Improving MongoDB Aggregation Performance
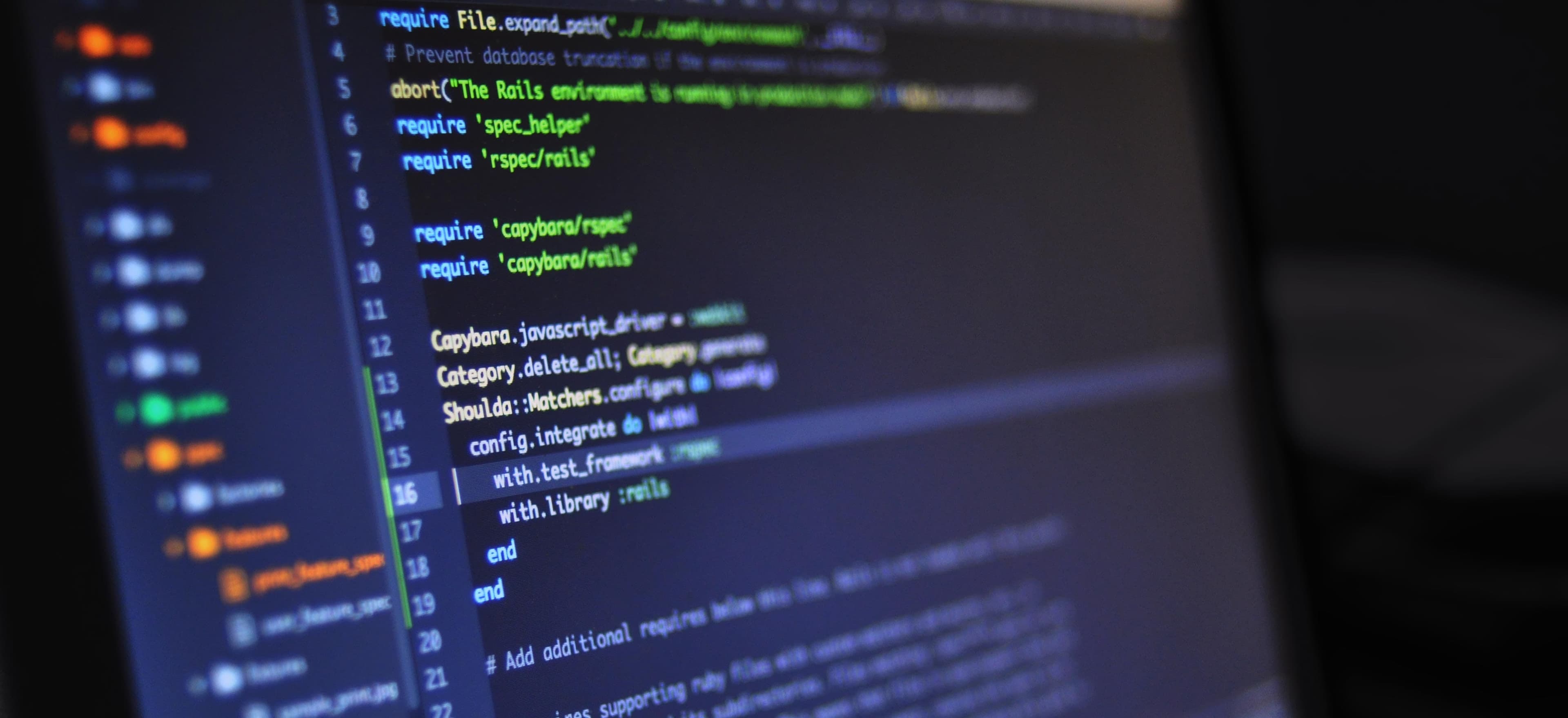
- Published on
Improving MongoDB Aggregation Performance
When working with large datasets in MongoDB, it's vital to ensure that the performance of aggregation queries is optimized. MongoDB provides a powerful aggregation framework that allows for the processing of data and returning computed results. In this article, we'll delve into various strategies to enhance the performance of aggregation queries in MongoDB, optimizing the processing of data for faster query results.
Indexing for Aggregation Queries
One of the most impactful ways to improve aggregation performance in MongoDB is through proper indexing. Indexes help the database engine locate and retrieve data effectively. When crafting aggregation queries, it's crucial to understand the fields being used for filtering, sorting, grouping, and projecting, and create indexes that align with these operations.
