Optimizing Java Lock Performance
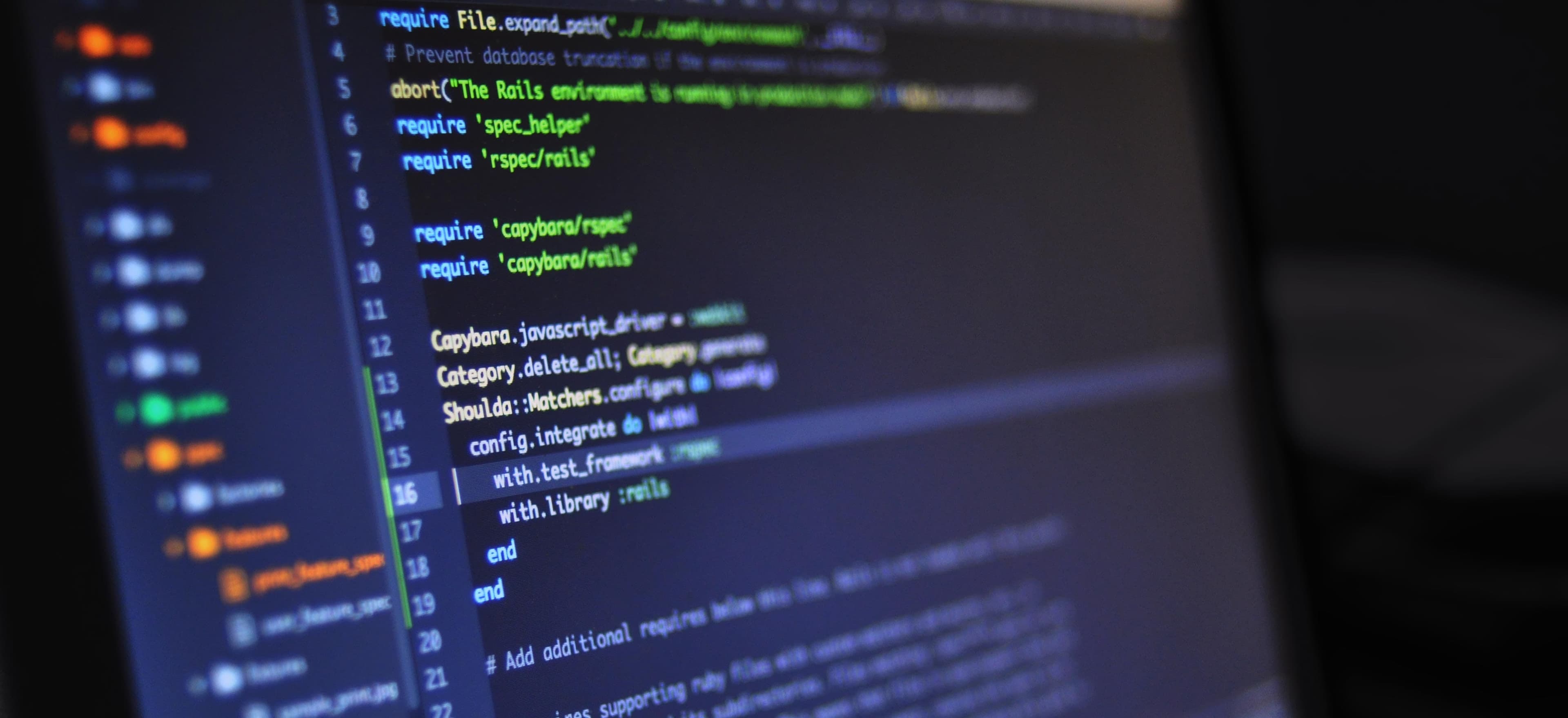
- Published on
Understanding and Optimizing Java Lock Performance
When developing concurrent Java applications, ensuring optimal lock performance is crucial for achieving efficient and scalable multi-threaded programs. In this article, we will delve into the intricacies of Java locks, their performance implications, and strategies for optimizing their usage.
The Importance of Lock Performance
In a multi-threaded environment, locks are essential for synchronizing access to shared resources, preventing data races, and maintaining consistency. However, the misuse or suboptimal implementation of locks can lead to various performance issues such as contention, deadlock, and increased thread overhead.
Ensuring high-performance locks is crucial for maintaining application responsiveness, scalability, and overall throughput. Therefore, understanding the underlying mechanisms and intricacies of Java locks is vital for writing efficient concurrent code.
Built-in Locking Mechanisms in Java
Java provides several built-in locking mechanisms, with the most commonly used ones being synchronized
blocks and the ReentrantLock
class. Both approaches serve the purpose of providing mutual exclusion, but they differ in their flexibility and performance characteristics.
