Dynamic Class Reloading in Java: A Complete Guide
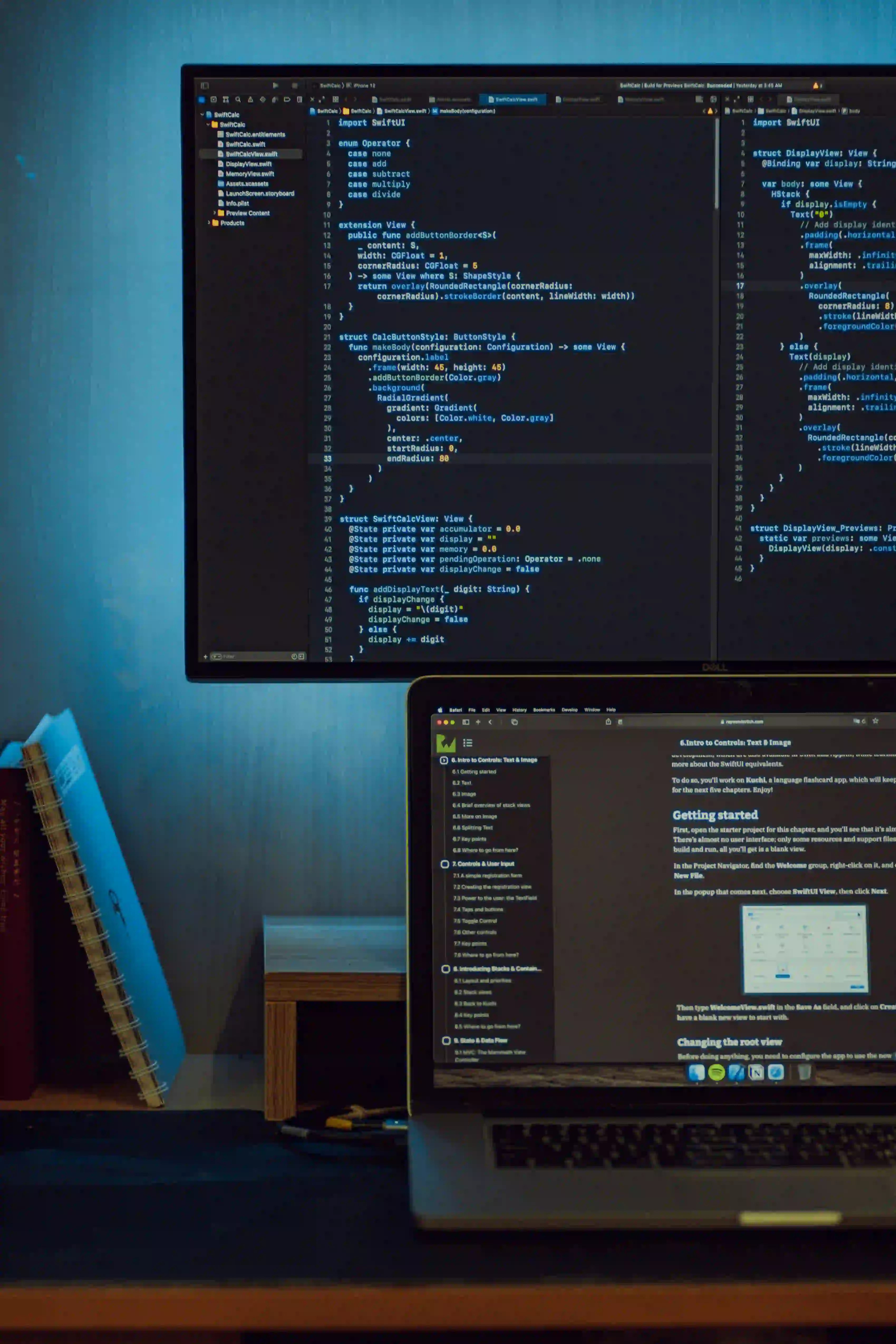
Dynamic Class Reloading in Java: A Complete Guide
Dynamic class reloading in Java allows you to reload and update classes at runtime without restarting the application. This can be particularly useful during development and testing phases, where frequent changes are made to the codebase. In this blog post, we will explore the concept of dynamic class reloading, its benefits, and how to implement it in Java.
Understanding Dynamic Class Reloading
When a Java application is running, the classes are loaded by the class loader and stored in the JVM's memory. Dynamic class reloading involves replacing the classes in memory with new versions without restarting the application. This enables developers to make changes to the code and see the effects immediately without going through the build and deployment process repeatedly.
Benefits of Dynamic Class Reloading
Dynamic class reloading offers several benefits, including:
- Faster Development: Developers can make changes to the code and see the results without restarting the application, which can significantly speed up the development process.
- Improved Testing: Testers can quickly iterate on test cases without incurring the overhead of restarting the application for each change.
- Enhanced Debugging: Developers can make on-the-fly changes to the code and test different scenarios without restarting the application, making debugging more efficient.
Implementing Dynamic Class Reloading
Using Java ClassLoaders
In Java, dynamic class reloading is typically implemented using custom class loaders. Here’s an example of how to implement dynamic class reloading using a custom class loader:
public class CustomClassLoader extends ClassLoader {
public Class<?> loadClass(String className) throws ClassNotFoundException {
// logic to load the class
}
public void reloadClass(String className) {
// logic to reload the class
}
}
In this example, the CustomClassLoader
extends the ClassLoader
class and provides methods to load and reload classes dynamically. The loadClass
method loads the initial version of the class, while the reloadClass
method replaces the existing class with a new version.
Using Libraries
There are also libraries available that facilitate dynamic class reloading in Java, such as JRebel and DCEVM. These tools provide more advanced features and seamless integration with popular IDEs, making it easier to incorporate dynamic class reloading into the development workflow.
Best Practices for Dynamic Class Reloading
While dynamic class reloading can be a powerful tool, it’s important to consider best practices to avoid potential pitfalls:
- Avoid Memory Leaks: Ensure that the old versions of the classes are properly garbage collected to prevent memory leaks.
- Maintain State Consistency: Take into account the state of the application when reloading classes to avoid inconsistencies and unexpected behavior.
- Perform Testing: Although dynamic class reloading can streamline the development process, thorough testing is crucial to ensure that the application remains stable and reliable.
The Closing Argument
Dynamic class reloading in Java provides a convenient way to update classes at runtime, offering benefits such as faster development, improved testing, and enhanced debugging. By leveraging custom class loaders or libraries, developers can implement dynamic class reloading efficiently while adhering to best practices to maintain application stability.
To delve deeper into dynamic class reloading and its implications, you may want to explore the OpenJDK documentation and the Java Virtual Machine specification. Stay tuned for more insightful articles on Java development!
Remember, mastering dynamic class reloading can significantly impact your development workflow, leading to more efficient and productive coding sessions. Embrace the power of dynamic class reloading and elevate your Java development experience!
Happy coding!