Overcoming Bottlenecks in Continuous Integration and Delivery
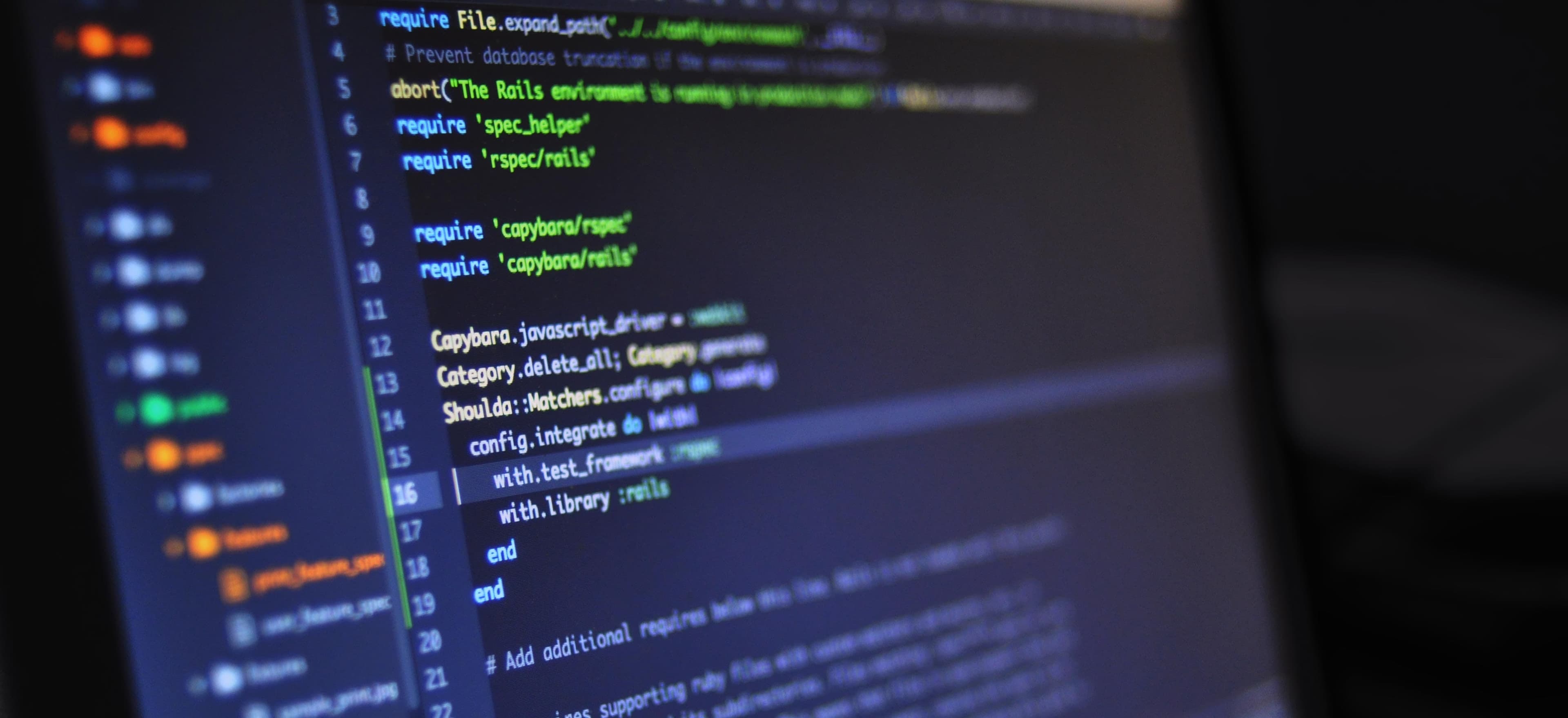
- Published on
Overcoming Bottlenecks in Continuous Integration and Delivery
In the fast-paced world of software development, Continuous Integration and Continuous Delivery (CI/CD) have become essential practices to ensure rapid and reliable delivery of high-quality software. However, as software projects grow in complexity and scale, bottlenecks can arise, impeding the smooth flow of the CI/CD pipeline. In this blog post, we will explore common bottlenecks in CI/CD, and discuss strategies and best practices to overcome these hurdles using Java.