Example of creating an index in MongoDB
db.collection.createIndex({ field1: 1, field2: 1 });
In the above example, an index is created on field1
and field2
in ascending order. Depending on the nature of the aggregation query, indexes can be tailored to support specific query patterns, thereby significantly enhancing aggregation performance.
Efficient Pipeline Design
MongoDB's aggregation framework operates on stages represented by different pipeline operators. Crafting an efficient pipeline design is pivotal for optimal aggregation performance. It's essential to utilize the aggregation stages judiciously, avoiding unnecessary computations and data manipulations within the pipeline.
Example of an efficient pipeline design
db.collection.aggregate([
{ $match: { status: "active" } },
{ $group: { _id: "$category", total: { $sum: "$amount" } } },
{ $sort: { total: -1 } },
]);
In the above example, the pipeline starts with a $match
stage to filter documents, followed by a $group
stage to calculate the total amount for each category, and finally concludes with a $sort
stage to sort the results by the total amount. By arranging the stages efficiently, unnecessary data processing is avoided, leading to improved aggregation performance.
Utilizing Projection to Reduce Data Size
Projection in MongoDB aggregation queries allows for the selective inclusion or exclusion of fields, consequently reducing the amount of data that needs to be processed. Efficient usage of projection can significantly enhance aggregation performance by curtailing the amount of data transmitted between server and client and decreasing computational overhead.
Example illustrating the utilization of projection
db.collection.aggregate([
{ $match: { status: "active" } },
{ $project: { _id: 0, category: 1, amount: 1 } },
{ $group: { _id: "$category", total: { $sum: "$amount" } } },
]);
In the above example, the $project
stage is employed to exclude the _id
field and include only the category
and amount
fields, reducing the data size for subsequent processing and resulting in improved aggregation performance.
Using Aggregation Pipeline Optimizations
MongoDB provides various aggregation pipeline optimizations that can be leveraged to enhance performance. Understanding and employing these optimizations, such as the use of the $merge
stage for merging aggregation results into an existing collection or the $out
stage for writing aggregation output to a new collection, can provide significant performance benefits in different scenarios.
Example of using the $merge stage for aggregation optimization
db.collection.aggregate([
{ $group: { _id: "$category", total: { $sum: "$amount" } } },
{ $merge: "aggregatedData" },
]);
In the above example, the $merge
stage is utilized to merge the aggregation results into an existing collection named aggregatedData
. This optimization can be beneficial in scenarios where the aggregated data needs to be stored persistently for further analysis, enabling efficient aggregation performance with reduced overhead.
Utilizing Index Intersection
Index intersection is a powerful mechanism in MongoDB that allows the database engine to combine multiple indexes to fulfill a query. By understanding the query patterns of aggregation operations, it's possible to create indexes that can be effectively combined through index intersection, thereby improving the performance of aggregation queries.
Example of utilizing index intersection in MongoDB
db.collection.createIndex({ status: 1, category: 1 });
In the above example, an index is created on status
and category
fields. This index can be leveraged through index intersection to efficiently support aggregation queries that filter or group based on the status
and category
fields, contributing to improved aggregation performance.
The Bottom Line
Enhancing the performance of aggregation queries in MongoDB is vital for efficiently processing large volumes of data. By employing strategies such as indexing for query patterns, optimizing aggregation pipelines, utilizing projection to reduce data size, leveraging aggregation pipeline optimizations, and understanding index intersection, it's possible to significantly improve aggregation performance. As always, thorough testing and profiling should be performed to validate the effectiveness of the applied optimizations in real-world scenarios, ensuring that aggregation queries in MongoDB operate at peak efficiency.
Incorporating these strategies and best practices will undoubtedly lead to optimized aggregation performance, allowing MongoDB to excel in handling complex data processing tasks with ease.
Remember, optimizing aggregation performance is an ongoing process, and it's essential to continuously analyze and refine the approaches based on evolving data patterns and usage scenarios. By staying informed about the latest advancements and continually fine-tuning aggregation operations, MongoDB can consistently deliver exceptional performance for aggregating and processing data at scale.
For further insights on MongoDB aggregation and performance tuning, refer to the MongoDB official documentation - MongoDB Aggregation.
Now it's your turn to optimize those aggregation queries and unlock superior performance in MongoDB!