Troubleshooting Common Errors in ANTLR
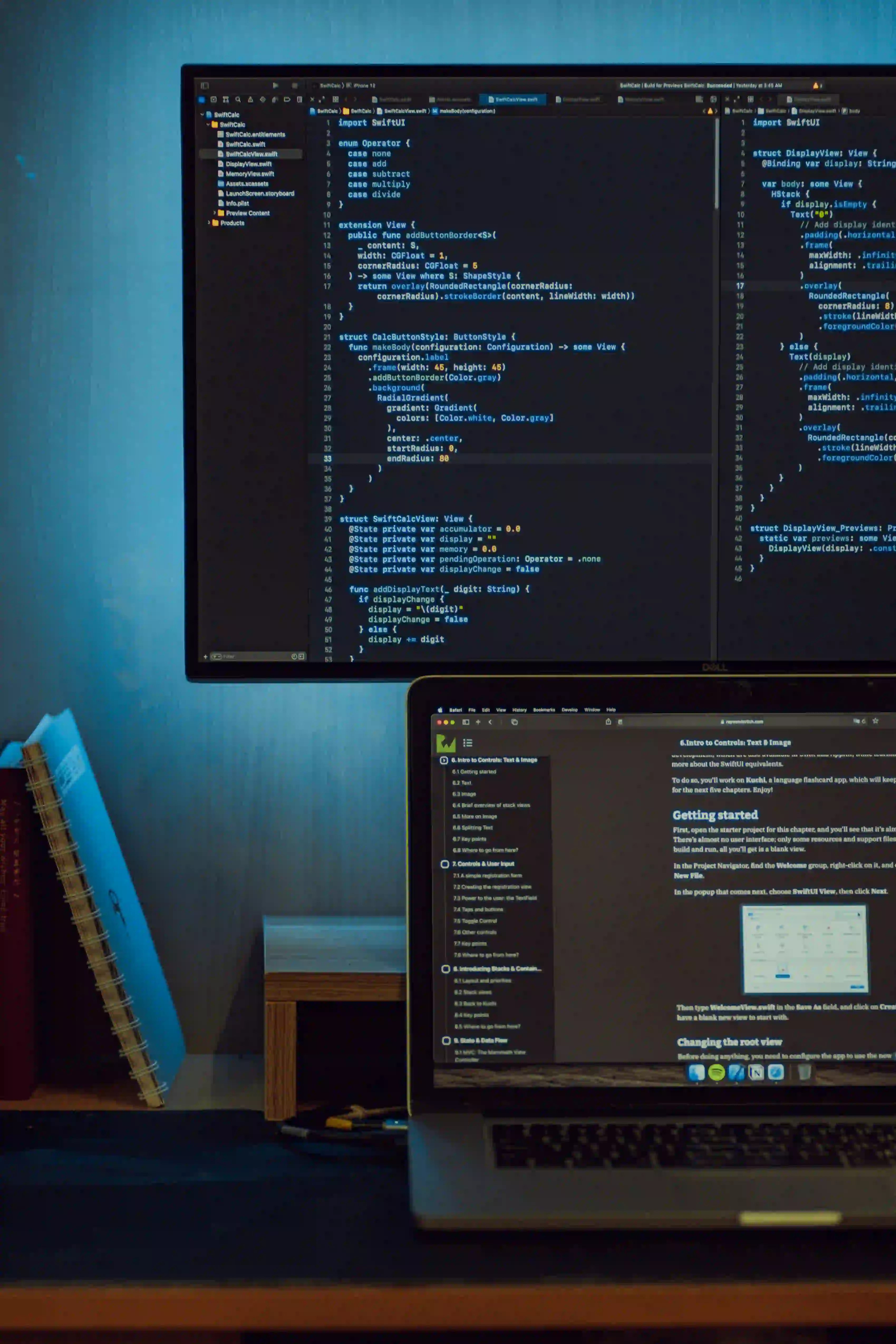
Troubleshooting Common Errors in ANTLR
ANTLR (ANother Tool for Language Recognition) is a powerful parser generator for reading, processing, executing, or translating structured text or binary files. However, like any other complex tool, it can sometimes throw errors that can puzzle even seasoned developers. In this article, we will explore some common errors that occur while working with ANTLR and how to troubleshoot them.
