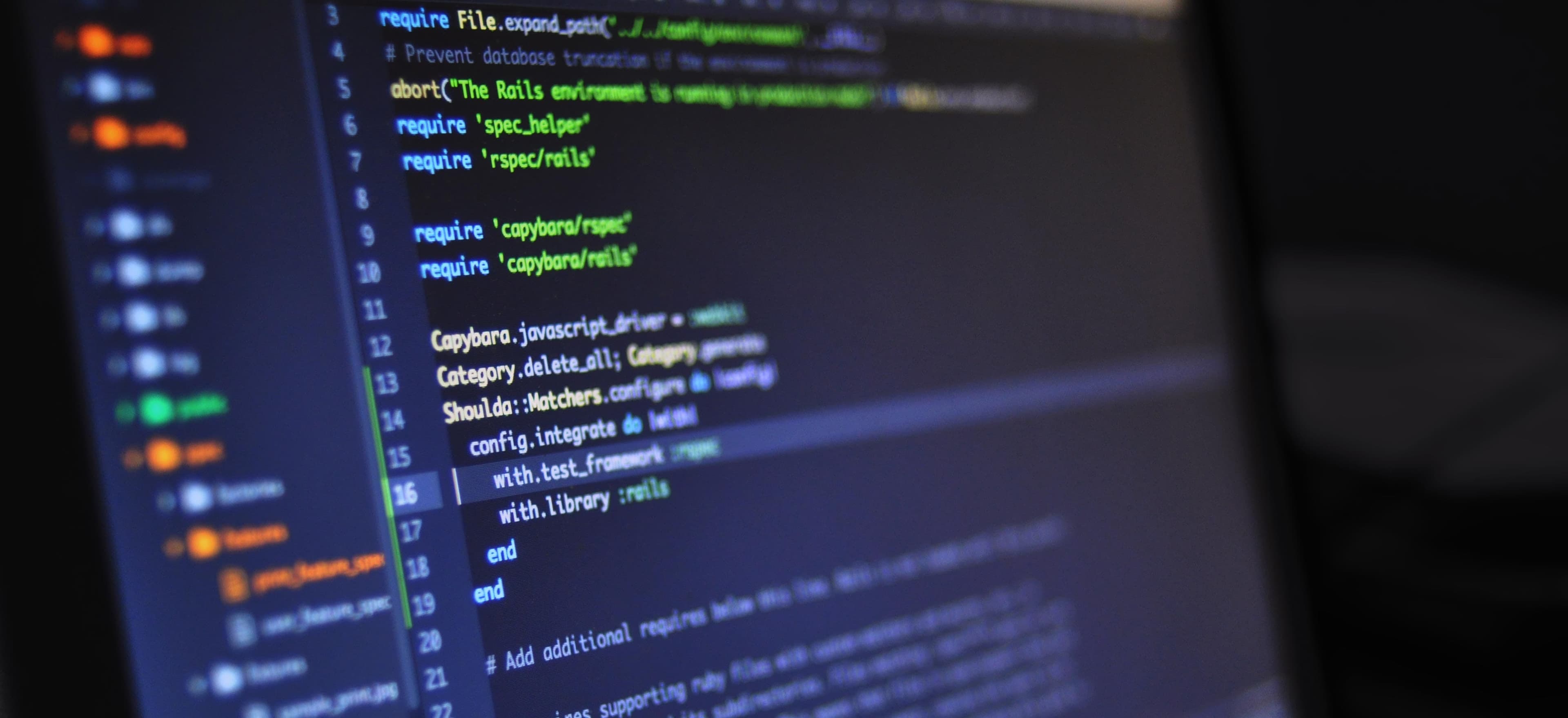
- Published on
Choosing the Best Integration Framework for Spring
When it comes to building enterprise-level applications, integrating multiple systems and components is a common challenge. Spring, with its vast ecosystem, offers several integration options. In this article, we'll explore some of the best integration frameworks for Spring and discuss their strengths and best use cases.
1. Spring Integration
Spring Integration is an extension of the Spring programming model that facilitates the integration of enterprise systems. It provides a set of inbound and outbound adapters to connect with external systems, along with components for message routing, transformation, and handling.
@Bean
public IntegrationFlow fileReadingFlow() {
return IntegrationFlows.from(Files.inboundAdapter(new File("/source"))
.patternFilter("*.txt"),
e -> e.poller(Pollers.fixedDelay(100)))
.handle(System.out::println)
.get();
}
Why it's great: Spring Integration offers seamless integration with the Spring ecosystem, making it an excellent choice for Spring-based applications. Its support for messaging patterns like pub-sub, point-to-point, and request-reply make it suitable for building robust messaging systems.
Best Use Case: Use Spring Integration when building applications that require extensive messaging and system integration capabilities, such as enterprise service buses and event-driven architectures.
2. Apache Camel
Apache Camel is a versatile open-source integration framework that focuses on enterprise integration patterns. It provides a rich set of components and a fluent API for routing and mediating messages.
from("file:sourceFolder?noop=true")
.to("jms:queue:orders");
Why it's great: Apache Camel excels in implementing complex integration scenarios thanks to its extensive support for various protocols and data formats. It also provides a gentle learning curve and a powerful routing engine.
Best Use Case: Use Apache Camel for integrating diverse systems, protocols, and data formats in a robust and maintainable manner. It's suitable for use in scenarios where complex routing and transformation logic are required.
3. MuleSoft
MuleSoft, an integration platform acquired by Salesforce, offers Mule ESB as its flagship product for integrating applications, data, and devices. It provides a powerful visual design environment and a scalable runtime engine for building application networks.
<flow name="httpFlow">
<http:listener config-ref="httpListenerConfig" path="/api/*" />
<logger message="Received HTTP request" level="INFO" />
</flow>
Why it's great: MuleSoft's visual design environment makes it easy to create and manage integration flows without delving deep into code. It also offers a wide range of connectors for seamless integration with various systems and SaaS applications.
Best Use Case: MuleSoft is ideal for large-scale integration projects that require a visual approach to designing integration flows. It's well-suited for enterprises looking for a comprehensive integration platform with support for API-led connectivity.
4. Integration Patterns with Kafka
Apache Kafka, while primarily known as a distributed streaming platform, can also be used for integrating systems and building event-driven architectures. Its support for fault-tolerance and real-time processing makes it suitable for implementing integration patterns.
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", StringSerializer.class.getName());
props.put("value.serializer", StringSerializer.class.getName());
Producer<String, String> producer = new KafkaProducer<>(props);
Why it's great: Kafka's distributed nature and scalability make it a powerful choice for building real-time integration pipelines. Its ability to handle high throughput and low latency makes it suitable for event sourcing and stream processing scenarios.
Best Use Case: Use Kafka for building event-driven architectures and real-time data pipelines. It's well-suited for scenarios that demand high throughput and fault-tolerance in event processing.
In conclusion, the choice of an integration framework for Spring depends on the specific requirements of the project. Spring Integration is a natural fit for Spring-based applications, while Apache Camel excels in complex integration scenarios. MuleSoft offers a visual approach to integration, and Kafka shines in event-driven architectures. By understanding the strengths of each framework, you can make an informed decision to ensure seamless integration within your Spring application.
Remember, the best integration framework is the one that aligns with your project's needs and integrates seamlessly within your existing architecture.
Now, armed with the knowledge of these integration frameworks, you're well-equipped to make an informed decision for your next integration project within a Spring application.
For more in-depth information, you can delve into the rich documentation provided by the respective frameworks:
- Spring Integration Documentation
- Apache Camel Documentation
- MuleSoft Documentation
- Apache Kafka Documentation