Synchronized Blocks
A synchronized
block is the most straightforward way to provide thread-safe access to a block of code in Java. While it offers simplicity and readability, it may not provide as much fine-grained control and performance optimizations compared to ReentrantLock
.
public class SynchronizedExample {
private final Object lock = new Object();
public void performSynchronizedTask() {
synchronized (lock) {
// Synchronized code block
}
}
}
ReentrantLock
The ReentrantLock
class, introduced in Java 5, offers a more flexible and powerful alternative to intrinsic locks. It provides advanced features such as the ability to interruptibly lock, try-lock with timeout, and condition variables. Additionally, it allows greater control over fairness and can potentially yield better performance in certain scenarios.
import java.util.concurrent.locks.ReentrantLock;
public class ReentrantLockExample {
private final ReentrantLock lock = new ReentrantLock();
public void performLockedTask() {
lock.lock();
try {
// Locked code block
} finally {
lock.unlock();
}
}
}
Performance Considerations
Contention and Scalability
One of the primary performance considerations when dealing with locks is contention. Contention occurs when multiple threads attempt to acquire the same lock simultaneously, leading to serialization and potential performance bottlenecks.
To mitigate contention and improve scalability, it's vital to design lock usage patterns that minimize the time critical sections are locked and to utilize lock-free algorithms where possible.
Lock Granularity
Another crucial aspect impacting lock performance is granularity. Lock granularity refers to the size of the protected code segment and determines the scope of the lock. Coarse-grained locks covering large sections of code can lead to increased contention and reduced concurrency. On the other hand, fine-grained locks can minimize contention and improve overall throughput.
Choosing the appropriate level of granularity is essential for achieving optimal lock performance, and it often involves careful analysis of the data access patterns and potential hotspots within the application.
Locking Strategies
Effective locking strategies play a pivotal role in achieving optimal performance. Choosing the right locking mechanisms, applying reentrant or read-write locks where applicable, and utilizing lock stripping techniques are among the strategies that can significantly impact lock performance.
Optimizing Lock Usage
Reduce Lock Contention
Reducing lock contention is essential for improving lock performance. Strategies such as lock splitting, lock striping, and using non-blocking algorithms can help alleviate contention by allowing multiple threads to access different parts of the data concurrently.
For example, using ConcurrentHashMap
instead of a synchronized HashMap
can significantly reduce contention by partitioning the data and employing fine-grained locking internally.
// Synchronized HashMap
Map<String, String> synchronizedMap = Collections.synchronizedMap(new HashMap<>());
// ConcurrentHashMap
ConcurrentMap<String, String> concurrentMap = new ConcurrentHashMap<>();
Favoring Read-Write Locks
In scenarios where the data access patterns are predominantly read-intensive, leveraging read-write locks can improve concurrency by allowing multiple threads to read simultaneously while still ensuring exclusive access during write operations.
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReadWriteLockExample {
private final ReentrantReadWriteLock rwLock = new ReentrantReadWriteLock();
private final ReentrantReadWriteLock.ReadLock readLock = rwLock.readLock();
private final ReentrantReadWriteLock.WriteLock writeLock = rwLock.writeLock();
public void performReadTask() {
readLock.lock();
try {
// Read operation
} finally {
readLock.unlock();
}
}
public void performWriteTask() {
writeLock.lock();
try {
// Write operation
} finally {
writeLock.unlock();
}
}
}
Use Lock Striping
Lock striping is a technique that partitions the protected data into multiple segments, each with its lock. This approach can reduce contention by allowing independent locks to operate on different parts of the data structure concurrently.
For instance, the ConcurrentHashMap
internally uses lock striping to achieve better concurrent performance by dividing the map into separate segments, each guarded by a distinct lock.
ConcurrentHashMap<String, String> concurrentHashMap = new ConcurrentHashMap<>();
Benchmark and Profile
Benchmarking and profiling lock usage is paramount for identifying potential contention points and evaluating the effectiveness of locking strategies. Tools such as JMH (Java Microbenchmark Harness) and profilers like VisualVM and YourKit can provide valuable insights into lock performance and help pinpoint areas for optimization.
// Example benchmarking with JMH
@State(Scope.Benchmark)
public class LockBenchmark {
private final ReentrantLock lock = new ReentrantLock();
@Benchmark
@Threads(8)
public void benchmarkLockPerformance() {
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
}
}
The Last Word
Optimizing lock performance in Java is essential for building responsive and scalable concurrent applications. By understanding the nuances of lock contention, granularity, and effective locking strategies, developers can make informed decisions to improve the performance of their multi-threaded programs.
Adopting appropriate locking mechanisms, reducing contention, leveraging read-write locks, and employing lock striping are essential tactics for achieving efficient lock performance. Continuous benchmarking and profiling are crucial for identifying bottlenecks and fine-tuning the locking strategies for optimal concurrency.
By applying these optimization techniques and adhering to best practices, developers can harness the full potential of Java locks and create high-performance concurrent applications.
For further reading, you may refer to the following resources:
- Java Locks and Synchronization
- Effective Java Concurrency: Locking
- Understanding Java Locks
Remember, acquiring seamless lock performance is not just about the code; it's about thoughtful, strategic application, impacting the efficiency and endurance of your concurrent Java applications.