1. NoViableAltException
This error is one of the most common errors encountered while working with ANTLR grammars. It indicates that the parser encountered a token that was not expected at that point in the parsing process. This can happen due to a missing token in the grammar rules or an issue with the tokenization process. To troubleshoot this error:
-
Review Your Grammar Rules: Double-check your grammar rules to ensure that all tokens are properly defined and used in the correct context.
-
Check Tokenization: Verify that the lexer is tokenizing the input correctly. Use ANTLR's built-in debugging capabilities to visualize the token stream and ensure that the tokens are being generated as expected.
// Example of using ANTLR's built-in debugging capabilities
CharStream input = CharStreams.fromString("your_input");
YourLexer lexer = new YourLexer(input);
CommonTokenStream tokens = new CommonTokenStream(lexer);
tokens.fill();
System.out.println(tokens.getTokens());
- Use ANTLRWorks: ANTLRWorks is a powerful IDE for ANTLR development. Utilize its debugging features to step through the parsing process and identify where the unexpected token is being encountered.
2. MismatchedTokenException
This error occurs when the parser encounters a token that does not match what was expected at that point in the parsing process. To troubleshoot this error:
-
Check Your Grammar Rules: Review your grammar rules to ensure that the expected tokens are defined correctly. Pay close attention to token literals and token references.
-
Inspect Tokenization: Verify that the input is being tokenized correctly and that the tokens are being matched against the grammar rules accurately. Debug the tokenization process to identify any discrepancies.
// Debug the tokenization process
CharStream input = CharStreams.fromString("your_input");
YourLexer lexer = new YourLexer(input);
CommonTokenStream tokens = new CommonTokenStream(lexer);
tokens.fill();
for (Token token : tokens.getTokens()) {
System.out.println(token);
}
- Use ANTLRWorks: Once again, ANTLRWorks can be an invaluable tool for stepping through the parsing process and pinpointing where the token mismatch occurs.
3. RecognitionError
RecognitionError indicates a general recognition problem with input. This can encompass a wide range of issues, such as syntax errors, tokenization problems, or semantic errors within the grammar. To troubleshoot this error:
-
Review Syntax Errors: Check for any syntax errors in the input that may be causing the recognition problem. Ensure that the input adheres to the grammar rules defined in your ANTLR grammar.
-
Debug Tokenization: Debug the tokenization process as mentioned earlier to identify any issues with token generation and matching.
-
Use ANTLR's Error Handling: Implement custom error handling in your parser to provide more descriptive error messages. This can aid in identifying the specific problem with the input.
// Example of custom error handling in ANTLR parser
@Override
public void reportError(RecognitionException e) {
throw new YourCustomRecognitionException("Recognition error: " + e.getMessage());
}
4. Custom Error Handling
Implementing custom error handling in your ANTLR parser can greatly assist in troubleshooting and providing better feedback for recognition issues. By overriding ANTLR's error reporting methods, you can customize error messages and handle specific error scenarios more effectively.
// Example of custom error handling in ANTLR parser
@Override
public void reportError(RecognitionException e) {
throw new YourCustomRecognitionException("Recognition error: " + e.getMessage());
}
Lessons Learned
In conclusion, troubleshooting errors in ANTLR can be a challenging task, but by thoroughly reviewing grammar rules, inspecting tokenization, utilizing debugging tools like ANTLRWorks, and implementing custom error handling, many issues can be swiftly identified and resolved. Understanding the common errors and how to troubleshoot them is crucial for harnessing the full power of ANTLR in language recognition and parsing tasks.
Hopefully, this guide has provided valuable insights into handling common ANTLR errors and will aid you in efficiently navigating through any obstacles you may encounter in your ANTLR projects.