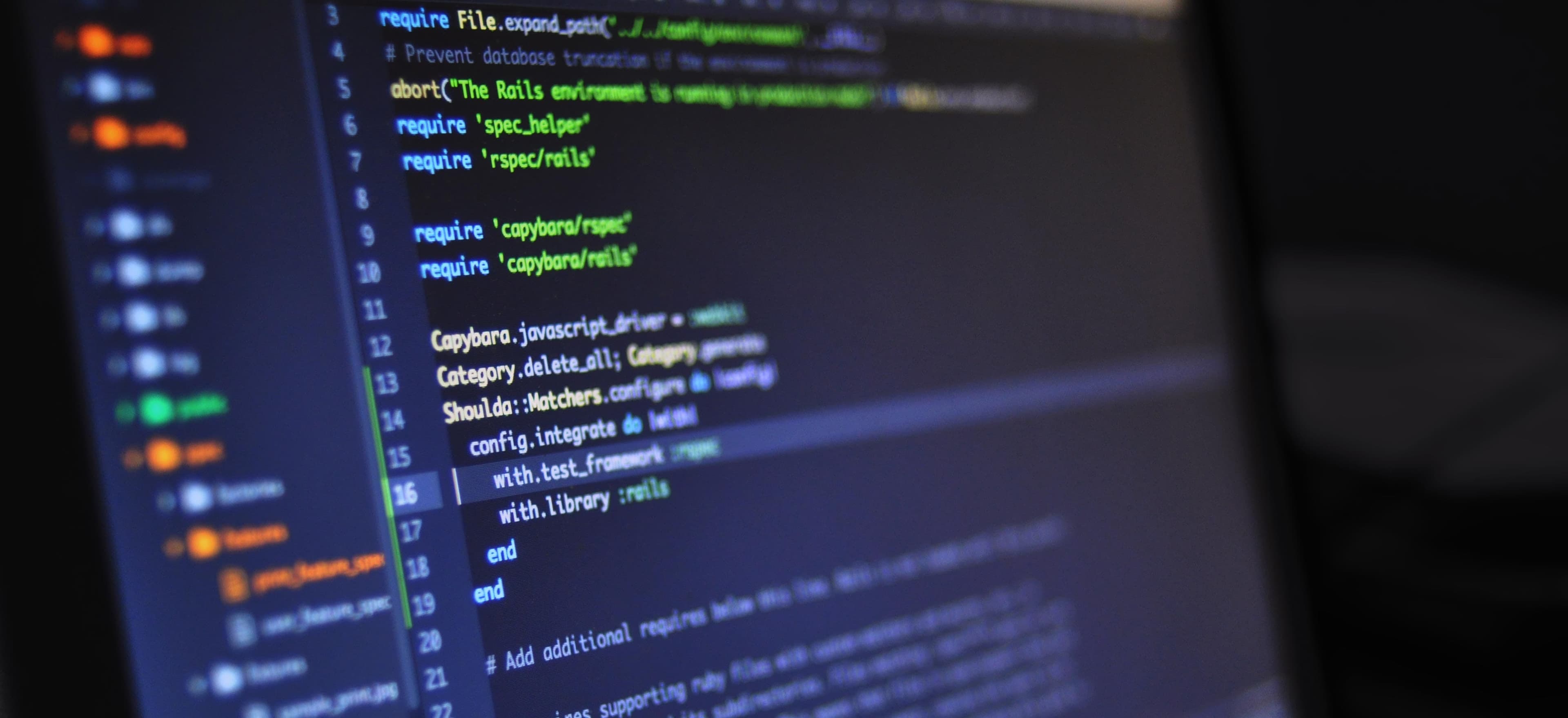
- Published on
Implementing Sustainable Database Changes in Java
In the world of application development, making changes to a database is an inevitable part of the process. Whether it’s adding new features, optimizing performance, or fixing bugs, developers need to apply changes to the database schema. However, making these changes in a way that ensures data integrity, performance, and maintainability can be quite challenging. In this blog post, we will explore how to implement sustainable database changes in Java applications.
