Optimizing CI/CD Pipeline for Apigee API Proxies
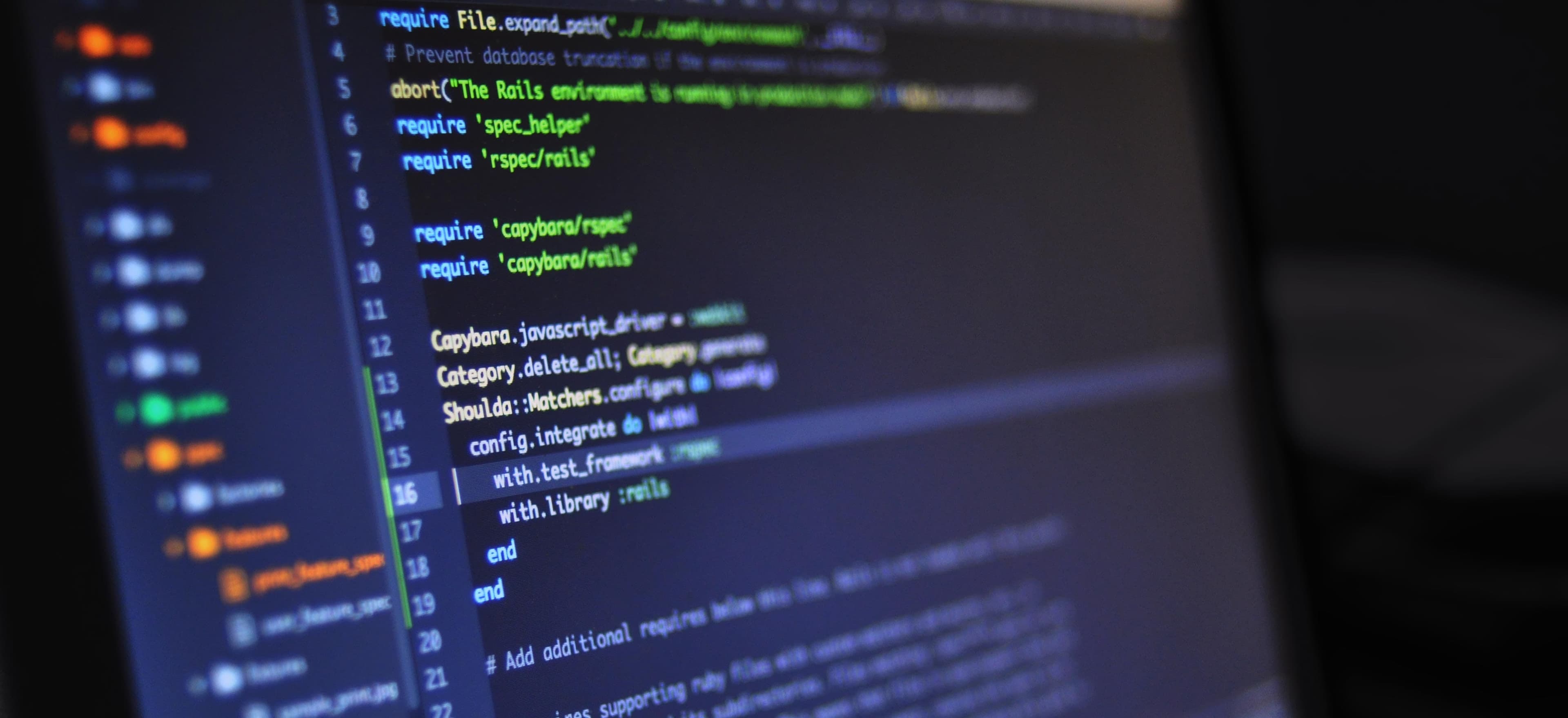
- Published on
Optimizing CI/CD Pipeline for Apigee API Proxies
In today's fast-paced software development environment, having a robust Continuous Integration/Continuous Deployment (CI/CD) pipeline is crucial for efficiently delivering high-quality software. This is especially true for API proxies built on the Apigee platform, where changes need to be deployed quickly and reliably. In this article, we'll explore how to optimize the CI/CD pipeline for Apigee API proxies using Java and best practices.
