Maximizing EJB Passivation Efficiency
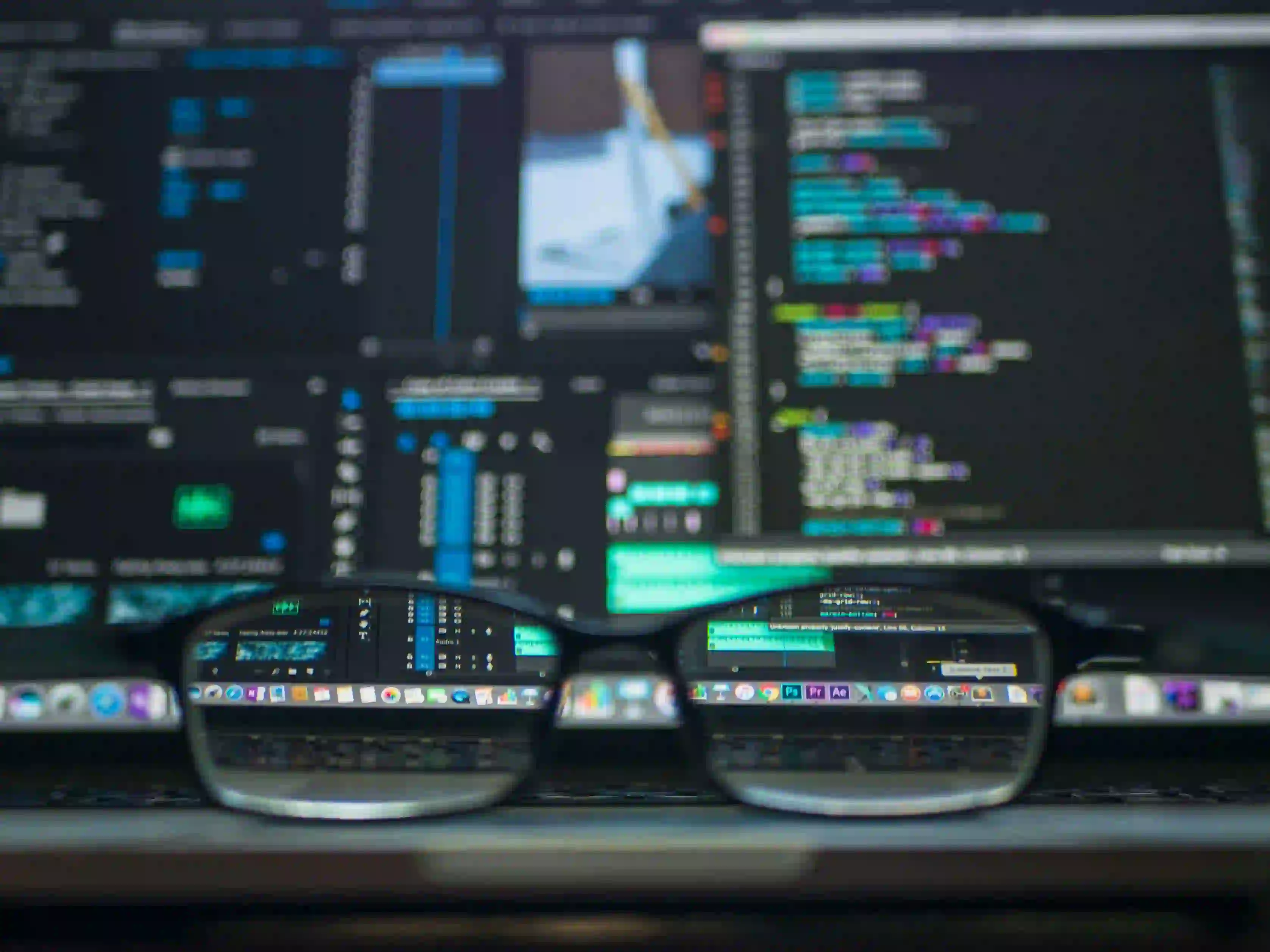
Maximizing EJB Passivation Efficiency
Enterprise JavaBeans (EJB) have been a fundamental part of Java EE (now Jakarta EE) for a long time. EJBs provide a powerful and scalable model for building enterprise-level applications. One key feature of EJBs is passivation, which allows the container to store a bean's state to disk when the bean is not being used actively, thus saving memory.
