Overcoming Data Privacy Challenges in IoT with TensorFlow
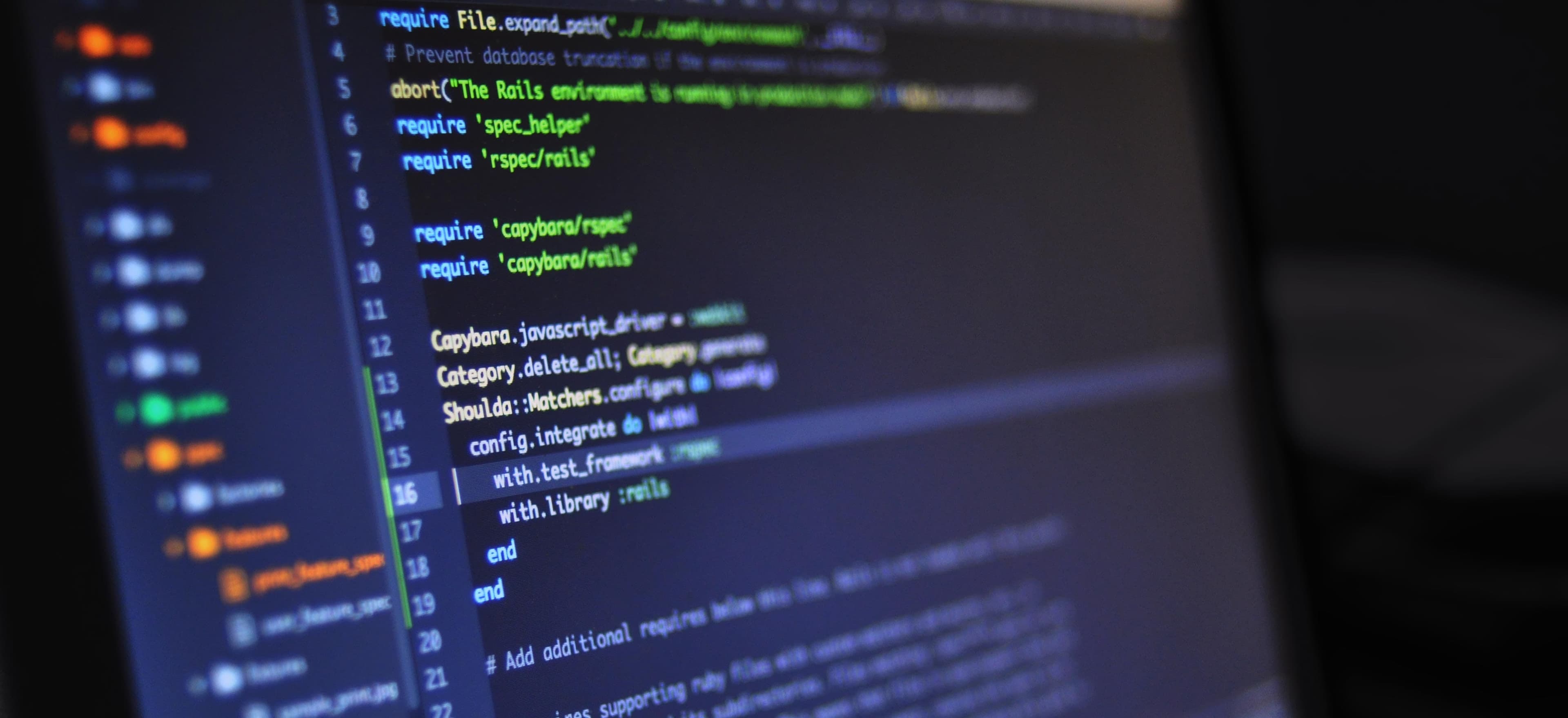
- Published on
Overcoming Data Privacy Challenges in IoT with TensorFlow
As the Internet of Things (IoT) continues to rapidly expand, the need to process and analyze large volumes of sensitive data at the edge has become increasingly critical. However, this also raises significant concerns about data privacy and security. In this article, we will explore how TensorFlow, a popular open-source machine learning framework, can be leveraged to address data privacy challenges in IoT environments.
