Customizing Spring Social Connect Framework for MongoDB
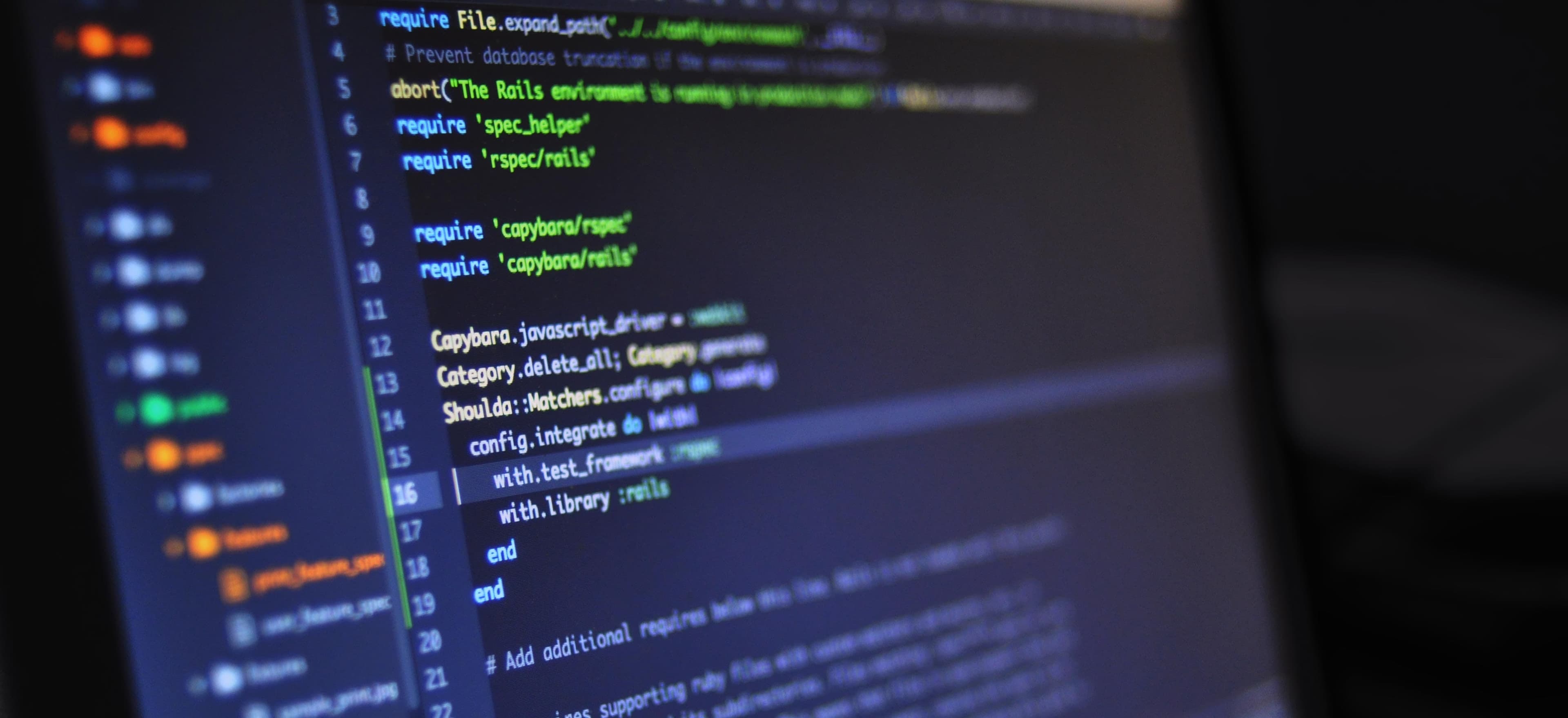
- Published on
Customizing Spring Social Connect Framework for MongoDB
When it comes to integrating social media platforms with Java applications, Spring Social Connect Framework provides a robust and flexible solution. However, out-of-the-box, Spring Social Connect Framework is set up to use a relational database for storing connection details. In this article, we'll explore how to customize Spring Social Connect Framework to use MongoDB as the storage mechanism for social media connections.