The Challenge of Database Changes
When it comes to making changes to the database schema, there are several challenges that developers often face:
Data Integrity
Maintaining data integrity is crucial when applying database schema changes. Incorrectly applied changes can lead to data corruption, loss of data, or inconsistent data state, causing issues for the application and its users.
Performance
Poorly executed database changes can have a significant impact on the performance of the application. Long-running, resource-intensive queries can degrade the overall performance of the system.
Maintainability
As the application evolves, the database schema needs to evolve as well. It’s important to make sure that database changes are easily maintainable and do not introduce unnecessary complexity.
Using Java for Database Changes
Java is a popular and powerful programming language used for building a wide range of applications, including those with complex database requirements. When it comes to implementing sustainable database changes, Java provides a rich ecosystem of tools and frameworks to help developers address the challenges mentioned above.
JDBC
Java Database Connectivity (JDBC) is a standard API for connecting Java applications to a database. It provides a way to execute SQL statements, retrieve results, and manage database connections. When implementing database changes in Java, JDBC can be used to execute DDL (Data Definition Language) statements for altering the database schema.
try (Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement()) {
statement.execute("ALTER TABLE my_table ADD COLUMN new_column VARCHAR(255)");
} catch (SQLException e) {
// Handle the exception
}
In the code above, we use JDBC to establish a connection to the database and execute an ALTER TABLE statement to add a new column to the 'my_table' table.
JPA and Hibernate
Java Persistence API (JPA) is a standard interface for Java applications to interact with databases using object-relational mapping (ORM). Hibernate is a popular JPA implementation that provides additional features for managing database schema changes.
@Entity
@Table(name = "my_table")
public class MyEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
// Other entity fields and methods
}
In this example, we have a JPA entity mapped to the 'my_table' table. When making changes to the database schema, Hibernate provides mechanisms to generate and execute DDL statements based on entity mappings.
Liquibase and Flyway
Liquibase and Flyway are popular open-source database migration tools that help manage and apply database changes in a controlled and sustainable manner. These tools provide features for versioning, executing, and tracking database schema changes through migration scripts.
<changeSet id="add-new-column" author="yourname">
<addColumn tableName="my_table">
<column name="new_column" type="VARCHAR(255)"/>
</addColumn>
</changeSet>
The above example shows a Liquibase changeset that adds a new column to the 'my_table' table. By using migration tools like Liquibase or Flyway, database schema changes can be tracked, versioned, and applied consistently across different environments.
Best Practices for Sustainable Database Changes
When implementing database changes in Java applications, it's important to follow best practices to ensure sustainability and maintainability.
Use Automated Tests
Automated tests play a crucial role in validating that database changes do not introduce regressions or disrupt the application’s functionality. Integration tests that cover database interactions should be written to verify the behavior of the application after applying schema changes.
Leverage Version Control
Database migration scripts should be treated as first-class citizens in version control alongside application code. This ensures that database changes are tracked, reviewed, and deployed in sync with application updates.
Document Database Changes
Maintaining documentation for database schema changes is essential for understanding the evolution of the database over time. Documenting the purpose and impact of each change helps in maintaining a clear history of database modifications.
Perform Rollback Planning
In the event of a failed database change, having a rollback plan is crucial. It’s important to consider how to revert the changes and ensure that data integrity is preserved during the rollback process.
A Final Look
Implementing sustainable database changes in Java applications is a critical aspect of software development. By leveraging Java’s rich ecosystem of database tools and frameworks, and following best practices for managing database changes, developers can ensure that their applications maintain data integrity, performance, and maintainability throughout their lifecycle.
As businesses continue to rely on efficient and effective data management, the importance of implementing sustainable database changes will only grow. Embracing the best practices and tools available in the Java ecosystem will be essential for developers to meet these evolving demands and deliver robust, reliable applications.
To learn more about sustainable database changes in Java, you can explore Hibernate, an industry-leading JPA implementation, and Liquibase, a powerful database migration tool that can greatly facilitate sustainable database changes. Happy coding!
Checkout our other articles