"Eliminating Redundant CSS Classes for Cleaner Code"
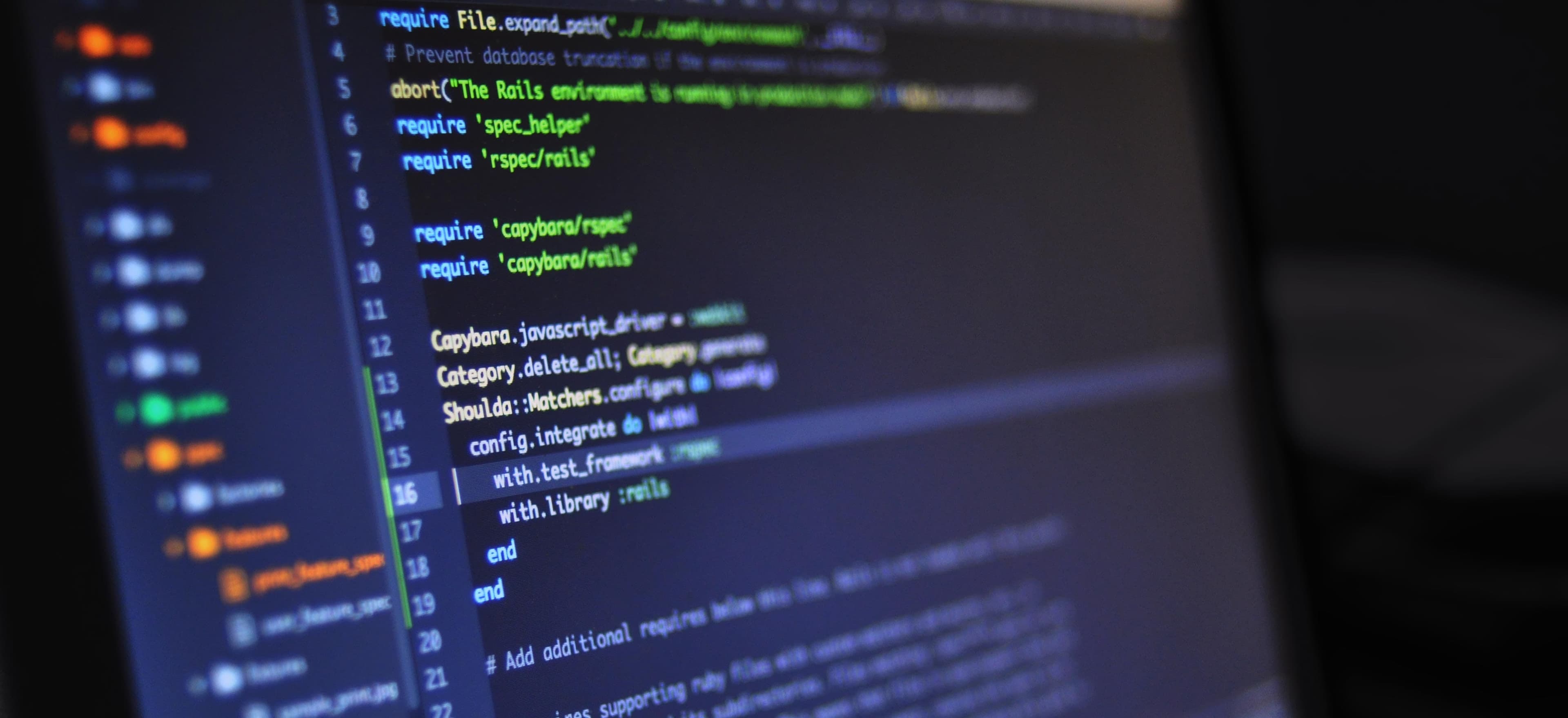
- Published on
Eliminating Redundant CSS Classes for Cleaner Code
In the world of web development, keeping your codebase clean and efficient is crucial for both performance and maintenance. One common area where we can improve our codebase is by eliminating redundant CSS classes. Redundant classes not only bloat our stylesheets but also make our code harder to maintain and understand. In this article, we will explore how we can identify and eliminate redundant CSS classes in our Java web applications, thereby improving the overall quality of our codebase.
Identifying Redundant CSS Classes
Before we can eliminate redundant CSS classes, we need to identify them first. One of the common reasons for redundancy is when multiple CSS classes have the same set of styles. This often occurs as a result of iterative development and a lack of code refactoring.
Let's consider the following example:
<div class="button primary">Submit</div>
.button {
/* Styles common to all buttons */
}
.primary {
/* Styles specific to primary buttons */
}
In this example, the .primary
class doesn't add any specific styles that aren't already covered by the .button
class. This redundancy can easily creep into a codebase, especially in larger projects with multiple developers.
The "Why" Behind Eliminating Redundant CSS Classes
- Performance: Redundant CSS classes contribute to larger stylesheet sizes, which can impact page loading times.
- Maintainability: When styles are scattered across redundant classes, it becomes harder to maintain and update them consistently.
- Clarity: Redundant classes can lead to confusion and make it difficult for developers to understand the purpose of each class.
How to Eliminate Redundant CSS Classes
1. Consolidate Styles
To eliminate redundant CSS classes, we can start by consolidating styles. Instead of having separate classes for common styles and variations, we can merge them into a single class.
Before:
<div class="button primary">Submit</div>
.button {
/* Styles common to all buttons */
}
.primary {
/* Styles specific to primary buttons */
}
After:
<div class="button">Submit</div>
.button {
/* Styles common to all buttons */
/* Styles specific to primary buttons */
}
By consolidating the styles, we remove the need for the .primary
class altogether, thus reducing redundancy in our codebase.
2. Use More Specific Selectors
Another approach to eliminate redundant CSS classes is to use more specific selectors. Instead of creating separate classes for specific cases, we can utilize CSS combinators and pseudo-classes to target the elements more precisely.
For example, rather than having separate classes for primary buttons, secondary buttons, and so on, we can use the :hover
pseudo-class and attribute selectors to achieve the same effect without duplicating styles.
3. Leverage CSS Preprocessors
CSS preprocessors like Sass and Less provide features such as variables, mixins, and nesting, which can help in reducing redundancy by promoting code reusability and maintainability.
By using variables for common styles and mixins for variations, we can significantly cut down on redundant classes in our stylesheets.
Lessons Learned
Eliminating redundant CSS classes is not only about reducing the size of our stylesheets but also about writing maintainable and understandable code. By identifying and consolidating redundant classes, we can improve the performance and maintainability of our Java web applications, leading to a better overall user experience and developer satisfaction.
In conclusion, keeping a clean codebase should be a priority for every developer. By striving for efficiency and simplicity, we can ensure that our Java web applications are not only performant but also easy to maintain and extend.
Remember, clean code is the best code!
Check out this article for more insights on maintaining legacy systems while implementing new technologies. Also, take a look at this guide on using Sass mixins for smarter CSS.