Customizing Transaction Validation in Extending Flows
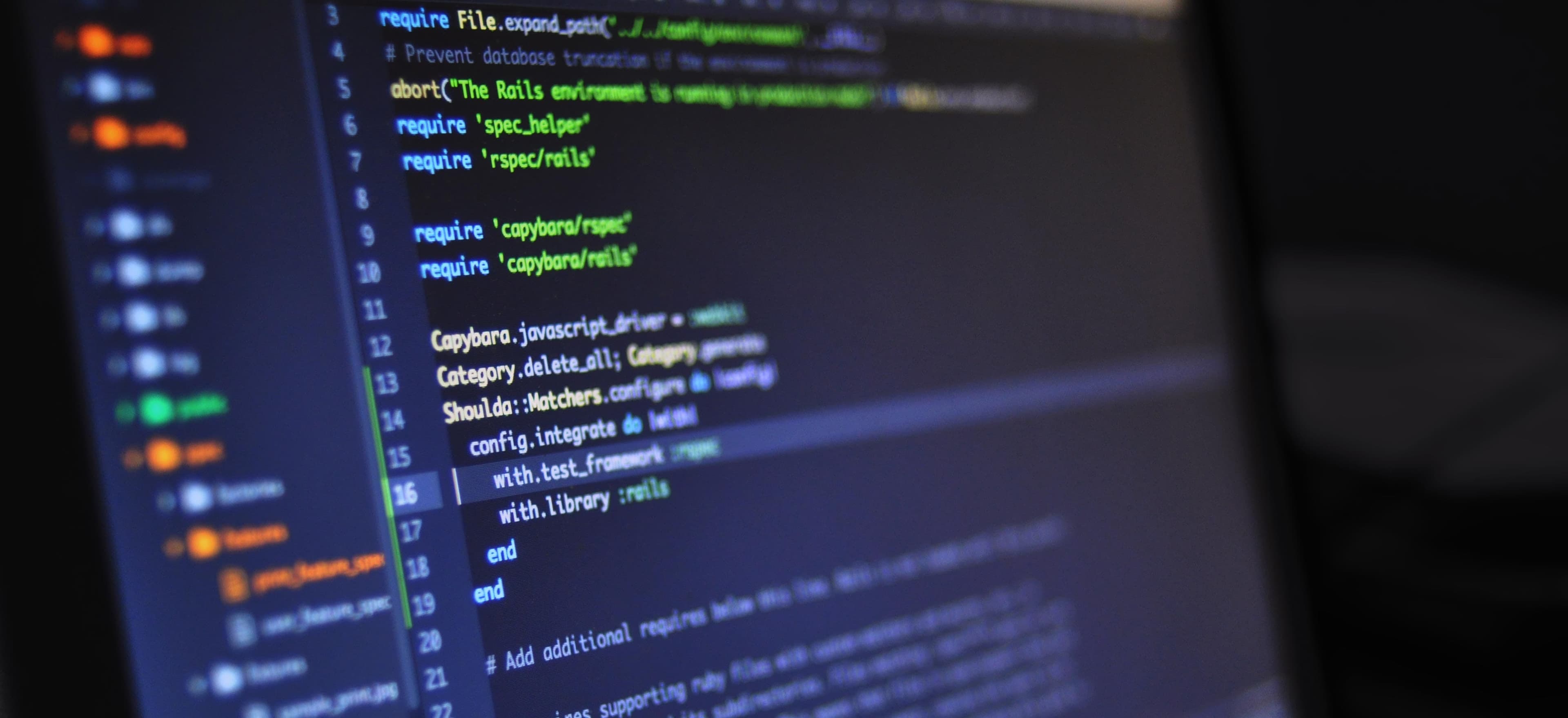
- Published on
Customizing Transaction Validation in Extending Flows
In the world of Java development, handling transaction validation is a crucial aspect of ensuring data integrity and consistency. When working with frameworks like Spring or Java EE, developers often encounter scenarios where they need to customize or extend the default transaction validation behavior.
In this article, we'll explore how to customize transaction validation when extending flows in Java applications. We'll delve into the fundamentals of transaction management, discuss common use cases for custom validation, and provide practical examples of how to achieve this customization.
