Writing Custom Spock Framework Extensions
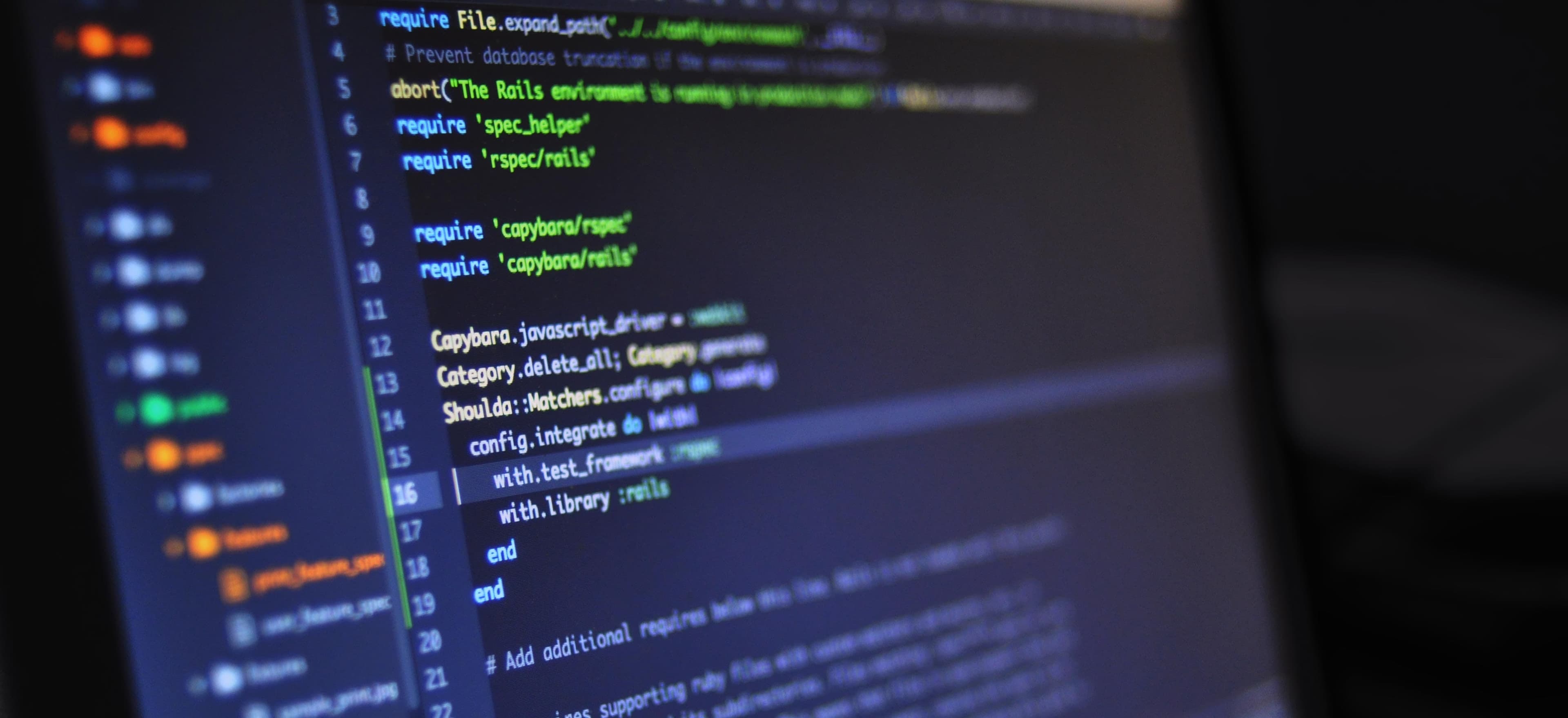
- Published on
Writing Custom Spock Framework Extensions
The Spock framework is a popular and powerful testing and specification framework for Java and Groovy applications. While Spock provides a rich set of features out-of-the-box, there may be scenarios where you need to extend its functionality to better suit your specific testing needs. In this article, we'll explore how to write custom Spock framework extensions to enhance your testing capabilities.
Understanding Spock Extensions
Spock extensions, also known as global extensions, allow you to intercept and modify the behavior of Spock's test execution. This can be useful for various purposes such as custom logging, creating reusable testing utilities, or integrating with third-party tools.
Creating a Custom Spock Extension
To create a custom Spock extension, you'll need to implement the org.spockframework.runtime.extension.IGlobalExtension
interface. This interface defines a single method void visitSpec(SpecInfo spec)
which is called for each specification (test class) before it is executed. Inside this method, you have the opportunity to manipulate the specification, its features (test methods), and their respective execution.
import org.spockframework.runtime.extension.AbstractAnnotationDrivenExtension;
import org.spockframework.runtime.model.SpecInfo;
public class CustomExtension extends AbstractAnnotationDrivenExtension<MyAnnotation> {
@Override
public void visitSpec(SpecInfo spec) {
// Your custom logic here
}
}
In the above code, CustomExtension
is a custom Spock extension that extends AbstractAnnotationDrivenExtension
and is associated with a custom annotation @MyAnnotation
.
Adding Behavior to the Extension
Next, let's consider a scenario where we want our custom Spock extension to log the details of each test method's execution. We can achieve this by intercepting the execution of these methods and adding our custom behavior.
import org.spockframework.runtime.model.FeatureInfo;
import org.spockframework.runtime.model.SpecInfo;
public class CustomExtension extends AbstractAnnotationDrivenExtension<MyAnnotation> {
@Override
public void visitSpec(SpecInfo spec) {
spec.getFeatures().forEach(feature -> {
feature.getFeatureMethod().setBody(() -> {
System.out.println("Executing test: " + feature.getFeatureMethod().getName());
feature.getFeatureMethod().invoke(spec.getReflection());
});
});
}
}
In this code snippet, we're iterating through each test method (or feature) in the specification and replacing its execution logic with a custom behavior that logs its name before invoking it.
Registering the Custom Extension
Once the custom extension is created, it needs to be registered with the Spock framework. This can be done by creating a file named META-INF/services/org.spockframework.runtime.extension.IGlobalExtension
in the src/test/resources
directory of your project, and adding the fully qualified name of the custom extension class to it.
com.example.CustomExtension
By doing this, Spock will automatically discover and load your custom extension when running tests.
Closing Remarks
In conclusion, writing custom Spock framework extensions can greatly enhance the capabilities of the Spock framework and allow you to tailor it to your specific testing needs. By implementing custom logic and behaviors, you can create a more streamlined and efficient testing process.
Moreover, creating custom extensions helps in keeping your test code DRY (Don't Repeat Yourself) by encapsulating common testing patterns and functionalities.
By following the steps outlined in this article, you'll be able to create and register your own custom Spock extensions, opening up a world of possibilities for advanced testing scenarios.
Start integrating custom Spock extensions in your projects today and experience the power of tailored testing capabilities.
For more information on Spock framework and custom extensions, check out the official Spock documentation and the Spock Github repository.