Understanding the Data Privacy Concerns in IoT
IoT devices are capable of collecting a vast array of data, ranging from personal health information to industrial production metrics. This wealth of sensitive data presents a prime target for malicious entities. Additionally, in scenarios where data is transmitted to central servers for processing, there is an inherent risk of interception during transmission. Therefore, ensuring data privacy in IoT systems is a paramount concern.
Utilizing Federated Learning with TensorFlow for Privacy-Preserving IoT
TensorFlow's federated learning offers a compelling solution to the data privacy challenges in IoT. By enabling model training to be conducted on the edge devices themselves, federated learning reduces the need for centralized data collection. This decentralized approach not only minimizes the risk of data exposure but also empowers individual devices to collaboratively learn without sharing raw data.
Let's delve into an example of implementing federated learning using TensorFlow in an IoT scenario.
Setting Up the TensorFlow Federated Environment
import org.tensorflow.*;
import org.tensorflow.lite.Interpreter;
import org.tensorflow.lite.support.label.TensorLabel;
import org.tensorflow.lite.support.tensorbuffer.TensorBuffer;
In this example, we import the necessary TensorFlow libraries to establish the federated learning environment within the IoT framework.
Implementing Federated Averaging
public class FederatedAveraging {
public void performFederatedAveraging(List<Device> devices, Model globalModel) {
for (Device device : devices) {
Model localModel = device.trainLocalModel();
device.sendModelUpdate(localModel);
}
Model federatedModel = globalModel.aggregateModelUpdates(devices);
globalModel.updateModelParams(federatedModel);
}
}
Here, we define a FederatedAveraging
class in Java to orchestrate the federated learning process. The performFederatedAveraging
method iterates through the connected IoT devices, allowing each device to train its local model and send updates to the global model. The global model then aggregates the updates from all devices, resulting in a federated model with refined parameters.
By employing federated learning with TensorFlow, IoT deployments can mitigate privacy risks associated with centralized data processing, while also preserving the confidentiality of sensitive information.
Leveraging Differential Privacy Techniques
In addition to federated learning, TensorFlow provides support for differential privacy, which adds noise to the learning process to prevent the extraction of individual records from the training data. This technique proves particularly effective in scenarios where fine-grained privacy protection is required, such as medical IoT devices.
Introducing Differential Privacy Measures with TensorFlow
import org.tensorflow.privacy.analysis.StatisticalTests;
import org.tensorflow.privacy.analysis.privacy_ledger.PrivacyLedger;
PrivacyLedger privacyLedger = new PrivacyLedger();
double epsilon = 0.5;
privacyLedger.incrementEpsilon(epsilon);
boolean isPrivate = StatisticalTests.isDifferentiallyPrivate(privacyLedger);
In the provided code snippet, we instantiate a PrivacyLedger
and increment the privacy budget (epsilon). The StatisticalTests
class from TensorFlow's privacy module enables us to verify if the learning process satisfies differential privacy guarantees.
The introduction of differential privacy mechanisms, alongside federated learning, fortifies the data privacy measures within IoT ecosystems, instilling greater confidence in handling sensitive information.
Final Thoughts
In the realm of IoT, preserving data privacy is an ongoing challenge, necessitating robust solutions to mitigate potential risks. With TensorFlow's federated learning and differential privacy capabilities, organizations can proactively address the security and privacy concerns associated with IoT data processing. By decentralizing model training and incorporating differential privacy measures, TensorFlow empowers IoT deployments to uphold stringent data privacy standards, fostering trust and reliability in the burgeoning landscape of connected devices.
To delve deeper into the intricate concepts of TensorFlow, federated learning, and differential privacy, consider exploring the TensorFlow documentation and Google's Differential Privacy Overview for comprehensive insights.
In conclusion, as the IoT domain continues to evolve, TensorFlow stands as a steadfast ally in navigating the intricacies of data privacy, equipping organizations with the tools needed to navigate the data privacy landscape with confidence.