Boosting Java Performance for Native-Like Speed
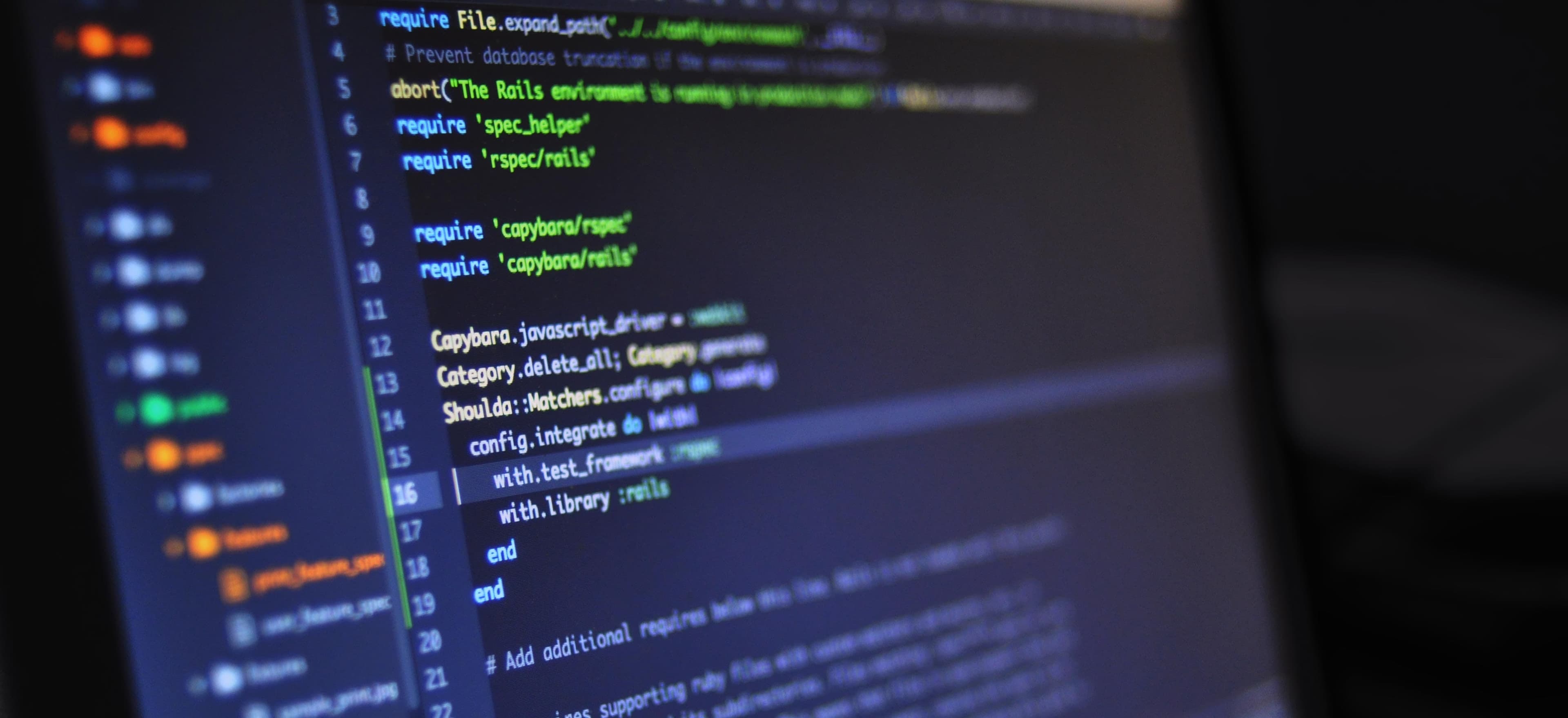
- Published on
Boosting Java Performance for Native-Like Speed
Java is one of the most popular programming languages, known for its portability and performance. However, achieving native-like speed in Java can be challenging. In this article, we will explore various techniques to optimize Java performance, allowing your applications to run faster and more efficiently.
1. Use Primitive Data Types
Java provides wrapper classes for primitive data types such as Integer
, Double
, and Boolean
. While these wrapper classes offer additional functionality, they come with the cost of increased memory consumption and slower performance due to autoboxing and unboxing.
// Using primitive type
int num = 10;
// Using wrapper class
Integer num = 10;
By utilizing primitive data types, you can reduce memory overhead and improve performance, especially in performance-critical code.
2. Employ StringBuilder for String Concatenation
String concatenation using the +
operator can result in performance overhead, especially when dealing with large strings or performing concatenation in a loop. This is because strings are immutable in Java, so each concatenation operation creates a new string object.
String result = "";
for (int i = 0; i < 1000; i++) {
result += "value" + i;
}
Instead, using StringBuilder
is more efficient for string concatenation, as it does not create new string objects for each operation.
StringBuilder result = new StringBuilder();
for (int i = 0; i < 1000; i++) {
result.append("value").append(i);
}
3. Leverage Just-In-Time (JIT) Compiler
The JIT compiler in Java optimizes the performance of bytecode at runtime by compiling it to native machine code. It identifies and hotspots the code, and optimizes it for better performance.
You can enable JIT compiler optimizations using the following JVM flags:
java -XX:+AggressiveOpts -XX:+UseFastAccessorMethods YourApplication
4. Use Efficient Data Structures and Algorithms
Choosing the right data structures and algorithms is crucial for achieving optimal performance in Java. For example, using HashMap
instead of Hashtable
for better performance, or employing efficient sorting algorithms such as Quicksort or Mergesort when dealing with large datasets.
// Using HashMap
Map<String, Integer> map = new HashMap<>();
// Using Hashtable
Hashtable<String, Integer> table = new Hashtable<>();
5. Minimize Synchronization Overhead
Excessive synchronization can introduce performance overhead in multi-threaded applications. While synchronization is necessary for thread safety, it's essential to minimize its usage and consider alternative thread-safe data structures such as ConcurrentHashMap
or CopyOnWriteArrayList
in performance-critical scenarios.
// Using ConcurrentHashMap
Map<String, Integer> map = new ConcurrentHashMap<>();
6. Profile and Optimize Critical Code Paths
Profiling your application using tools like JProfiler or VisualVM can help identify performance bottlenecks and hotspots. Once identified, you can optimize the critical code paths by employing techniques such as caching frequently accessed data, reducing unnecessary object creation, and refactoring inefficient algorithms.
7. Utilize Compilation Optimization
Modern Java compilers come with advanced optimization techniques that can significantly improve performance. For instance, the Java 9 introduced Ahead-of-Time (AOT) compilation with the GraalVM, allowing you to compile Java code ahead of time for improved startup time and reduced memory footprint.
/**
* GraalVM AOT compilation
*/
public class Main {
public static void main(String[] args) {
System.out.println("Hello, GraalVM!");
}
}
The Bottom Line
In conclusion, optimizing Java performance for native-like speed involves various strategies, including using primitive data types, efficient data structures, JIT compiler, and profiling critical code paths. By applying these techniques, you can significantly enhance the performance of your Java applications, making them run faster and more efficiently.
By implementing these tips, your Java applications can achieve performance levels closer to that of native applications, providing a seamless and efficient user experience.
Remember, achieving optimal performance is an ongoing process that requires continuous monitoring, benchmarking, and optimization to adapt to changing requirements and environments.
Start optimizing your Java applications today and experience the power of native-like speed!
Remember, for more information on Java performance optimization, be sure to visit Oracle's official Java documentation and Baeldung's Java Performance Tuning Guide.