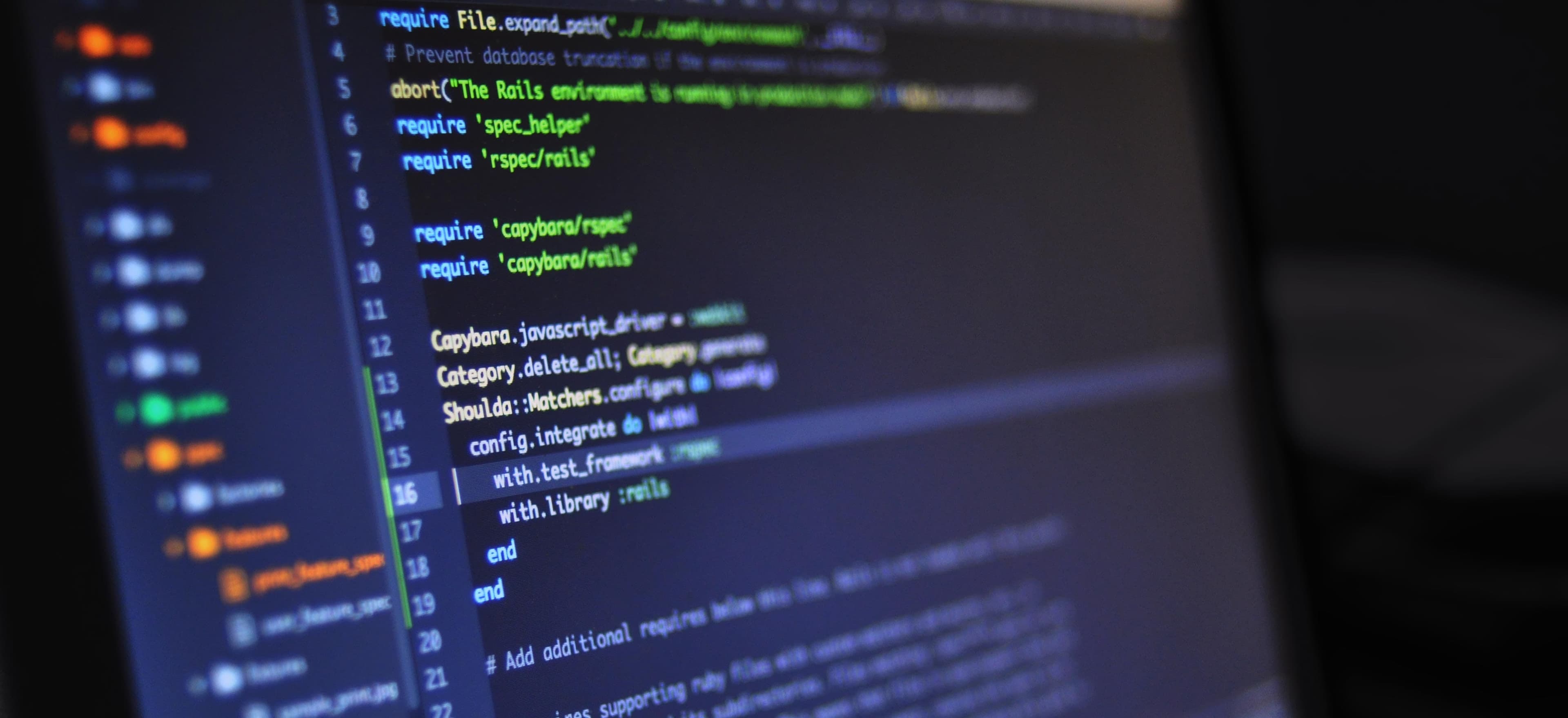
- Published on
Integrating Weather Data on Android Google Maps
When it comes to developing Android applications, integrating various APIs can significantly enhance user experience and add value to the app. In this article, we will explore the process of integrating weather data onto Google Maps in an Android app using Java. We'll leverage the OpenWeatherMap API to retrieve weather information based on geographical coordinates and display this information on the Google Map markers.
Prerequisites
Before diving into the implementation, it's essential to have the following set up:
- Android Studio
- Basic knowledge of Android development
- OpenWeatherMap API key (sign up here if you don't have one)
- Google Maps API key (obtain it from the Google Cloud Console)
Setting up the Project
-
Create a new Android Studio project.
-
Add the necessary permissions in the
AndroidManifest.xml
file for internet access:<uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
-
Ensure that the required dependencies are included in the
build.gradle
file:
dependencies {
implementation 'com.google.android.gms:play-services-maps:18.0.0'
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
}
Implementing the Weather Service
We'll create a service to interact with the OpenWeatherMap API and fetch weather data based on the coordinates.
Create the Model Class
Let's start by creating a Weather
model class to represent the retrieved weather information:
public class Weather {
private String main;
private String description;
// Other relevant fields
// Getters and setters
}
Implement Retrofit Interface
Next, we'll define the Retrofit interface with a method to fetch weather data:
public interface WeatherService {
@GET("weather")
Call<Weather> getWeatherData(@Query("lat") double latitude, @Query("lon") double longitude, @Query("appid") String apiKey);
}
Ensure to replace "YOUR_API_KEY"
with your OpenWeatherMap API key.
Create Weather API Client
Now, let's create a class to handle API requests and response handling using Retrofit:
public class WeatherApiClient {
private static final String BASE_URL = "https://api.openweathermap.org/data/2.5/";
private Retrofit retrofit;
public WeatherApiClient() {
retrofit = new Retrofit.Builder()
.baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.build();
}
public WeatherService getWeatherService() {
return retrofit.create(WeatherService.class);
}
}
Adding Weather Data to Google Maps
After fetching the weather information, we can display it on the Google Map markers.
Get Current Location
First, we need to obtain the user's current location. This can be achieved using the Fused Location Provider API.
Add Markers with Weather Information
Once we have the user's location, we can fetch the weather data using the created service and add markers with weather information to the Google Map:
@Override
public void onMapReady(GoogleMap googleMap) {
mMap = googleMap;
// Add a marker with weather information
WeatherService weatherService = new WeatherApiClient().getWeatherService();
Call<Weather> call = weatherService.getWeatherData(latitude, longitude, "YOUR_API_KEY");
call.enqueue(new Callback<Weather>() {
@Override
public void onResponse(Call<Weather> call, Response<Weather> response) {
if (response.isSuccessful() && response.body() != null) {
Weather weather = response.body();
// Add marker with weather details
mMap.addMarker(new MarkerOptions().position(new LatLng(latitude, longitude))
.title("Weather: " + weather.getMain())
.snippet("Description: " + weather.getDescription()));
}
}
@Override
public void onFailure(Call<Weather> call, Throwable t) {
// Handle failure
}
});
}
In Conclusion, Here is What Matters
In this tutorial, we have explored the process of integrating weather data onto Google Maps in an Android app using Java. By leveraging the OpenWeatherMap API and Google Maps SDK, we can provide users with real-time weather information right on the map. This not only enhances user experience but also adds practical value to the application. Be sure to handle errors and edge cases effectively for a robust implementation.
By following these steps, you can enrich your Android app with dynamic weather data, creating a more engaging and informative experience for users. Happy coding!