment requires robust strategies. With data distributed across services, ensuring proper access control, encryption, and compliance with privacy regulations becomes more challenging.
4. Data Integration
Integrating data across microservices and external systems without introducing tight coupling or dependencies is a significant concern. Changes in data schemas, APIs, or protocols should not cause disruptions across the entire system.
Overcoming Data Management Challenges with Java Solutions
1. Implementing CQRS (Command Query Responsibility Segregation)
CQRS separates the command (write) and query (read) operations, allowing for independent scaling and optimization. Java frameworks like Axon Framework (https://axoniq.io/product-overview/axon-framework) provide robust support for implementing CQRS patterns, enabling efficient data management within microservices.
// Example of a Command in Axon Framework
@CommandHandler
public void handle(CreateOrderCommand command) {
// Handle the creation of an order
}
In this example, the CreateOrderCommand
is handled independently, facilitating efficient writes without impacting read operations.
2. Using Event Sourcing
Event sourcing, supported by frameworks like Eventuate (https://eventuate.io/), captures all changes to application state as a sequence of events. These events can be replayed to reconstruct past states, enabling reliable data synchronization and consistency across microservices.
// Example of Event Sourcing in Eventuate
public class OrderAggregate {
@Aggregate
private boolean orderCreated;
@CommandHandler
public void handle(CreateOrderCommand command) {
apply(new OrderCreatedEvent());
}
@EventSourcingHandler
public void on(OrderCreatedEvent event) {
this.orderCreated = true;
}
}
By leveraging event sourcing, microservices can maintain consistent data without relying on a single, central database.
3. Using Spring Data
Spring Data provides a powerful and consistent programming model for data access, making it easier to work with different data stores. With its support for JPA, MongoDB, Redis, and other data technologies, Spring Data simplifies the integration of disparate data sources within microservices.
// Example of Spring Data Repository
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
}
Spring Data repositories enable seamless data access and abstraction, allowing microservices to interact with diverse data sources using a unified interface.
Final Considerations
Effectively managing data within a microservices architecture is essential for ensuring reliability, scalability, and maintainability. Java-based solutions such as CQRS, event sourcing, and Spring Data offer powerful tools for overcoming the data management challenges inherent in microservices.
By adopting these strategies and leveraging the capabilities of Java frameworks, organizations can build resilient and efficient microservices architectures capable of handling complex data management requirements.
For more in-depth insights into microservices and Java, continue reading our article on Building Microservices with Java.