Understanding Apigee API Proxies
Before diving into the CI/CD pipeline optimization, let's briefly understand what Apigee API proxies are. Apigee is a leading API management platform that allows organizations to design, secure, deploy, monitor, and scale APIs. API proxies in Apigee act as intermediaries between the clients and the backend services, providing features such as security, caching, rate limiting, and more.
Implementing CI/CD for Apigee API Proxies
Setting Up the Development Environment
The first step in optimizing the CI/CD pipeline for Apigee API proxies is to set up the development environment. This typically involves using a version control system such as Git, and a build tool such as Maven or Gradle. Additionally, you'll need the Apigee Edge Maven plugin, which provides seamless integration between Apigee Edge and Maven.
Here's an example of a pom.xml
file with the Apigee Edge Maven plugin configuration:
<plugins>
<plugin>
<groupId>com.apigee.tools</groupId>
<artifactId>apigee-edge-maven-plugin</artifactId>
<version>1.0.0</version>
<configuration>
<org>${org}</org>
<api>${api}</api>
<environment>${env}</environment>
<username>${username}</username>
<password>${password}</password>
<options>${options}</options>
<delay>${delay}</delay>
</configuration>
</plugin>
</plugins>
In the above configuration, ${org}
, ${api}
, ${env}
, ${username}
, ${password}
, ${options}
, and ${delay}
are placeholders for the organization, API proxy name, environment, Apigee username, password, additional options, and delay, respectively.
Building and Testing the API Proxies
Once the development environment is set up, you can start building and testing the API proxies locally. Using Java, you can write JUnit tests for your proxy logic to ensure that it behaves as expected. This is a critical step in the CI/CD pipeline, as it helps catch any issues early in the development cycle.
Here's an example of a JUnit test for an Apigee API proxy written in Java:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MyApiProxyTest {
@Test
public void testProxyLogic() {
// Mock input and expected output
String input = "some input";
String expectedOutput = "some output";
// Call the proxy logic
String actualOutput = MyApiProxy.process(input);
// Assert the expected output
assertEquals(expectedOutput, actualOutput);
}
}
In the above test, we're mocking the input and the expected output of the API proxy logic and asserting that the actual output matches the expected output.
Integrating with CI/CD Tools
The next step is to integrate the API proxy code with your CI/CD tools, such as Jenkins, CircleCI, or any other preferred tool. This involves configuring your CI/CD pipeline to trigger builds, run tests, and deploy the API proxies to the Apigee Edge platform.
For example, in a Jenkins pipeline, you can use the Apigee Edge Maven plugin to deploy the API proxies:
apigee-edge:deployProxy
This command triggers the deployment of the API proxy to the specified environment in Apigee Edge.
Continuous Deployment and Monitoring
With the CI/CD pipeline set up, changes to the API proxies can be continuously deployed to the Apigee Edge platform. It's important to closely monitor the deployments to ensure that they are successful and do not introduce any issues into the production environment.
Tools such as Apigee Edge's built-in monitoring features, along with custom monitoring solutions, can be used to monitor the API proxies for performance, reliability, and security.
Wrapping Up
Optimizing the CI/CD pipeline for Apigee API proxies is essential for ensuring fast, reliable, and high-quality deployments. By leveraging Java and best practices in software development, you can streamline the process of building, testing, and deploying API proxies, ultimately improving the agility and efficiency of your API development team.
In conclusion, implementing a well-structured CI/CD pipeline, integrating with CI/CD tools, and continuously monitoring deployments are crucial steps in optimizing the development and deployment of Apigee API proxies using Java.
For further reading on optimizing CI/CD pipelines for Apigee, you can refer to the official documentation. Additionally, exploring best practices in CI/CD implementation for API management platforms will provide valuable insights for optimizing your workflows.
Checkout our other articles