Identifying Bottlenecks in CI/CD
1. Slow Builds and Tests
One of the most prevalent bottlenecks in CI/CD is slow build and test processes. As the codebase expands, build and test times tend to increase, slowing down the feedback loop. This can result in longer development cycles and reduced productivity.
2. Manual Intervention
Manual interventions such as code reviews, approvals, and deployments can introduce delays and bottlenecks in the CI/CD pipeline. While these steps are crucial for maintaining code quality, excessive manual intervention can hinder the rapid delivery of software.
3. Resource Constraints
Limited infrastructure resources such as CPU, memory, and storage can also pose significant bottlenecks in CI/CD. Insufficient resources can lead to queue buildup, longer build times, and unstable CI/CD pipelines.
Strategies to Overcome Bottlenecks
1. Code Optimizations
Optimizing the codebase can significantly reduce build times and test durations. Techniques such as code refactoring, parallel test execution, and efficient dependency management can streamline the CI/CD process.
Example:
public class Example {
public int add(int a, int b) {
return a + b;
}
}
In this example, we have a simple add
method. However, in a real-world scenario, this method might be part of a larger codebase. By optimizing the codebase and improving algorithm efficiency, we can reduce execution times.
2. Automated Testing
Implementing a robust suite of automated tests, including unit tests, integration tests, and end-to-end tests, can help catch bugs early and ensure the stability of the codebase. This reduces the reliance on manual testing and accelerates the feedback loop.
3. Continuous Deployment
Automating the deployment process minimizes manual intervention and reduces deployment times. Tools like Jenkins, Travis CI, and GitLab CI enable seamless integration with version control systems and facilitate automated deployments.
4. Scalable Infrastructure
Utilizing cloud-based services and scalable infrastructure allows for dynamic allocation of resources based on demand. Platforms such as AWS, Google Cloud, and Azure offer auto-scaling capabilities, ensuring that resources are available when needed.
Leveraging Java for CI/CD Optimization
Java, with its robust ecosystem and extensive tooling, provides several avenues to address CI/CD bottlenecks.
1. Gradle Build Optimization
Gradle, a popular build automation tool for Java, offers features such as incremental builds and build caching to minimize build times. By leveraging these features, developers can accelerate the build process, especially in large projects.
2. TestNG for Parallel Test Execution
TestNG, a testing framework for Java, supports parallel test execution out of the box. By running tests in parallel, developers can significantly reduce the overall test execution time, leading to faster feedback on the code quality.
Example:
@Test
public void testAddition() {
// Test logic here
}
By annotating test methods with @Test
, TestNG can identify and run these tests in parallel, improving testing efficiency.
3. Docker for Containerized Deployments
Docker, along with tools like Kubernetes, provides a robust platform for containerized deployments. Packaging Java applications in Docker containers allows for consistent and reproducible deployments, mitigating potential deployment bottlenecks.
Dockerfile Example:
FROM openjdk:11
COPY ./target/app.jar /app
CMD ["java", "-jar", "/app/app.jar"]
Using Docker, we can create a Dockerfile to package and deploy our Java application in a containerized environment.
Final Thoughts
Continuous Integration and Delivery are integral parts of modern software development practices, and addressing bottlenecks in the CI/CD pipeline is crucial for maintaining efficiency and velocity. By employing strategies such as code optimizations, automated testing, continuous deployment, and scalable infrastructure, teams can mitigate bottlenecks and ensure a smooth CI/CD process. With Java's extensive tooling and ecosystem, developers can leverage various optimizations and best practices to streamline their CI/CD pipelines, ultimately delivering high-quality software at a rapid pace.
In summary, identifying bottlenecks, implementing optimization strategies, and leveraging Java's capabilities are key steps towards overcoming CI/CD hurdles, paving the way for a seamless and efficient software delivery process.