Enriching Apache Avro Classes with Custom Annotations
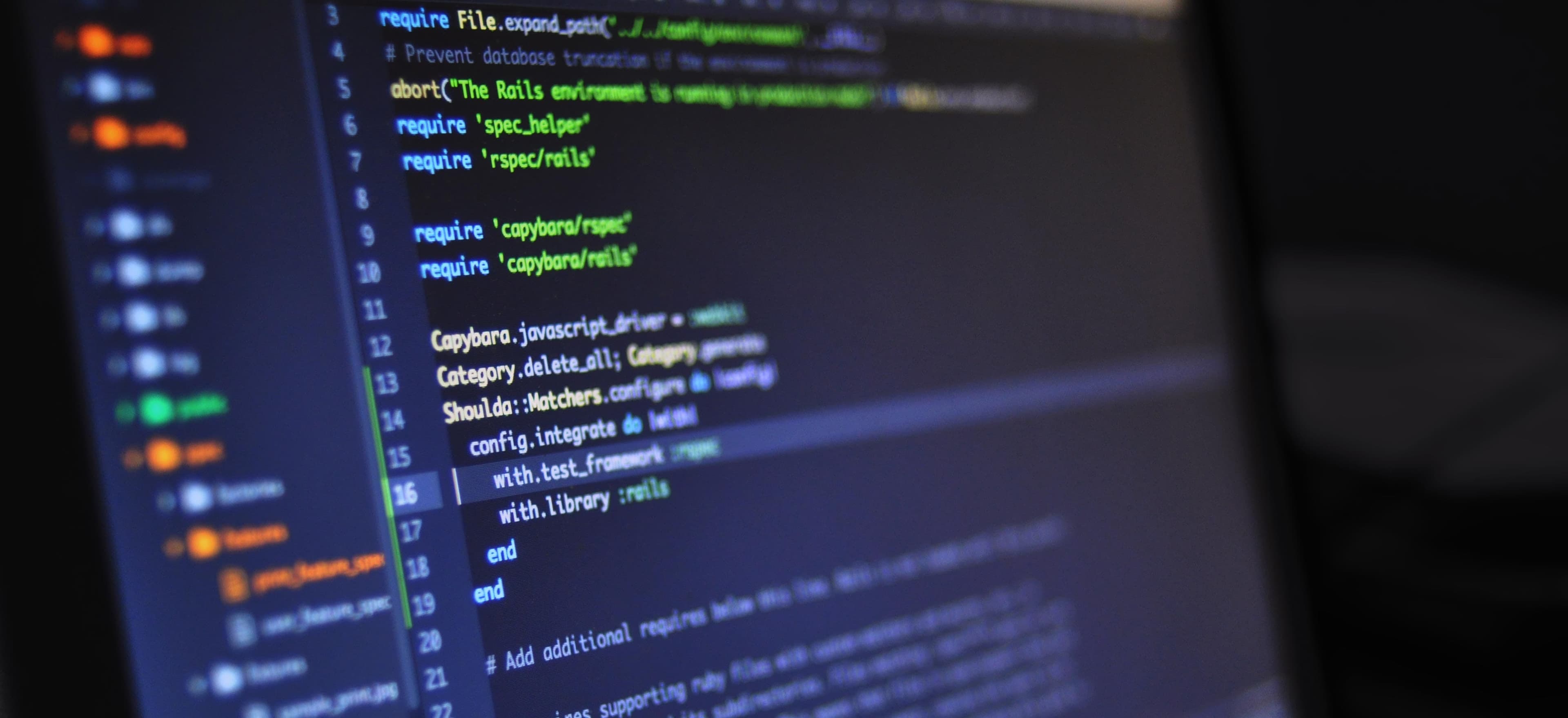
- Published on
Enriching Apache Avro Classes with Custom Annotations
Apache Avro is a popular data serialization system that provides rich, efficient data serialization coupled with code generation for Java. It's widely used in big data processing frameworks such as Apache Hadoop, Apache Kafka, and more. Avro allows for schema evolution, which means that data can be read by any process using the same schema.
In this blog post, we'll explore how to enhance Avro classes with custom annotations. This will enable us to add metadata to the Avro schema, enriching it with additional information that can be beneficial in various scenarios.
Why Use Custom Annotations in Avro Classes?
Custom annotations offer a clean and concise way to enrich Avro classes with supplemental metadata. This additional metadata can be utilized in a variety of ways, such as guiding serialization and deserialization processes, validation mechanisms, documentation generation, and more.
Setting Up the Project
To get started, let's create a new Maven project and add the necessary dependencies for working with Apache Avro.
<dependencies>
<dependency>
<groupId>org.apache.avro</groupId>
<artifactId>avro</artifactId>
<version>1.10.2</version>
</dependency>
</dependencies>
Creating a Custom Annotation
We'll begin by creating a custom annotation that can be applied to fields in our Avro classes. Let's define an annotation called @AvroMetadata
which will allow us to add metadata to the Avro schema.
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface AvroMetadata {
String value();
}
In this example, we've defined an annotation @AvroMetadata
that can be applied to fields. The annotation carries a single element value
, which can hold the metadata we want to associate with the field.
Using the Custom Annotation in an Avro Class
Let's now create an Avro class User
and apply our custom annotation to one of its fields.
import org.apache.avro.reflect.AvroDefault;
import org.apache.avro.reflect.Nullable;
import org.apache.avro.reflect.Nullable;
public class User {
private String name;
@AvroMetadata("This field stores the user's age")
private int age;
// Getters and setters omitted for brevity
}
Here, we've applied our @AvroMetadata
annotation to the age
field of the User
class, providing a meaningful description of the field's purpose.
Generating Avro Schema with Custom Annotation Metadata
When generating the Avro schema from the User
class, we can utilize the metadata provided by our custom annotation.
import org.apache.avro.Schema;
import org.apache.avro.reflect.ReflectData;
public class Main {
public static void main(String[] args) {
Schema schema = ReflectData.get().getSchema(User.class);
System.out.println(schema.toString(true));
}
}
When we run the above code, the output will include the metadata from our custom annotation in the Avro schema definition for the User
class.
Final Considerations
In this blog post, we've explored how to enrich Apache Avro classes with custom annotations to add metadata to the Avro schema. Custom annotations provide a flexible way to incorporate additional information into Avro classes, enhancing their functionality and usability in various scenarios.
By leveraging custom annotations, we can improve the documentation of Avro schemas, guide serialization and deserialization processes, and enable validation mechanisms based on the annotated metadata.
Custom annotations add a layer of versatility to Apache Avro, empowering developers to enhance their data serialization and schema evolution workflows.
To learn more about Apache Avro and its capabilities, explore the official Avro documentation.
Start enriching your Avro classes with custom annotations today and unlock a new dimension of flexibility and information within your data serialization processes!