Improving Accuracy of Android Activity Recognition
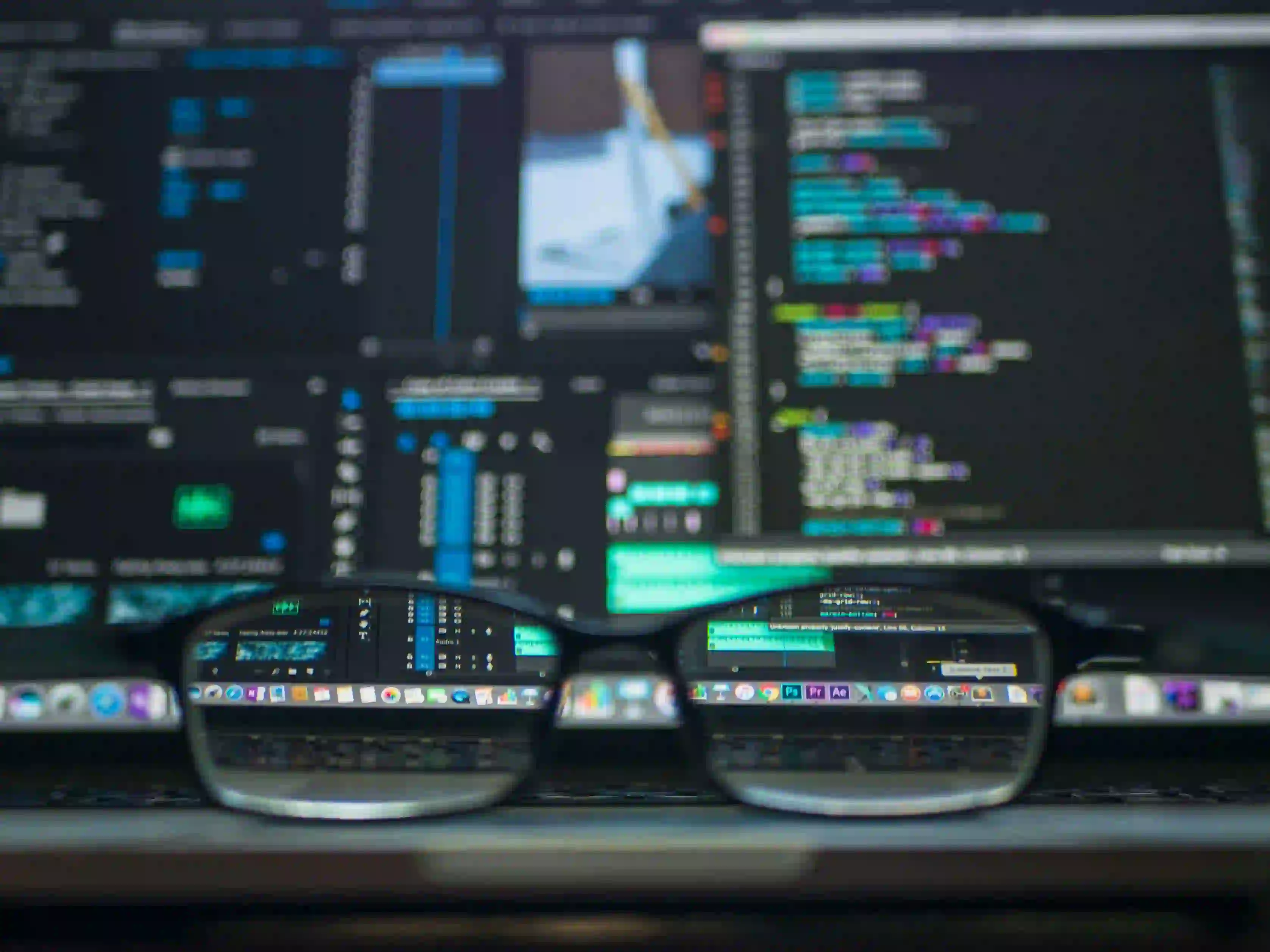
Improving Accuracy of Android Activity Recognition
Activity recognition is an essential feature in many Android applications, allowing them to understand a user's behavior and context to provide more personalized and relevant experiences. However, achieving high accuracy in activity recognition can be challenging due to varying sensor data and user behavior. In this blog post, we will explore some strategies to improve the accuracy of activity recognition in Android using the Google Play Services API.
Understanding Activity Recognition
Activity recognition in Android involves identifying the user's current activity, such as walking, running, cycling, or being still. Google Play Services provides a robust Activity Recognition API that uses sensor data, including accelerometer and gyroscope readings, to detect and classify the user's activity in real-time.
The Challenge of Accuracy
While the Activity Recognition API provides a convenient way to access activity data, ensuring high accuracy can be difficult. Factors such as sensor noise, device placement, and individual variations in movement patterns can lead to misclassifications and false positives.
Strategies to Improve Accuracy
1. Sensor Fusion
Sensor fusion involves combining data from multiple sensors to improve accuracy and robustness. In the context of activity recognition, fusing data from the accelerometer, gyroscope, and even the magnetometer can provide a more comprehensive view of the user's movements.
Example:
SensorManager sensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE);
Sensor accelerometer = sensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER);
Sensor gyroscope = sensorManager.getDefaultSensor(Sensor.TYPE_GYROSCOPE);
// Register sensor listeners
sensorManager.registerListener(this, accelerometer, SensorManager.SENSOR_DELAY_NORMAL);
sensorManager.registerListener(this, gyroscope, SensorManager.SENSOR_DELAY_NORMAL);
By registering listeners for multiple sensors, we can obtain richer data for activity recognition.
2. Machine Learning Models
Integrating machine learning models, such as decision trees or neural networks, can enhance the accuracy of activity recognition. These models can learn from labeled sensor data and adapt to individual user behavior, improving accuracy over time.
Example:
// Load pre-trained machine learning model
Model activityModel = ModelLoader.loadModel("activity_model.ml");
// Make predictions
String activity = activityModel.predict(currentSensorData);
By leveraging machine learning, we can build more sophisticated activity recognition systems.
3. Contextual Information
Incorporating contextual information, such as time of day, location, and user history, can help refine activity predictions. By considering the user's environment and past behavior, we can make more accurate predictions about their current activity.
Example:
// Use location data to infer activity (e.g., walking near a park)
if (currentLocation.equals("park")) {
return "walking";
}
By including contextual information, we can make activity recognition more context-aware and accurate.
4. Continuous Calibration
Regular calibration of activity recognition models based on user feedback and ground truth data can help maintain accuracy over time. By continuously updating models and parameters based on user input, we can adapt to changes in sensor behavior and user activity patterns.
To Wrap Things Up
Achieving high accuracy in activity recognition is crucial for delivering meaningful user experiences in Android applications. By employing strategies such as sensor fusion, machine learning, contextual information, and continuous calibration, developers can enhance the accuracy of activity recognition and provide users with more personalized and reliable functionality.
Implementing these strategies may require a deep understanding of sensor data processing, machine learning, and context-aware computing, but the benefits in terms of user experience and application quality make it a worthwhile investment.
To learn more about the Google Play Services Activity Recognition API and advanced techniques for improving accuracy, explore the official documentation and research papers on sensor data processing and machine learning in activity recognition.