Effective Techniques for Regression Testing
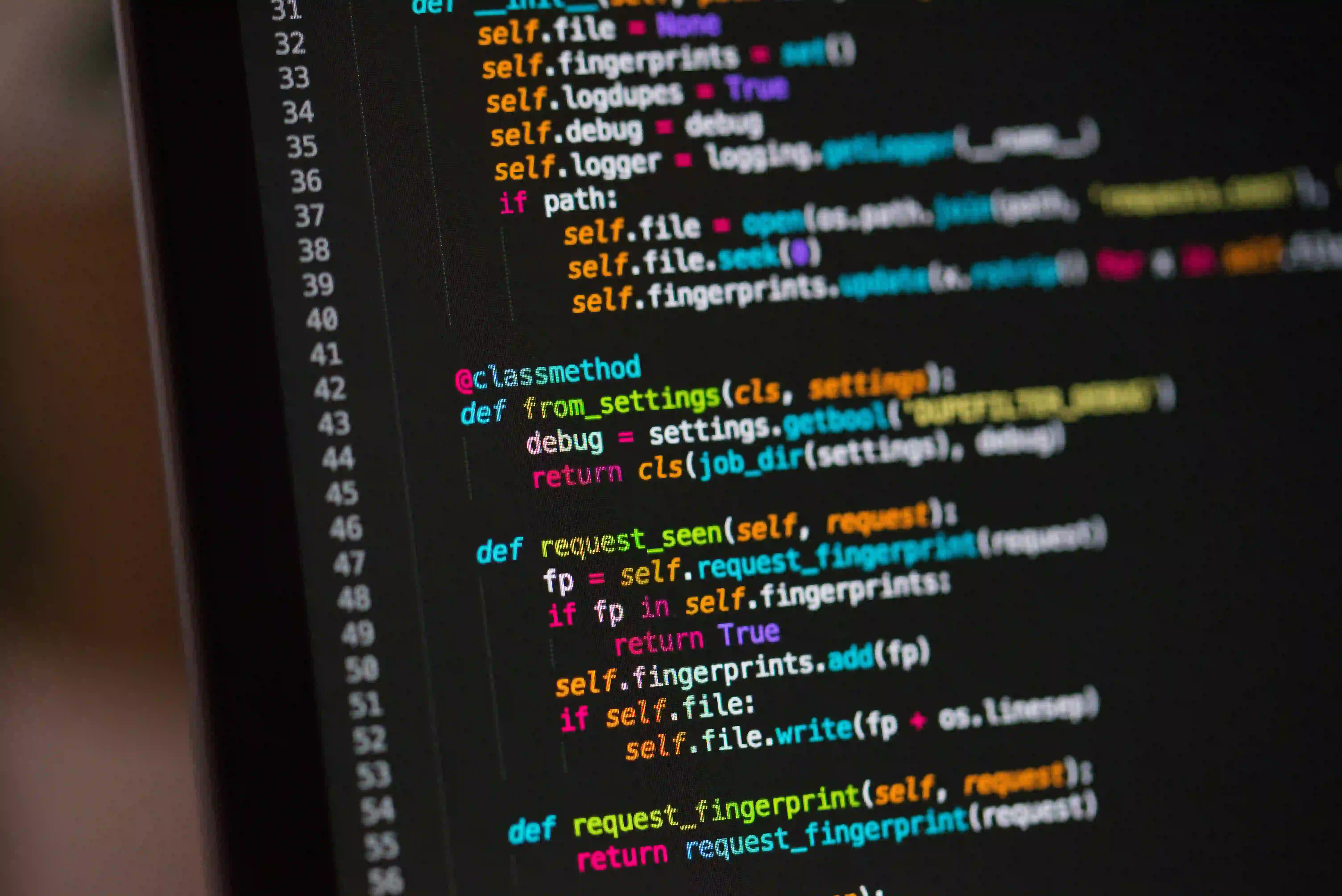
Effective Techniques for Regression Testing
Regression testing is a crucial part of the software development process, ensuring that new code changes do not adversely affect the existing functionality of the application. In this blog post, we will explore some effective techniques for conducting regression testing in Java.
Getting Started to Regression Testing in Java
Regression testing in Java involves re-running test cases to ensure that new code changes have not broken the existing functionality of the application. This is particularly important in Agile and DevOps environments, where frequent code changes are made. By identifying and fixing defects early, regression testing helps maintain the overall quality of the software.
1. Automated Testing with JUnit
JUnit is a popular Java testing framework that is widely used for automated regression testing. It provides annotations such as @Test
, @Before
, and @After
to define test methods and setup/teardown procedures. Here's an example of a simple JUnit test case:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 5);
assertEquals(8, result);
}
}
In this example, the testAddition
method verifies the correctness of the add
method in the Calculator
class. By automating such test cases, we can quickly identify regressions caused by new code changes.
2. TestNG for Functional Testing
TestNG is another popular testing framework for Java that provides more advanced features than JUnit, especially for functional testing and regression testing. TestNG allows for the grouping of test cases, parameterization, and dependency testing. Here's an example of a TestNG test case with parameterization:
import org.testng.annotations.Test;
import org.testng.Assert;
public class ParameterizedTest {
@Test(dataProvider = "testData")
public void testAddition(int a, int b, int expected) {
Calculator calculator = new Calculator();
int result = calculator.add(a, b);
Assert.assertEquals(expected, result);
}
@DataProvider(name = "testData")
public Object[][] testData() {
return new Object[][] { { 3, 5, 8 }, { 2, 2, 4 }, { -1, 1, 0 } };
}
}
3. Mocking with Mockito
Mockito is a popular mocking framework for Java that is extremely useful for regression testing. Mocking allows us to create simulated objects that mimic the behavior of real objects. This is particularly useful when testing code that depends on external services or components. Here's an example of using Mockito to mock a database service:
import static org.mockito.Mockito.*;
import org.junit.Test;
import java.util.List;
public class DatabaseServiceTest {
@Test
public void testDatabaseQuery() {
DatabaseService mockService = mock(DatabaseService.class);
List<String> data = Arrays.asList("result1", "result2");
when(mockService.query()).thenReturn(data);
// Perform the test using the mock service
// ...
}
}
4. Test Coverage Analysis with JaCoCo
JaCoCo is a popular code coverage library for Java, which can be used for regression testing to ensure that all parts of the code have been tested. By analyzing test coverage, we can identify which parts of the code have not been adequately covered by test cases, helping us focus our regression testing efforts. Integrating JaCoCo with build tools such as Maven or Gradle provides automated test coverage reports.
To Wrap Things Up
In conclusion, regression testing is an essential part of the software development process, and using effective techniques in Java is crucial for maintaining the quality of the application. Automated testing with JUnit and TestNG, mocking with Mockito, and test coverage analysis with JaCoCo are just some of the techniques that can be employed to ensure thorough regression testing. By utilizing these techniques, developers can have confidence in the stability and reliability of their code.
Incorporating these techniques into your Java regression testing strategy will not only help you catch defects early but also streamline the testing process, enabling you to release high-quality software with confidence.
By incorporating these techniques into your Java regression testing strategy, you can catch defects early, streamline the testing process, and release high-quality software with confidence. Happy testing!
Remember, the effectiveness of regression testing relies heavily on the thoroughness of the test cases, so make sure to craft comprehensive and meaningful tests to get the most out of these techniques.
For more insights into Java testing, you can explore blogs by Baeldung, a leading Java blog with a wealth of information on testing and development.
So, go ahead, leverage the power of these techniques, and fortify your regression testing process in Java!