In this article, we will explore how to maximize EJB passivation efficiency to ensure optimal performance and scalability in your Java EE applications.
Understanding EJB Passivation
Before optimizing passivation efficiency, it's essential to understand what passivation is and how it works in EJBs.
In EJB, passivation is the process of moving a stateful session bean (SFSB) from memory to secondary storage when the container needs to reclaim memory. This is typically done when the bean is idle and not being used. The passivated bean can then be activated and brought back into memory when it is needed again.
Passivation is especially crucial for stateful session beans as they consume more memory than stateless beans, and efficient passivation helps in conserving memory and optimizing the performance of the application.
Tips for Maximizing EJB Passivation Efficiency
To maximize EJB passivation efficiency, consider the following tips and best practices:
1. Minimize State Size
When designing EJBs, it's important to minimize the size of the bean's state. This can be achieved by avoiding unnecessary attributes and only storing essential data within the bean. By reducing the amount of data that needs to be passivated and activated, the passivation process becomes more efficient.
2. Mark Non-serializable Fields as Transient
In Java, fields that are not serializable will not be included in the passivation process. By marking non-serializable fields as transient, you can exclude them from passivation, thereby reducing the amount of data that needs to be written to disk during passivation.
public class MyStatefulBean implements Serializable {
private transient Logger logger; // Marking non-serializable field as transient
// Other bean fields and methods
}
3. Optimize Disk I/O
Efficient disk I/O is crucial for improving passivation performance. Using high-performance storage for passivating beans, such as SSDs, can significantly reduce the time it takes to write and read the bean's state to and from disk.
4. Use Lazy Loading
Consider using lazy loading for bean attributes that are not always needed. By loading data only when it is actually required, you can reduce the amount of state that needs to be passivated, leading to better passivation efficiency.
5. Implement Custom Passivation Callbacks
EJBs provide the @PrePassivate
and @PostActivate
lifecycle callbacks, which allow you to perform custom logic before passivation and after activation. Utilizing these callbacks can help in managing resources and optimizing the passivation process based on the specific needs of the bean.
@Stateful
public class MyStatefulBean implements MyStatefulBeanRemote {
// EJB methods
@PrePassivate
private void prePassivate() {
// Custom logic before passivation
}
@PostActivate
private void postActivate() {
// Custom logic after activation
}
}
6. Monitor Passivation Events
Monitoring passivation events can provide insights into the frequency and duration of passivation, helping you identify areas for improvement. Jakarta EE application servers often provide monitoring and management APIs that can be used to track passivation-related metrics.
7. Consider using EJB 3.2 Features
If you are using EJB 3.2 or later versions, take advantage of the enhanced passivation and activation features introduced in these specifications. EJB 3.2 introduced the @Cacheable
annotation, which allows fine-grained control over when passivation should occur.
By following these tips and best practices, you can maximize the passivation efficiency of your EJBs, leading to improved performance and scalability of your Jakarta EE applications.
Final Considerations
Optimizing EJB passivation efficiency is essential for ensuring the performance and scalability of enterprise Java applications. By minimizing state size, optimizing disk I/O, using lazy loading, implementing custom passivation callbacks, monitoring passivation events, and leveraging EJB 3.2 features, you can achieve optimal passivation efficiency in your applications.
For further reading on EJB passivation and optimization, the Jakarta EE documentation and Java EE Design Patterns can provide comprehensive insights into best practices and advanced techniques.
Remember, efficient EJB passivation is a critical aspect of building high-performance Jakarta EE applications, and investing time in understanding and optimizing passivation can significantly impact the overall quality of your enterprise software.