Understanding Transaction Validation
Before we delve into customizing transaction validation, it's essential to understand the basics of transaction management in Java applications. A transaction is a unit of work that is performed within a database management system. It represents a series of operations that must be executed as a single, indivisible unit. These operations should either all succeed or all fail, ensuring the consistency and integrity of the data.
In Java, frameworks like Spring and Java EE provide support for declarative transaction management, where developers can specify the transactional behavior of their methods using annotations or configuration files. These frameworks also offer ways to customize transaction behavior, including validation logic, to cater to specific application requirements.
Use Cases for Custom Transaction Validation
There are various scenarios where custom transaction validation may be necessary in Java applications. Some common use cases include:
-
Custom Business Rules: When the default validation rules provided by the framework are not sufficient to enforce specific business requirements, custom validation logic may be needed.
-
Integration with External Systems: In cases where a transaction involves interactions with external systems or services, additional validation may be required to ensure data consistency across these boundaries.
-
Complex Data Validation: If the data being processed within a transaction requires complex validation logic that cannot be expressed using standard validation frameworks, custom validation becomes essential.
Customizing Transaction Validation in Java
Let's dive into the practical aspect of customizing transaction validation in Java. To demonstrate this, we'll focus on extending the validation behavior within a Spring-based application.
Example: Custom Transaction Validation in Spring
Consider a scenario where we need to perform custom validation for a financial transaction before committing it to the database. In this case, we can create a custom validator class implementing the TransactionValidator
interface and then incorporate it into the transactional flow.
public interface TransactionValidator {
void validateTransaction(Transaction transaction);
}
public class CustomTransactionValidator implements TransactionValidator {
@Override
public void validateTransaction(Transaction transaction) {
// Custom validation logic
if (transaction.getAmount() <= 0) {
throw new InvalidTransactionException("Transaction amount must be positive");
}
// Additional validation rules
}
}
In the above example, CustomTransactionValidator
implements the TransactionValidator
interface, providing a custom validateTransaction
method to enforce specific validation rules for the transaction object.
Integrating Custom Validation into Transaction Flow
To integrate the custom validation into the transactional flow, we can utilize Spring's AOP (Aspect-Oriented Programming) to apply the validation logic before the transactional method is executed.
@Aspect
@Component
public class TransactionValidationAspect {
private final TransactionValidator transactionValidator;
@Autowired
public TransactionValidationAspect(TransactionValidator transactionValidator) {
this.transactionValidator = transactionValidator;
}
@Before("execution(* com.example.service.TransactionService.processTransaction(..)) && args(transaction)")
public void validateTransactionBeforeExecution(Transaction transaction) {
transactionValidator.validateTransaction(transaction);
}
}
In the above aspect, we define a TransactionValidationAspect
that intercepts the processTransaction
method defined in the TransactionService
. Before the method execution, the validateTransactionBeforeExecution
advice calls the validateTransaction
method from the injected TransactionValidator
, enforcing the custom validation logic.
By leveraging Spring AOP, we effectively integrate custom transaction validation into the transactional flow without cluttering the business logic of the TransactionService
.
Key Takeaways
In this article, we've explored the significance of customizing transaction validation when extending flows in Java applications. Understanding the fundamentals of transaction management and recognizing the need for custom validation are crucial for implementing robust and flexible transactional behavior.
By demonstrating a practical example of custom transaction validation in a Spring-based application, we've illustrated how to seamlessly integrate custom validation logic into the transactional flow using Spring AOP. This approach allows for the enforcement of application-specific validation rules without compromising the modularity and clarity of the business logic.
Customizing transaction validation in Java empowers developers to adapt transaction management to the unique requirements of their applications, ensuring data integrity and consistency in various complex scenarios.
Incorporating custom validation logic within transaction flows not only enhances the reliability of the application but also fosters maintainability and extensibility, laying the foundation for robust and scalable Java applications.
For further reading on transaction management and customization in Java, check out the official Spring documentation and Java EE tutorial for comprehensive insights into transaction-related features and best practices.
Customizing transaction validation is a powerful tool in the arsenal of a Java developer, offering tailored solutions to ensure the integrity and consistency of data within the application's transactional workflows.