Understanding the Spring Social Connect Framework
Before diving into the customization process, let's first take a closer look at Spring Social Connect Framework. This framework allows developers to connect their applications with popular social media platforms such as Facebook, Twitter, and LinkedIn. It provides a set of APIs and services for establishing connections, retrieving user profiles, and interacting with the social media platforms.
By default, Spring Social Connect Framework uses JDBC-based repositories to store connection details. This means that it relies on relational databases like MySQL, PostgreSQL, or H2 to persist connection information.
Using MongoDB with Spring Social Connect Framework
MongoDB is a popular NoSQL database that offers flexibility and scalability, making it a great choice for storing unstructured and semi-structured data such as social media connections. To customize Spring Social Connect Framework to use MongoDB, we'll need to create custom implementations of its connection repository interfaces.
Customizing Connection Repository Interfaces
public interface CustomConnectionRepository extends ConnectionRepository {
// Custom methods for MongoDB operations
}
public class MongoConnectionRepository implements CustomConnectionRepository {
// Implement custom methods using MongoDB operations
}
In the above code snippet, we're creating a custom ConnectionRepository
interface that extends the standard ConnectionRepository
. We then implement the custom interface using MongoDB-specific operations. This allows us to leverage MongoDB's features for storing and retrieving social media connections.
Configuring MongoDB Connection
In addition to customizing the connection repository interfaces, we also need to configure the MongoDB connection within the Spring application context.
@Configuration
@EnableMongoRepositories(basePackages = "com.example.repositories")
public class MongoConfig extends AbstractMongoClientConfiguration {
@Override
protected String getDatabaseName() {
return "social_media";
}
@Override
public MongoClient mongoClient() {
return MongoClients.create("mongodb://localhost:27017");
}
}
In the configuration class above, we're setting up the MongoDB connection for our Spring application. We specify the database name and the connection details for the MongoDB instance. By using @EnableMongoRepositories
, we enable Spring Data MongoDB repositories for interacting with the MongoDB database.
Updating Spring Social Connect Configuration
Finally, we need to update the Spring Social Connect configuration to use our custom MongoDB-based connection repository.
@Configuration
@EnableSocial
public class SocialConfig extends SocialConfigurerAdapter {
@Autowired
private CustomConnectionRepository connectionRepository;
@Override
public void addConnectionFactories(ConnectionFactoryConfigurer connectionFactoryConfigurer, Environment environment) {
// Configure connection factories for social media platforms
}
@Override
public UsersConnectionRepository getUsersConnectionRepository(ConnectionFactoryLocator connectionFactoryLocator) {
return connectionRepository;
}
}
In the SocialConfig
class, we're injecting our custom connection repository and using it to override the default UsersConnectionRepository
provided by Spring Social Connect Framework. This ensures that all social media connections are stored and retrieved using our MongoDB-based implementation.
Closing the Chapter
Customizing Spring Social Connect Framework to use MongoDB as the storage mechanism for social media connections provides greater flexibility and scalability, especially for applications dealing with large volumes of social media interactions. By creating custom connection repository interfaces, configuring the MongoDB connection, and updating the Spring Social Connect configuration, developers can seamlessly integrate MongoDB with the framework.
In summary, leveraging MongoDB with Spring Social Connect Framework opens up new possibilities for building social media-enabled Java applications, allowing for efficient management of social media connections and interactions.
To learn more about integrating MongoDB with Java applications, check out this comprehensive guide to MongoDB Java Driver. Additionally, for in-depth insights into Spring Social Connect Framework, refer to the official Spring Social documentation.
Start customizing Spring Social Connect Framework for MongoDB today and elevate your social media integration capabilities with Java!